一 Vue路由
https://router.vuejs.org/zh/installation.html Vue Router
https://cn.vuejs.org/v2/guide/routing.html 官方路由
对于大多数单页面应用,都推荐使用官方支持的 vue-router 库。更多细节可以移步 vue-router 文档。
https://www.runoob.com/vue2/vue-routing.html 菜鸟路由
1.路由语法基础使用(单路由vue1.9版之前)
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Document</title> <script src="bower_components/vue/dist/vue.js"></script> <script src="bower_components/vue-router/dist/vue-router.js"></script> <style> </style> </head> <body> <div id="box"> <ul> <li> <!--路由必须用v-link来实现这固定死了的 路由提供的--> <a v-link="{path:'/home'}">主页</a> </li> <li> <a v-link="{path:'/news'}">新闻</a> </li> </ul> <!--//路由必须提供一个div展示 必须包含<router-view></router-view> 展示内容--> <div> <router-view></router-view> </div> </div> <!--路由设计 根据不同url地址,出现不同效果--> <script> //1. 准备一个根组件 var App=Vue.extend(); //2. Home News组件都准备 var Home=Vue.extend({ template:'<h3>我zhu是主页</h3>' }); var News=Vue.extend({ template:'<h3>我xin是新闻</h3>' }); //3. 准备路由 var router=new VueRouter(); //4. 关联 router.map({ 'home':{ component:Home }, 'news':{ component:News } }); //5. 启动路由 router.start(App,'#box'); </script> </body> </html>
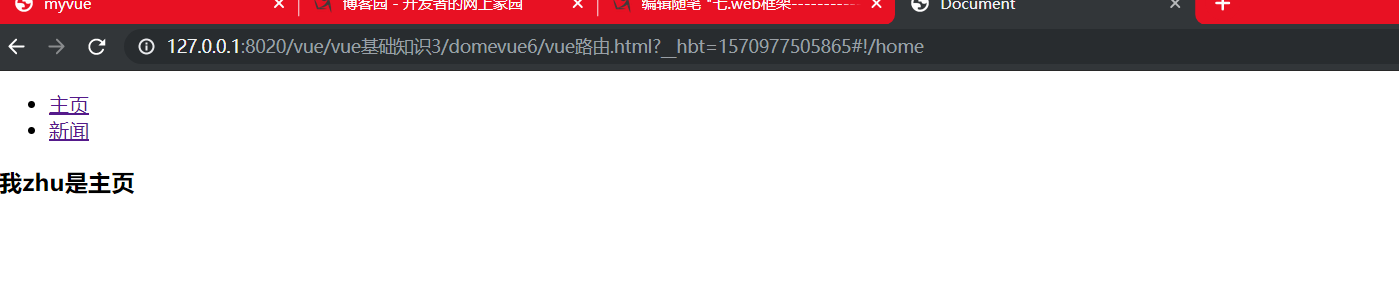
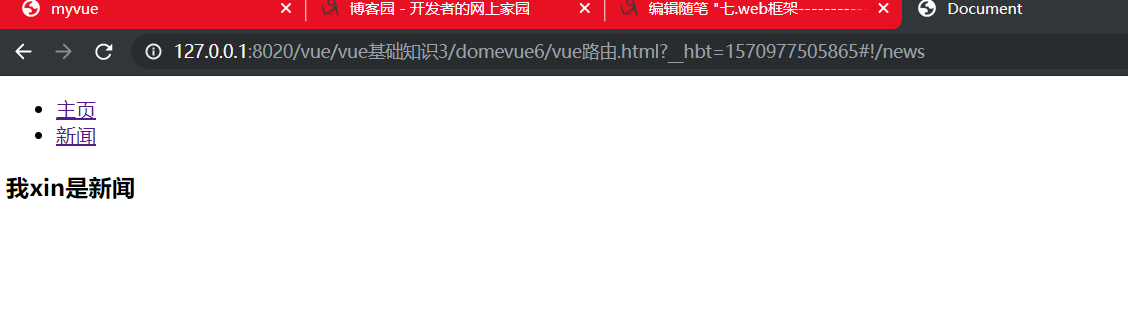
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Document</title> <script src="bower_components/vue/dist/vue.js"></script> <script src="bower_components/vue-router/dist/vue-router.js"></script> <style> </style> </head> <body> <div id="box"> <ul> <li> <a v-link="{path:'/home'}">主页</a> </li> <li> <a v-link="{path:'/news'}">新闻</a> </li> </ul> <div> <router-view></router-view> </div> </div> <script> //1. 准备一个根组件 var App=Vue.extend(); //2. Home News组件都准备 var Home=Vue.extend({ template:'<h3>zzzzz我是主页</h3>' }); var News=Vue.extend({ template:'<h3>xxxx我是新闻</h3>' }); //3. 准备路由 var router=new VueRouter(); //4. 关联 router.map({ 'home':{ component:Home }, 'news':{ component:News } }); //5. 启动路由 router.start(App,'#box'); //6. 跳转 router.redirect({ '/':'/home' }); </script> </body> </html>
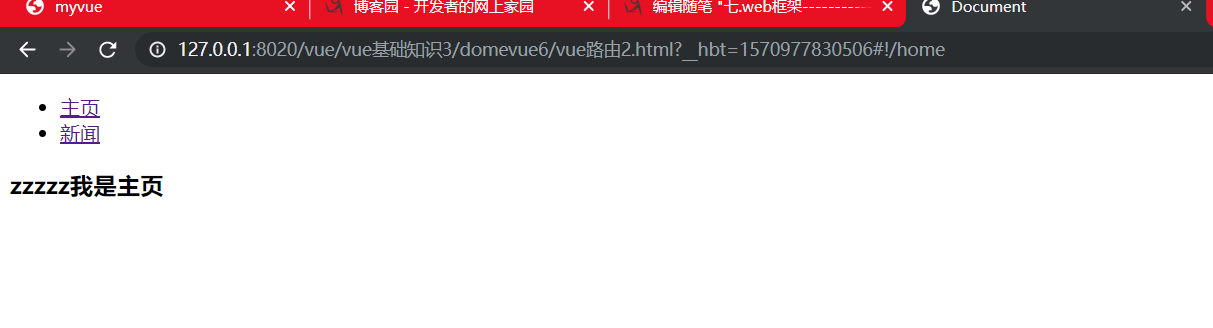
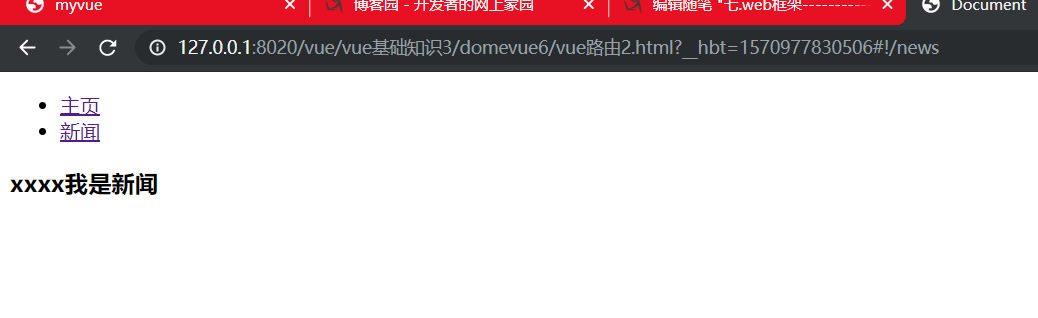
