Java 8
Spring Boot 2.5.2
Spring Cloud 2020.0.3
--
本文为 搞清楚classpath而作。
参考文档里面的两篇文章讲的很到位,可以直接看它们。
不同项目可能略有不同。
Java文件首先“编译”成字节码文件——*.class,然后运行字节码文件——平台无关。
怎么运行?通过不同层级的ClassLoader——ClassLoader加载class文件到内存,然后从main函数开始运行。
classpath即指出 不同的class文件的位置。来自 博客园
在javac、java命令中有 -cp、-classpath 选项,可以指定 类加载路径。
javac的:
java的:
三种获取classpath的方式:
// 方式1
ctx.getClassLoader().getResources("");
// 方式2
ClassLoader.getSystemResources("");
底层调用:
return getSystemClassLoader().getResources(name);
// 方式3
Thread.currentThread().getContextClassLoader().getResources("");
除了 getResources函数,还有 getResource 函数,前者返回 Enumeration<URL>,后者仅返回一个 URL。
疑问:
getResources函数的参数 只能是 "" 吗?来自 博客园
目录
程序如下:
import java.io.IOException;
import java.net.URL;
import java.util.Date;
import java.util.Enumeration;
public class MyClass{
public static void main(String[] args) {
System.out.println("hello world");
ThisClass tc = new ThisClass();
// 方式1
try {
System.out.println("class loader 1: " + tc.getClass().getClassLoader());
Enumeration<URL> cpurl1 = tc.getClass().getClassLoader().getResources("");
while (cpurl1.hasMoreElements()) {
URL cp1 = cpurl1.nextElement();
System.out.println("cp1=" + cp1);
}
System.out.println();
} catch (IOException e) {
e.printStackTrace();
}
// 方式2
try {
System.out.println("class loader 2: " + ClassLoader.getSystemClassLoader());
Enumeration<URL> cpurl2 = ClassLoader.getSystemResources("");
while (cpurl2.hasMoreElements()) {
URL cp2 = cpurl2.nextElement();
System.out.println("cp2=" + cp2);
}
System.out.println();
} catch (IOException e) {
e.printStackTrace();
}
// 方式3
try {
System.out.println("class loader 3: " + Thread.currentThread().getContextClassLoader());
Enumeration<URL> cpurl3 = Thread.currentThread().getContextClassLoader().getResources("");
while (cpurl3.hasMoreElements()) {
URL cp3 = cpurl3.nextElement();
System.out.println("cp3=" + cp3);
}
System.out.println();
} catch (IOException e) {
e.printStackTrace();
}
}
}
class ThisClass {
}
在Windows上执行结果:
hello world
class loader 1: sun.misc.Launcher$AppClassLoader@73d16e93
cp1=file:/D:/test/
cp1=file:/D:/Program%20Files/Java/jdk1.8.0_202/lib/
class loader 2: sun.misc.Launcher$AppClassLoader@73d16e93
cp2=file:/D:/test/
cp2=file:/D:/Program%20Files/Java/jdk1.8.0_202/lib/
class loader 3: sun.misc.Launcher$AppClassLoader@73d16e93
cp3=file:/D:/test/
cp3=file:/D:/Program%20Files/Java/jdk1.8.0_202/lib/
在Ubuntu上执行结果:来自 博客园
ben@ben-VirtualBox:~/test$ java MyClass
hello world
class loader 1: sun.misc.Launcher$AppClassLoader@75b84c92
cp1=file:/home/ben/test/
class loader 2: sun.misc.Launcher$AppClassLoader@75b84c92
cp2=file:/home/ben/test/
class loader 3: sun.misc.Launcher$AppClassLoader@75b84c92
cp3=file:/home/ben/test/
三种方式执行的ClassLoader是同一个,获取的类路径相同。来自 博客园
