1
using System;
2
using System.Collections.Generic;
3
using System.Linq;
4
using System.Text;
5
using System.ComponentModel;
6
7
namespace EventSample
8
{
9
//定义事件参数类
10
public class AlarmEventArgs:EventArgs
11
{
12
private readonly bool keepingSleep;
13
private readonly int numrings;
14
15
//构造函数
16
public AlarmEventArgs(bool keepingSleep, int numrings)
17
: base()
18
{
19
this. keepingSleep = keepingSleep;
20
this.numrings = numrings;
21
}
22
23
//定义属性
24
public int NumRings
25
{
26
get { return numrings; }
27
}
28
29
public bool KeepingSleep
30
{
31
get { return keepingSleep; }
32
}
33
34
public string AlarmText
35
{
36
get
37
{
38
if ( keepingSleep)
39
{
40
return ("要迟到了!懒虫快起床!");
41
}
42
else
43
{
44
return ("太阳出来啰,起床吧!");
45
}
46
}
47
}
48
}
49
50
//声明自定义代理类型,它的事件参数的类型为AlarmEventArgs
51
public delegate void AlarmEventHandler(object sender, AlarmEventArgs e);
52
53
//包含事件和触发事件方法的类
54
public class AlarmClock
55
{
56
private bool keepingSleep=false ;
57
private int numrings=0;
58
private bool stop=false ;
59
60
public bool Stop
61
{
62
get {return stop; }
63
set {stop =value ;}
64
}
65
66
public bool KeepingSleep
67
{
68
get {return keepingSleep; }
69
set { keepingSleep=value ;}
70
}
71
72
//声明事件,它所使用的代理类型为AlarmEventHandler
73
public event AlarmEventHandler Alarm;
74
75
76
//触发事件的方法
77
protected virtual void OnAlarm(AlarmEventArgs e)
78
{
79
if (Alarm !=null )
80
{
81
//通过代理调用事件处理方法
82
Alarm (this ,e );
83
}
84
}
85
86
//在该方法中循环调用OnAlarm方法来触发事件,直到stop字段的值为true
87
public void Start()
88
{
89
for ( ; ; )
90
{
91
numrings++;
92
if (stop)
93
{
94
break;
95
}
96
else if (KeepingSleep)
97
{
98
//程序暂停0.5秒钟
99
System.Threading.Thread.Sleep(500);
100
{
101
//创建事件参数对象实例
102
AlarmEventArgs e = new AlarmEventArgs(KeepingSleep, numrings);
103
OnAlarm(e);
104
//触发事件,在事件处理方法中会相应地设置KeepingSleep和Stop字段的值(通过属性来设置)
105
}
106
}
107
else
108
{
109
//程序暂停0.3秒
110
System.Threading.Thread.Sleep(300);
111
AlarmEventArgs e = new AlarmEventArgs(keepingSleep, numrings);
112
OnAlarm(e);//触发事件
113
}
114
}
115
}
116
}
117
118
//包含事件处理方法的类
119
public class WakeMeUp
120
{
121
//事件处理方法,在该方法中根据用户的输入来通过事件参数sender设置事件发送者属性
122
public void AlarmRing(object sender, AlarmEventArgs e)
123
{
124
Console.WriteLine(e.AlarmText + "\n");
125
126
if (!(e.KeepingSleep))
127
{
128
if (e.NumRings % 10 == 0)
129
{
130
Console.WriteLine("继续叫你起床!(请输入Y)");
131
Console.WriteLine("让你再睡一会儿!(请输入N)");
132
Console.WriteLine("停止闹铃!(请输入不同于Y和N的键)");
133
string input = Console.ReadLine();
134
135
if (input.Equals("Y") || input.Equals("y")) return;
136
else if (input.Equals("N") || input.Equals("n"))
137
{
138
//通过事件参数sender来访问触发事件的对象
139
((AlarmClock)sender).KeepingSleep = true;
140
return;
141
}
142
else
143
{
144
((AlarmClock)sender).Stop = true;
145
return;
146
}
147
}
148
}
149
else
150
{
151
Console.WriteLine("继续叫你起床!(请输入Y)");
152
string input = Console.ReadLine();
153
if (input.Equals("Y") || input.Equals("y")) return;
154
else
155
{
156
((AlarmClock)sender).Stop = true;
157
return;
158
}
159
}
160
}
161
}
162
163
//包含程序入口方法的类
164
public class AlarmDriver
165
{
166
public static void Main(string[] args)
167
{
168
//创建事件接收者对象实例
169
WakeMeUp waking = new WakeMeUp();
170
171
//创建事件发送者对象实例
172
AlarmClock clock = new AlarmClock();
173
174
//把事件处理方法绑定到发送者的事件上
175
clock.Alarm += new AlarmEventHandler(waking.AlarmRing);
176
177
clock.Start();
178
}
179
}
180
}
181
182
运行结果:
2

3

4

5

6

7

8

9

10

11

12

13

14

15

16

17

18

19

20

21

22

23

24

25

26

27

28

29

30

31

32

33

34

35

36

37

38

39

40

41

42

43

44

45

46

47

48

49

50

51

52

53

54

55

56

57

58

59

60

61

62

63

64

65

66

67

68

69

70

71

72

73

74

75

76

77

78

79

80

81

82

83

84

85

86

87

88

89

90

91

92

93

94

95

96

97

98

99

100

101

102

103

104

105

106

107

108

109

110

111

112

113

114

115

116

117

118

119

120

121

122

123

124

125

126

127

128

129

130

131

132

133

134

135

136

137

138

139

140

141

142

143

144

145

146

147

148

149

150

151

152

153

154

155

156

157

158

159

160

161

162

163

164

165

166

167

168

169

170

171

172

173

174

175

176

177

178

179

180

181

182

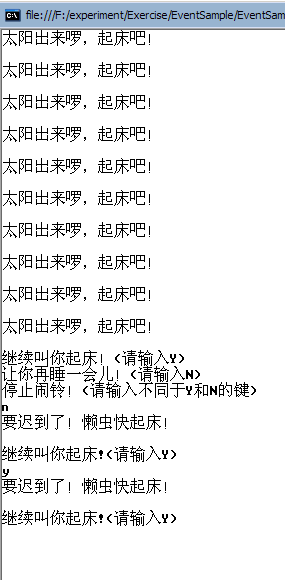