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Document</title> <script src="bower_components/vue/dist/vue.js"></script> <script src="bower_components/vue-router/dist/vue-router.js"></script> <style> </style> </head> <body> <div id="box"> <ul> <li> <a v-link="{path:'/home'}">主页</a> </li> <hr /> <li> <a v-link="{path:'/news'}">新闻</a> </li> </ul> <div> <router-view></router-view> </div> </div> <script> //1. 准备一个根组件 var App=Vue.extend(); //2. Home News组件都准备 var Home=Vue.extend({ template:'<h3>我是主页</h3>' }); var News=Vue.extend({ template:'<h3>我是新闻</h3>' }); //3. 准备路由 var router=new VueRouter(); //4. 关联 router.map({ 'home':{ component:Home }, 'news':{ component:News } }); //5. 启动路由 router.start(App,'#box'); //6. 跳转redirect router.redirect({ '/':'home' }); </script> </body> </html>
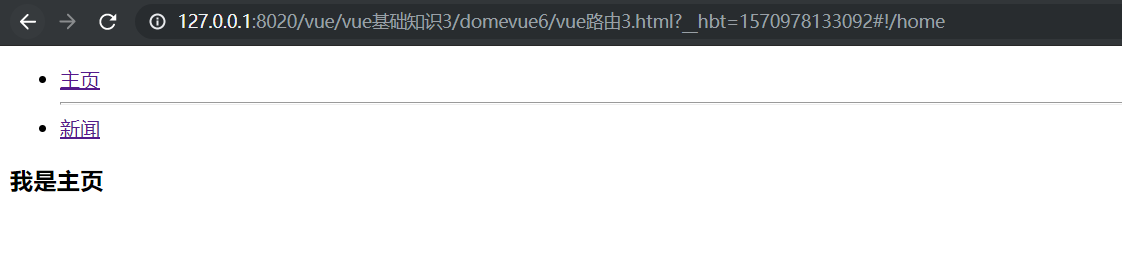
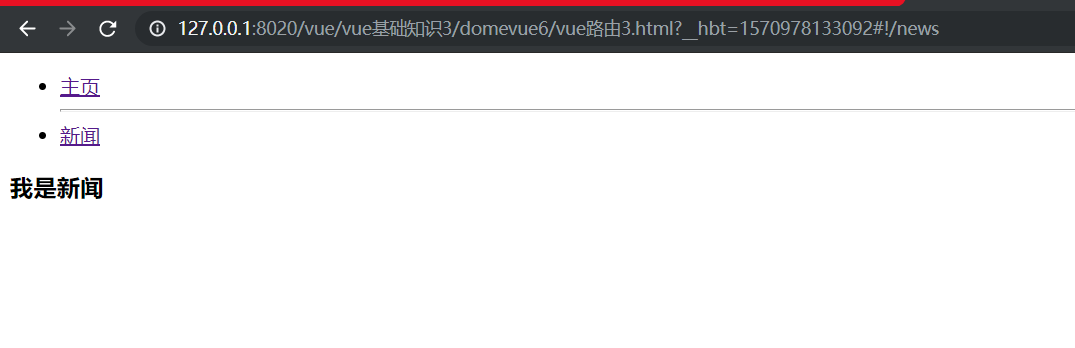
2.路由语法基础使用(多路由 vue1.9版之前)
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Document</title> <script src="bower_components/vue/dist/vue.js"></script> <script src="bower_components/vue-router/dist/vue-router.js"></script> <style> .v-link-active{ font-size: 20px; color: #f60; } </style> </head> <body> <div id="box"> <ul> <li> <a v-link="{path:'/home'}">主页</a> </li> <li> <a v-link="{path:'/news'}">新闻</a> </li> </ul> <div> <router-view></router-view> </div> </div> <template id="home"> <h3>我是主页</h3> <div> <a v-link="{path:'/home/login'}">登录</a> <a v-link="{path:'/home/reg'}">注册</a> </div> <div> <router-view></router-view> </div> </template> <template id="news"> <h3>我是新闻</h3> </template> <script> //1. 准备一个根组件 var App=Vue.extend(); //2. Home News组件都准备 var Home=Vue.extend({ template:'#home' }); var News=Vue.extend({ template:'#news' }); //3. 准备路由 var router=new VueRouter(); //4. 关联 router.map({ 'home':{ component:Home, subRoutes:{ 'login':{ component:{ template:'<strong>我是登录信息</strong>' } }, 'reg':{ component:{ template:'<strong>我是注册信息</strong>' } } } }, 'news':{ component:News } }); //5. 启动路由 router.start(App,'#box'); //6. 跳转 router.redirect({ '/':'home' }); </script> </body> </html>
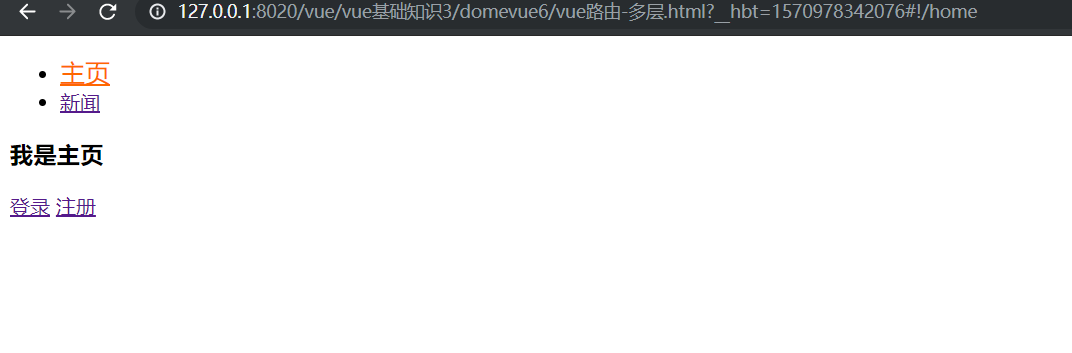