项目名:web-first,一个Web项目,依赖了spring-boot-starter-web、spring-cloud-starter-netflix-eureka-client包。
程序如下:
@SpringBootApplication
public class WebFirstApplication {
public static void main(String[] args) {
ConfigurableApplicationContext ctx = SpringApplication.run(WebFirstApplication.class, args);
// 方式1
try {
System.out.println("class loader 1: " + ctx.getClassLoader());
Enumeration<URL> cpurl1 = ctx.getClassLoader().getResources("");
while (cpurl1.hasMoreElements()) {
URL cp1 = cpurl1.nextElement();
System.out.println("cp1=" + cp1);
}
System.out.println();
} catch (IOException e) {
e.printStackTrace();
}
// 方式2
try {
System.out.println("class loader 2: " + ClassLoader.getSystemClassLoader());
Enumeration<URL> cpurl2 = ClassLoader.getSystemResources("");
while (cpurl2.hasMoreElements()) {
URL cp2 = cpurl2.nextElement();
System.out.println("cp2=" + cp2);
}
System.out.println();
} catch (IOException e) {
e.printStackTrace();
}
// 方式3
try {
System.out.println("class loader 3: " + Thread.currentThread().getContextClassLoader());
Enumeration<URL> cpurl3 = Thread.currentThread().getContextClassLoader().getResources("");
while (cpurl3.hasMoreElements()) {
URL cp3 = cpurl3.nextElement();
System.out.println("cp3=" + cp3);
}
System.out.println();
} catch (IOException e) {
e.printStackTrace();
}
}
}
在Eclipse执行结果:
class loader 1: jdk.internal.loader.ClassLoaders$AppClassLoader@7382f612
cp1=file:/D:/spcloud/web-first/target/classes/
cp1=jar:file:/D:/work/mvnrepo/org/apache/logging/log4j/log4j-api/2.14.1/log4j-api-2.14.1.jar!/META-INF/versions/9/
cp1=jar:file:/D:/work/mvnrepo/org/bouncycastle/bcpkix-jdk15on/1.68/bcpkix-jdk15on-1.68.jar!/META-INF/versions/9/
cp1=jar:file:/D:/work/mvnrepo/org/bouncycastle/bcprov-jdk15on/1.68/bcprov-jdk15on-1.68.jar!/META-INF/versions/15/
class loader 2: jdk.internal.loader.ClassLoaders$AppClassLoader@7382f612
cp2=file:/D:/spcloud/web-first/target/classes/
cp2=jar:file:/D:/work/mvnrepo/org/apache/logging/log4j/log4j-api/2.14.1/log4j-api-2.14.1.jar!/META-INF/versions/9/
cp2=jar:file:/D:/work/mvnrepo/org/bouncycastle/bcpkix-jdk15on/1.68/bcpkix-jdk15on-1.68.jar!/META-INF/versions/9/
cp2=jar:file:/D:/work/mvnrepo/org/bouncycastle/bcprov-jdk15on/1.68/bcprov-jdk15on-1.68.jar!/META-INF/versions/15/
class loader 3: jdk.internal.loader.ClassLoaders$AppClassLoader@7382f612
cp3=file:/D:/spcloud/web-first/target/classes/
cp3=jar:file:/D:/work/mvnrepo/org/apache/logging/log4j/log4j-api/2.14.1/log4j-api-2.14.1.jar!/META-INF/versions/9/
cp3=jar:file:/D:/work/mvnrepo/org/bouncycastle/bcpkix-jdk15on/1.68/bcpkix-jdk15on-1.68.jar!/META-INF/versions/9/
cp3=jar:file:/D:/work/mvnrepo/org/bouncycastle/bcprov-jdk15on/1.68/bcprov-jdk15on-1.68.jar!/META-INF/versions/15/
三种方式的 ClassLoader对象是同一个,类路径也是同一个。
疑问:
作为一个Spring Boot的Web项目,类路径中怎么没有 spring相关的Jar包呢?