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Document</title> <script src="bower_components/vue/dist/vue.js"></script> <script src="bower_components/vue-router/dist/vue-router.js"></script> <style> .v-link-active{ font-size: 20px; color: #f60; } </style> </head> <body> <div id="box"> <ul> <li> <a v-link="{path:'/home'}">主页</a> </li> <li> <a v-link="{path:'/news'}">新闻</a> </li> </ul> <div> <router-view></router-view> </div> </div> <template id="home"> <h3>我是主页</h3> <div> <a v-link="{path:'/home/login'}">登录</a> <a v-link="{path:'/home/reg'}">注册</a> </div> <div> <router-view></router-view> </div> </template> <template id="news"> <h3>我是新闻</h3> <div> <a v-link="{path:'/news/detail/001'}">新闻001</a> <a v-link="{path:'/news/detail/002'}">新闻002</a> </div> <router-view></router-view> </template> <template id="detail"> {{$route.params | json}} <!--{{$route.params | json}} -> 当前参数--> </template> <script> //1. 准备一个根组件 var App=Vue.extend(); //2. Home News组件都准备 var Home=Vue.extend({ template:'#home' }); var News=Vue.extend({ template:'#news' }); var Detail=Vue.extend({ template:'#detail' }); //3. 准备路由 var router=new VueRouter(); //4. 关联 router.map({ 'home':{ component:Home, subRoutes:{ 'login':{ component:{ template:'<strong>我是登录信息</strong>' } }, 'reg':{ component:{ template:'<strong>我是注册信息</strong>' } } } }, 'news':{ component:News, subRoutes:{ '/detail/:id':{ component:Detail } } } }); //5. 启动路由 router.start(App,'#box'); //6. 跳转 router.redirect({ '/':'home' }); </script> </body> </html>
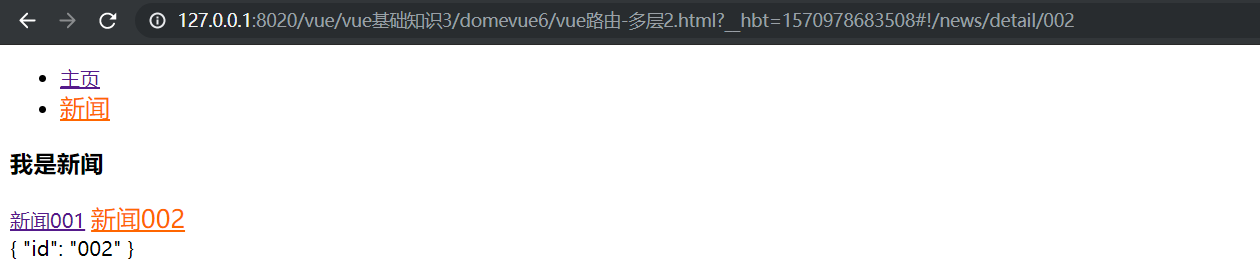
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Document</title> <script src="bower_components/vue/dist/vue.js"></script> <script src="bower_components/vue-router/dist/vue-router.js"></script> <style> .v-link-active{ font-size: 20px; color: #f60; } </style> </head> <body> <div id="box"> <ul> <li> <a v-link="{path:'/home'}">主页</a> </li> <li> <a v-link="{path:'/news'}">新闻</a> </li> </ul> <div> <router-view></router-view> </div> </div> <template id="home"> <h3>我是主页</h3> <div> <a v-link="{path:'/home/login'}">登录</a> <a v-link="{path:'/home/reg'}">注册</a> </div> <div> <router-view></router-view> </div> </template> <template id="news"> <h3>我是新闻</h3> <div> <a v-link="{path:'/news/detail/001'}">新闻001</a> <a v-link="{path:'/news/detail/002'}">新闻002</a> </div> <router-view></router-view> </template> <template id="detail"> {{$route.params | json}} <!--当前参数-->------ 当前参数 <br> {{$route.path}} <!--{{$route.path}} -> 当前路径--> -------------当前路径 <br> {{$route.query | json}} <!-- {{$route.query | json}} -> ------------------ 数据--> -------------数据 </template> <script> //1. 准备一个根组件 var App=Vue.extend(); //2. Home News组件都准备 var Home=Vue.extend({ template:'#home' }); var News=Vue.extend({ template:'#news' }); var Detail=Vue.extend({ template:'#detail' }); //3. 准备路由 var router=new VueRouter(); //4. 关联 router.map({ 'home':{ component:Home, subRoutes:{ 'login':{ component:{ template:'<strong>我是登录信息</strong>' } }, 'reg':{ component:{ template:'<strong>我是注册信息</strong>' } } } }, 'news':{ component:News, subRoutes:{ '/detail/:id':{ component:Detail } } } }); //5. 启动路由 router.start(App,'#box'); //6. 跳转 router.redirect({ '/':'home' }); </script> </body> </html>
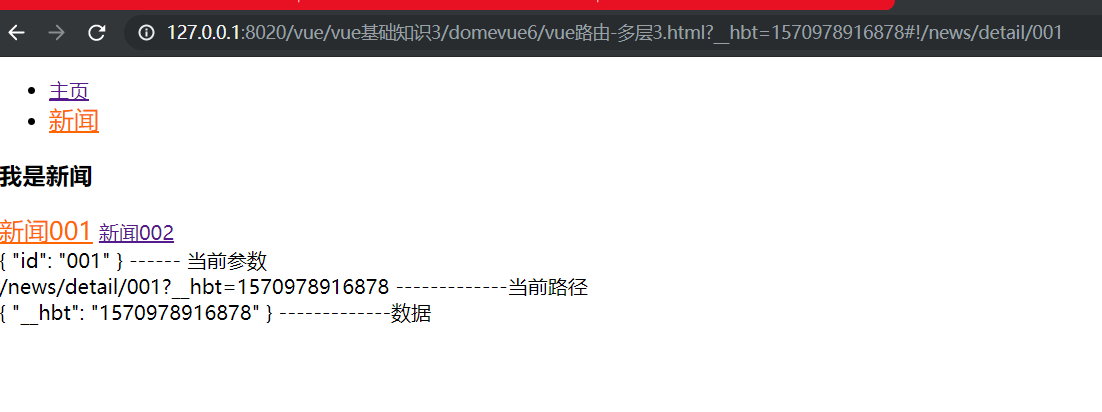
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Document</title> <script src="bower_components/vue/dist/vue.js"></script> <script src="bower_components/vue-router/dist/vue-router.js"></script> <style> .v-link-active{ font-size: 20px; color: #f60; } </style> </head> <body> <div id="box"> <ul> <li> <a v-link="{path:'/home'}">主页</a> </li> <li> <a v-link="{path:'/news'}">新闻</a> </li> </ul> <div> <router-view></router-view> </div> </div> <template id="home"> <h3>我是主页</h3> <div> <a v-link="{path:'/home/login/zns'}">登录</a> <a v-link="{path:'/home/reg/strive'}">注册</a> </div> <div> <router-view></router-view> </div> </template> <template id="news"> <h3>我是新闻</h3> <div> <a v-link="{path:'/news/detail/001'}">新闻001</a> <a v-link="{path:'/news/detail/002'}">新闻002</a> </div> <router-view></router-view> </template> <template id="detail"> {{$route.params | json}} <br> {{$route.path}} <br> {{$route.query | json}} </template> <script> //1. 准备一个根组件 var App=Vue.extend(); //2. Home News组件都准备 var Home=Vue.extend({ template:'#home' }); var News=Vue.extend({ template:'#news' }); var Detail=Vue.extend({ template:'#detail' }); //3. 准备路由 var router=new VueRouter(); //4. 关联 router.map({ 'home':{ component:Home, subRoutes:{ 'login/:name':{ component:{ template:'<strong>我是登录信息 {{$route.params | json}}</strong>' } }, 'reg':{ component:{ template:'<strong>我是注册信息</strong>' } } } }, 'news':{ component:News, subRoutes:{ '/detail/:id':{ component:Detail } } } }); //5. 启动路由 router.start(App,'#box'); //6. 跳转 router.redirect({ '/':'home' }); </script> </body> </html>