首先打包web-first项目,得到:web-first-0.0.1-SNAPSHOT.jar;
执行:java -jar web-first-0.0.1-SNAPSHOT.jar
控制台输出:
点击查看代码
class loader 1: org.springframework.boot.loader.LaunchedURLClassLoader@2e5d6d97
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/classes!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-boot-2.5.2.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-boot-autoconfigure-2.5.2.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/logback-classic-1.2.3.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/logback-core-1.2.3.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/log4j-to-slf4j-2.14.1.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/log4j-api-2.14.1.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jul-to-slf4j-1.7.31.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jakarta.annotation-api-1.3.5.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/snakeyaml-1.28.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jackson-databind-2.12.3.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jackson-datatype-jdk8-2.12.3.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jackson-datatype-jsr310-2.12.3.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jackson-module-parameter-names-2.12.3.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/tomcat-embed-core-9.0.48.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/tomcat-embed-el-9.0.48.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/tomcat-embed-websocket-9.0.48.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-web-5.3.8.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-beans-5.3.8.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-webmvc-5.3.8.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-aop-5.3.8.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-context-5.3.8.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-expression-5.3.8.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-cloud-starter-netflix-eureka-client-3.0.3.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-cloud-starter-3.0.3.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-cloud-context-3.0.3.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-security-crypto-5.5.1.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-cloud-commons-3.0.3.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-security-rsa-1.0.10.RELEASE.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/bcpkix-jdk15on-1.68.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/bcprov-jdk15on-1.68.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-cloud-netflix-eureka-client-3.0.3.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/eureka-client-1.10.14.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/netflix-eventbus-0.3.0.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/netflix-infix-0.3.0.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/commons-jxpath-1.3.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/joda-time-2.3.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/antlr-runtime-3.4.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/stringtemplate-3.2.1.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/antlr-2.7.7.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/gson-2.8.7.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/commons-math-2.2.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/xstream-1.4.16.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/mxparser-1.2.1.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/xmlpull-1.1.3.1.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jsr311-api-1.1.1.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/servo-core-0.12.21.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/guava-19.0.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/httpclient-4.5.13.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/httpcore-4.4.14.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/commons-codec-1.15.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/commons-configuration-1.10.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/commons-lang-2.6.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/guice-4.1.0.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/javax.inject-1.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/aopalliance-1.0.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jackson-annotations-2.12.3.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jackson-core-2.12.3.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jettison-1.4.0.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/eureka-core-1.10.14.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/woodstox-core-6.2.1.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/stax2-api-4.2.1.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-cloud-starter-loadbalancer-3.0.3.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-cloud-loadbalancer-3.0.3.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/hibernate-validator-6.2.0.Final.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jakarta.validation-api-2.0.2.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jboss-logging-3.4.2.Final.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/classmate-1.5.1.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/reactor-core-3.4.7.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/reactive-streams-1.0.3.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/reactor-extra-3.4.3.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-context-support-5.3.8.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/evictor-1.0.0.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/slf4j-api-1.7.31.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-core-5.3.8.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-jcl-5.3.8.jar!/
cp1=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-boot-jarmode-layertools-2.5.2.jar!/
class loader 2: sun.misc.Launcher$AppClassLoader@5c647e05
class loader 3: org.springframework.boot.loader.LaunchedURLClassLoader@2e5d6d97