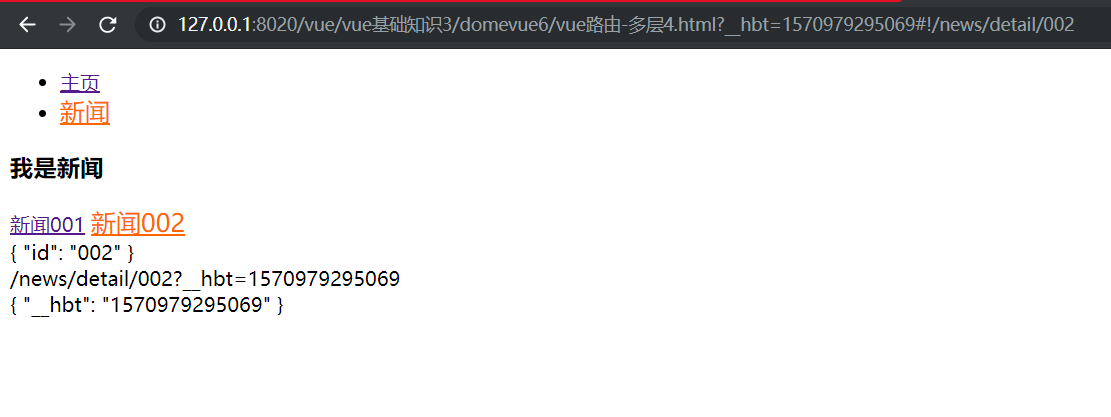
3. 路由基础使用(vue2.0版 单路由)
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>智能社——http://www.zhinengshe.com</title> <meta name="viewport" content="width=device-width, initial-scale=1.0, maximum-scale=1.0, user-scalable=0"> <meta name="apple-mobile-web-app-capable" content="yes"> <meta name="apple-mobile-web-app-status-bar-style" content="black"> <style> </style> <script src="vue2.js"></script> <script src="bower_components/vue-router/dist/vue-router.min.js"></script> </head> <body> <div id="box"> <div> <router-link to="/home">主页</router-link> <router-link to="/news">新闻</router-link> </div> <div> <router-view></router-view> </div> </div> <script> //组件 var Home={ template:'<h3>home我是主页</h3>' }; var News={ template:'<h3>nwe我是新闻</h3>' }; //配置路由 const routes=[ {path:'/home', component:Home}, {path:'/news', component:News}, {path:'*', redirect:'/home'} ]; //生成路由实例 const router=new VueRouter({ routes }); //最后挂到vue上 new Vue({ router, el:'#box' }); </script> </body> </html>
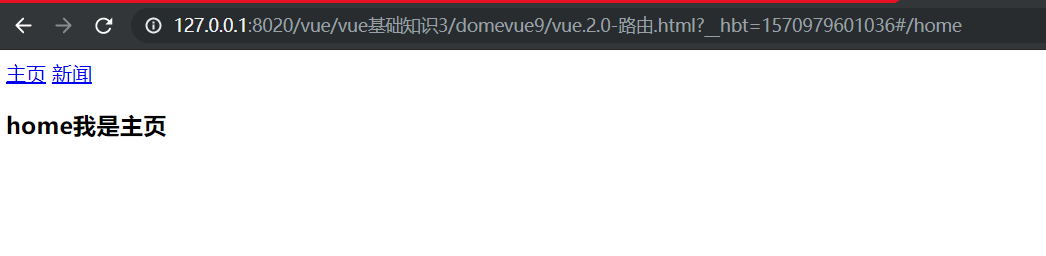
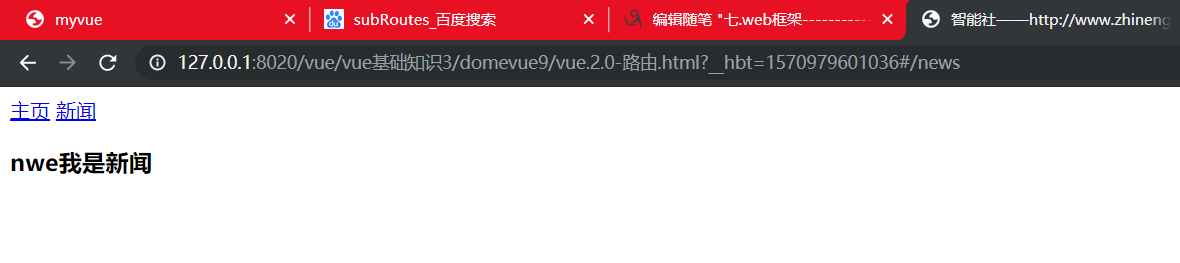
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>智能社——http://www.zhinengshe.com</title> <meta name="viewport" content="width=device-width, initial-scale=1.0, maximum-scale=1.0, user-scalable=0"> <meta name="apple-mobile-web-app-capable" content="yes"> <meta name="apple-mobile-web-app-status-bar-style" content="black"> <style> .router-link-active{ font-size: 20px; color:#f60; } </style> <script src="vue2.js"></script> <script src="bower_components/vue-router/dist/vue-router.min.js"></script> </head> <body> <div id="box"> <div> <router-link to="/home">主页</router-link> <router-link to="/news">新闻</router-link> </div> <div> <router-view></router-view> </div> </div> <script> //组件 var Home={ template:'<h3>我是主页</h3>' }; var News={ template:'<h3>我是新闻</h3>' }; //配置路由 const routes=[ {path:'/home', component:Home}, {path:'/news', component:News}, {path:'*', redirect:'/home'} ]; //生成路由实例 const router=new VueRouter({ routes }); //最后挂到vue上 new Vue({ router, el:'#box' }); </script> </body> </html>