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/classes!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-boot-2.5.2.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-boot-autoconfigure-2.5.2.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/logback-classic-1.2.3.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/logback-core-1.2.3.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/log4j-to-slf4j-2.14.1.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/log4j-api-2.14.1.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jul-to-slf4j-1.7.31.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jakarta.annotation-api-1.3.5.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/snakeyaml-1.28.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jackson-databind-2.12.3.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jackson-datatype-jdk8-2.12.3.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jackson-datatype-jsr310-2.12.3.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jackson-module-parameter-names-2.12.3.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/tomcat-embed-core-9.0.48.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/tomcat-embed-el-9.0.48.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/tomcat-embed-websocket-9.0.48.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-web-5.3.8.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-beans-5.3.8.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-webmvc-5.3.8.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-aop-5.3.8.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-context-5.3.8.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-expression-5.3.8.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-cloud-starter-netflix-eureka-client-3.0.3.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-cloud-starter-3.0.3.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-cloud-context-3.0.3.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-security-crypto-5.5.1.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-cloud-commons-3.0.3.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-security-rsa-1.0.10.RELEASE.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/bcpkix-jdk15on-1.68.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/bcprov-jdk15on-1.68.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-cloud-netflix-eureka-client-3.0.3.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/eureka-client-1.10.14.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/netflix-eventbus-0.3.0.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/netflix-infix-0.3.0.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/commons-jxpath-1.3.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/joda-time-2.3.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/antlr-runtime-3.4.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/stringtemplate-3.2.1.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/antlr-2.7.7.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/gson-2.8.7.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/commons-math-2.2.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/xstream-1.4.16.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/mxparser-1.2.1.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/xmlpull-1.1.3.1.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jsr311-api-1.1.1.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/servo-core-0.12.21.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/guava-19.0.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/httpclient-4.5.13.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/httpcore-4.4.14.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/commons-codec-1.15.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/commons-configuration-1.10.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/commons-lang-2.6.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/guice-4.1.0.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/javax.inject-1.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/aopalliance-1.0.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jackson-annotations-2.12.3.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jackson-core-2.12.3.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jettison-1.4.0.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/eureka-core-1.10.14.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/woodstox-core-6.2.1.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/stax2-api-4.2.1.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-cloud-starter-loadbalancer-3.0.3.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-cloud-loadbalancer-3.0.3.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/hibernate-validator-6.2.0.Final.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jakarta.validation-api-2.0.2.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jboss-logging-3.4.2.Final.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/classmate-1.5.1.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/reactor-core-3.4.7.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/reactive-streams-1.0.3.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/reactor-extra-3.4.3.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-context-support-5.3.8.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/evictor-1.0.0.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/slf4j-api-1.7.31.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-core-5.3.8.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-jcl-5.3.8.jar!/
cp3=jar:file:/D:/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-boot-jarmode-layertools-2.5.2.jar!/
class loader 1、3相同,有配置classpath,而class locader 2存在,但classpath为空。
使用这种方式执行,可以看到程序依赖的Jar包了。
可以看出,打包得到的web-first-0.0.1-SNAPSHOT.jar已经包含了各个依赖包了。
点击查看代码
class loader 1: org.springframework.boot.loader.LaunchedURLClassLoader@5a10411