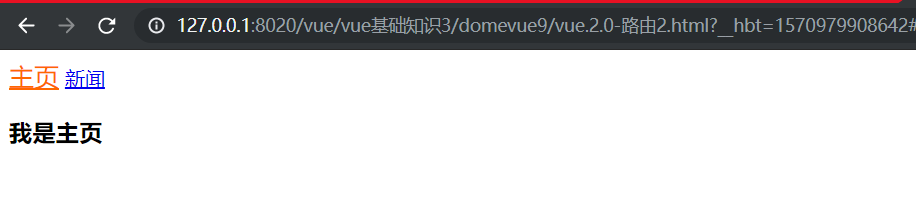
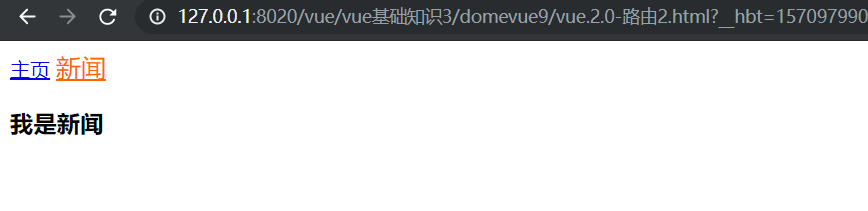
4. 路由(vue2.0版 多路由)
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>智能社——http://www.zhinengshe.com</title> <meta name="viewport" content="width=device-width, initial-scale=1.0, maximum-scale=1.0, user-scalable=0"> <meta name="apple-mobile-web-app-capable" content="yes"> <meta name="apple-mobile-web-app-status-bar-style" content="black"> <style> .router-link-active{ font-size: 20px; color:#f60; } </style> <script src="vue2.js"></script> <script src="bower_components/vue-router/dist/vue-router.min.js"></script> </head> <body> <div id="box"> <div> <router-link to="/home">主页</router-link> <router-link to="/user">用户</router-link> </div> <div> <router-view></router-view> </div> </div> <script> //组件 var Home={ template:'<h3>我是主页</h3>' }; var User={ template:` <div> <ul> <h3>我是用户信息</h3> <li><router-link to="/user/username">某个用户</router-link></li> </ul> <div> <router-view></router-view> </div> </div> ` }; var UserDetail={ template:'<div>我是XX用户</div>' }; //配置路由 const routes=[ {path:'/home', component:Home}, { path:'/user', component:User, children:[ {path:'username', component:UserDetail} ] }, {path:'*', redirect:'/home'} //404 ]; //生成路由实例 const router=new VueRouter({ routes }); //最后挂到vue上 new Vue({ router, el:'#box' }); </script> </body> </html>
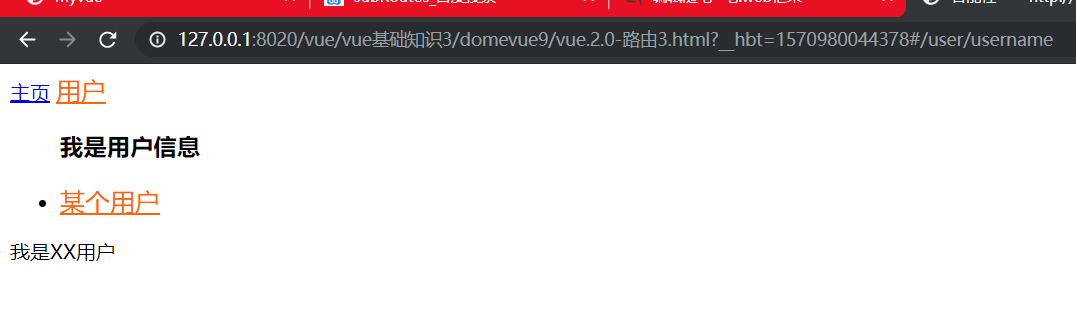
vue.2.0-路由配合运动 1
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>智能社——http://www.zhinengshe.com</title> <meta name="viewport" content="width=device-width, initial-scale=1.0, maximum-scale=1.0, user-scalable=0"> <meta name="apple-mobile-web-app-capable" content="yes"> <meta name="apple-mobile-web-app-status-bar-style" content="black"> <style> .router-link-active{ font-size: 20px; color:#f60; } </style> <script src="vue.js"></script> <script src="bower_components/vue-router/dist/vue-router.min.js"></script> </head> <body> <div id="box"> <div> <router-link to="/home">主页</router-link> <router-link to="/user">用户</router-link> </div> <div> <router-view></router-view> </div> </div> <script> //组件 var Home={ template:'<h3>我是主页</h3>' }; var User={ template:` <div> <h3>我是用户信息</h3> <ul> <li><router-link to="/user/strive/age/10">Strive</router-link></li> <li><router-link to="/user/blue/age/80">Blue</router-link></li> <li><router-link to="/user/eric/age/70">Eric</router-link></li> </ul> <div> <router-view></router-view> </div> </div> ` }; var UserDetail={ template:'<div>{{$route.params}}</div>' }; //配置路由 const routes=[ {path:'/home', component:Home}, { path:'/user', component:User, children:[ {path:':username/age/:age', component:UserDetail} ] }, {path:'*', redirect:'/home'} //404 ]; //生成路由实例 const router=new VueRouter({ routes }); //最后挂到vue上 new Vue({ router, el:'#box' }); </script> </body> </html>
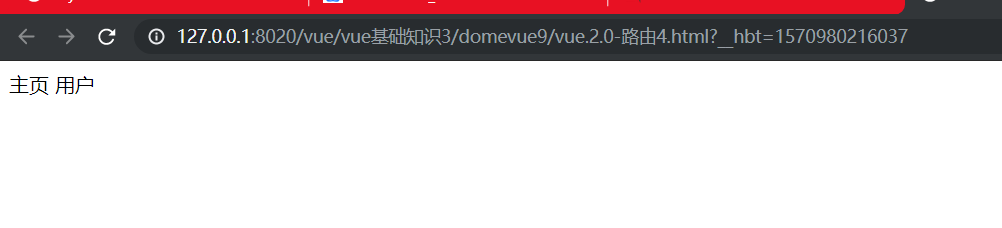
vue.2.0-路由配合运动 2
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>智能社——http://www.zhinengshe.com</title> <meta name="viewport" content="width=device-width, initial-scale=1.0, maximum-scale=1.0, user-scalable=0"> <meta name="apple-mobile-web-app-capable" content="yes"> <meta name="apple-mobile-web-app-status-bar-style" content="black"> <style> .router-link-active{ font-size: 20px; color:#f60; } </style> <script src="vue.js"></script> <script src="bower_components/vue-router/dist/vue-router.min.js"></script> </head> <body> <div id="box"> <input type="button" value="添加一个路由" @click="push"> <input type="button" value="替换一个路由" @click="replace"> <div> <router-link to="/home">主页</router-link> <router-link to="/user">用户</router-link> </div> <div> <router-view></router-view> </div> </div> <script> //组件 var Home={ template:'<h3>我是主页</h3>' }; var User={ template:` <div> <h3>我是用户信息</h3> <ul> <li><router-link to="/user/strive/age/10">Strive</router-link></li> <li><router-link to="/user/blue/age/80">Blue</router-link></li> <li><router-link to="/user/eric/age/70">Eric</router-link></li> </ul> <div> <router-view></router-view> </div> </div> ` }; var UserDetail={ template:'<div>{{$route.params}}</div>' }; //配置路由 const routes=[ {path:'/home', component:Home}, { path:'/user', component:User, children:[ {path:':username/age/:age', component:UserDetail} ] }, {path:'*', redirect:'/home'} //404 ]; //生成路由实例 const router=new VueRouter({ routes }); //最后挂到vue上 new Vue({ router, methods:{ push(){ router.push({path:'home'}); }, replace(){ router.replace({path:'user'}); } } }).