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/classes!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-boot-2.5.2.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-boot-autoconfigure-2.5.2.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/logback-classic-1.2.3.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/logback-core-1.2.3.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/log4j-to-slf4j-2.14.1.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/log4j-api-2.14.1.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jul-to-slf4j-1.7.31.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jakarta.annotation-api-1.3.5.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/snakeyaml-1.28.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jackson-databind-2.12.3.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jackson-datatype-jdk8-2.12.3.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jackson-datatype-jsr310-2.12.3.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jackson-module-parameter-names-2.12.3.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/tomcat-embed-core-9.0.48.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/tomcat-embed-el-9.0.48.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/tomcat-embed-websocket-9.0.48.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-web-5.3.8.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-beans-5.3.8.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-webmvc-5.3.8.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-aop-5.3.8.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-context-5.3.8.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-expression-5.3.8.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-cloud-starter-netflix-eureka-client-3.0.3.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-cloud-starter-3.0.3.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-cloud-context-3.0.3.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-security-crypto-5.5.1.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-cloud-commons-3.0.3.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-security-rsa-1.0.10.RELEASE.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/bcpkix-jdk15on-1.68.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/bcprov-jdk15on-1.68.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-cloud-netflix-eureka-client-3.0.3.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/eureka-client-1.10.14.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/netflix-eventbus-0.3.0.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/netflix-infix-0.3.0.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/commons-jxpath-1.3.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/joda-time-2.3.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/antlr-runtime-3.4.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/stringtemplate-3.2.1.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/antlr-2.7.7.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/gson-2.8.7.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/commons-math-2.2.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/xstream-1.4.16.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/mxparser-1.2.1.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/xmlpull-1.1.3.1.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jsr311-api-1.1.1.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/servo-core-0.12.21.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/guava-19.0.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/httpclient-4.5.13.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/httpcore-4.4.14.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/commons-codec-1.15.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/commons-configuration-1.10.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/commons-lang-2.6.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/guice-4.1.0.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/javax.inject-1.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/aopalliance-1.0.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jackson-annotations-2.12.3.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jackson-core-2.12.3.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jettison-1.4.0.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/eureka-core-1.10.14.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/woodstox-core-6.2.1.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/stax2-api-4.2.1.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-cloud-starter-loadbalancer-3.0.3.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-cloud-loadbalancer-3.0.3.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/hibernate-validator-6.2.0.Final.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jakarta.validation-api-2.0.2.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jboss-logging-3.4.2.Final.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/classmate-1.5.1.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/reactor-core-3.4.7.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/reactive-streams-1.0.3.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/reactor-extra-3.4.3.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-context-support-5.3.8.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/evictor-1.0.0.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/slf4j-api-1.7.31.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-core-5.3.8.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-jcl-5.3.8.jar!/
cp1=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-boot-jarmode-layertools-2.5.2.jar!/
class loader 2: sun.misc.Launcher$AppClassLoader@677327b6
class loader 3: org.springframework.boot.loader.LaunchedURLClassLoader@5a10411
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/classes!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-boot-2.5.2.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-boot-autoconfigure-2.5.2.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/logback-classic-1.2.3.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/logback-core-1.2.3.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/log4j-to-slf4j-2.14.1.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/log4j-api-2.14.1.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jul-to-slf4j-1.7.31.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jakarta.annotation-api-1.3.5.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/snakeyaml-1.28.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jackson-databind-2.12.3.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jackson-datatype-jdk8-2.12.3.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jackson-datatype-jsr310-2.12.3.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jackson-module-parameter-names-2.12.3.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/tomcat-embed-core-9.0.48.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/tomcat-embed-el-9.0.48.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/tomcat-embed-websocket-9.0.48.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-web-5.3.8.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-beans-5.3.8.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-webmvc-5.3.8.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-aop-5.3.8.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-context-5.3.8.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-expression-5.3.8.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-cloud-starter-netflix-eureka-client-3.0.3.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-cloud-starter-3.0.3.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-cloud-context-3.0.3.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-security-crypto-5.5.1.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-cloud-commons-3.0.3.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-security-rsa-1.0.10.RELEASE.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/bcpkix-jdk15on-1.68.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/bcprov-jdk15on-1.68.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-cloud-netflix-eureka-client-3.0.3.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/eureka-client-1.10.14.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/netflix-eventbus-0.3.0.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/netflix-infix-0.3.0.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/commons-jxpath-1.3.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/joda-time-2.3.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/antlr-runtime-3.4.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/stringtemplate-3.2.1.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/antlr-2.7.7.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/gson-2.8.7.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/commons-math-2.2.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/xstream-1.4.16.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/mxparser-1.2.1.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/xmlpull-1.1.3.1.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jsr311-api-1.1.1.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/servo-core-0.12.21.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/guava-19.0.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/httpclient-4.5.13.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/httpcore-4.4.14.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/commons-codec-1.15.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/commons-configuration-1.10.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/commons-lang-2.6.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/guice-4.1.0.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/javax.inject-1.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/aopalliance-1.0.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jackson-annotations-2.12.3.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jackson-core-2.12.3.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jettison-1.4.0.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/eureka-core-1.10.14.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/woodstox-core-6.2.1.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/stax2-api-4.2.1.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-cloud-starter-loadbalancer-3.0.3.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-cloud-loadbalancer-3.0.3.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/hibernate-validator-6.2.0.Final.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jakarta.validation-api-2.0.2.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/jboss-logging-3.4.2.Final.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/classmate-1.5.1.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/reactor-core-3.4.7.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/reactive-streams-1.0.3.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/reactor-extra-3.4.3.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-context-support-5.3.8.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/evictor-1.0.0.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/slf4j-api-1.7.31.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-core-5.3.8.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-jcl-5.3.8.jar!/
cp3=jar:file:/home/ben/web-first-0.0.1-SNAPSHOT.jar!/BOOT-INF/lib/spring-boot-jarmode-layertools-2.5.2.jar!/
和 2.2节的结果对比,除了 class loader的地址不同、classpath的路径不同,其它完全相同。
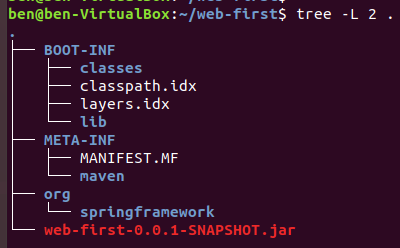
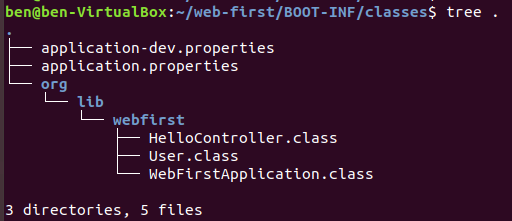
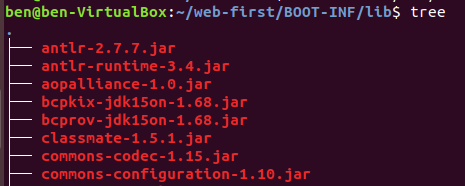
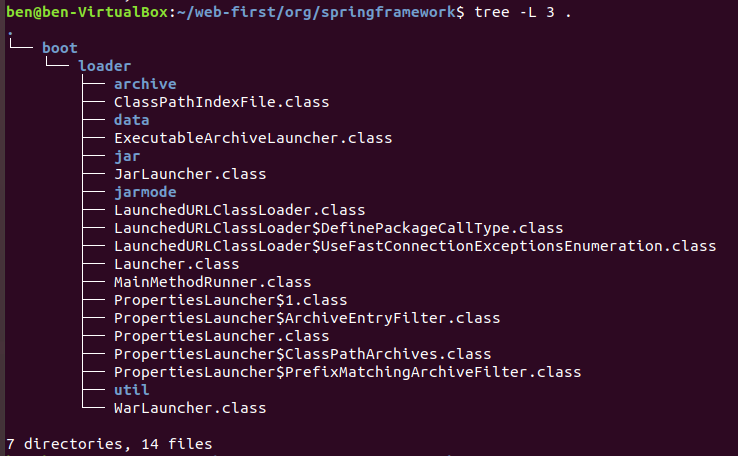
jar包中部分文件的内容:来自 博客园
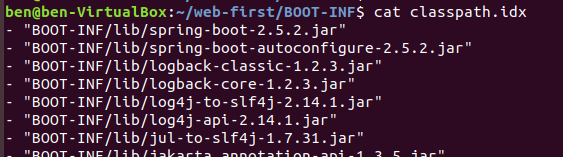
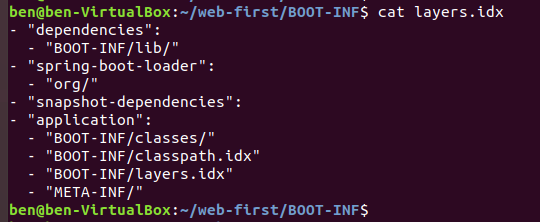
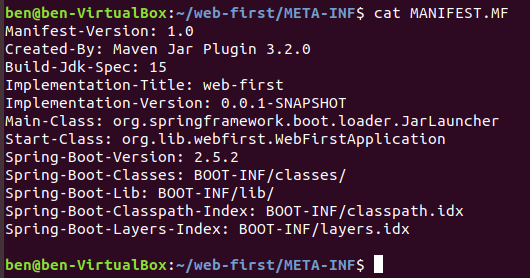
想要了解spring boot程序是如何启动的,需要清楚这些文件在执行 java -jar 时是怎么用的,TODO
1、java打印出classpath_JAVA获取CLASSPATH路径
5、