$mount('#box'); </script> </body> </html>
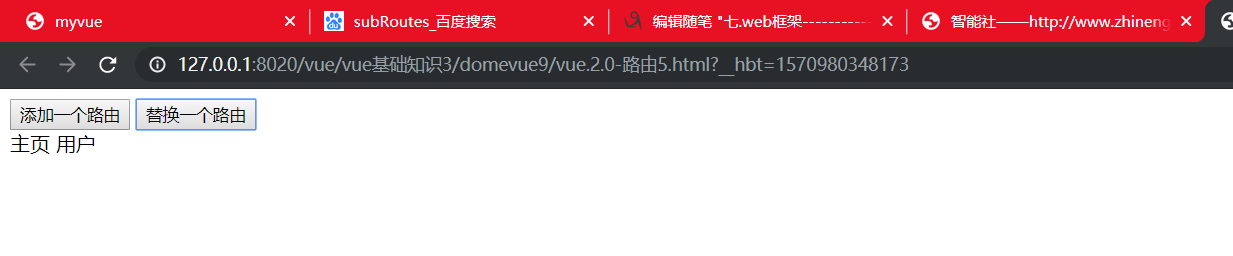
vue.2.0-路由配合运动 3
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>智能社——http://www.zhinengshe.com</title> <meta name="viewport" content="width=device-width, initial-scale=1.0, maximum-scale=1.0, user-scalable=0"> <meta name="apple-mobile-web-app-capable" content="yes"> <meta name="apple-mobile-web-app-status-bar-style" content="black"> <style> .router-link-active{ font-size: 20px; color:#f60; } </style> <script src="vue2.js"></script> <script src="bower_components/vue-router/dist/vue-router.min.js"></script> <link rel="stylesheet" href="animate.css"> </head> <body> <div id="box"> <input type="button" value="添加一个路由" @click="push"> <input type="button" value="替换一个路由" @click="replace"> <div> <router-link to="/home">主页</router-link> <router-link to="/user">用户</router-link> </div> <div> <transition enter-active-class="animated bounceInLeft" leave-active-class="animated bounceOutRight"> <router-view></router-view> </transition> </div> </div> <script> //组件 var Home={ template:'<h3>我是主页</h3>' }; var User={ template:` <div> <h3>我是用户信息</h3> <ul> <li><router-link to="/user/strive/age/10">Strive</router-link></li> <li><router-link to="/user/blue/age/80">Blue</router-link></li> <li><router-link to="/user/eric/age/70">Eric</router-link></li> </ul> <div> <router-view></router-view> </div> </div> ` }; var UserDetail={ template:'<div>{{$route.params}}</div>' }; //配置路由 const routes=[ {path:'/home', component:Home}, { path:'/user', component:User, children:[ {path:':username/age/:age', component:UserDetail} ] }, {path:'*', redirect:'/home'} //404 ]; //生成路由实例 const router=new VueRouter({ routes }); //最后挂到vue上 new Vue({ router, methods:{ push(){ router.push({path:'home'}); }, replace(){ router.replace({path:'user'}); } } }).$mount('#box'); </script> </body> </html>
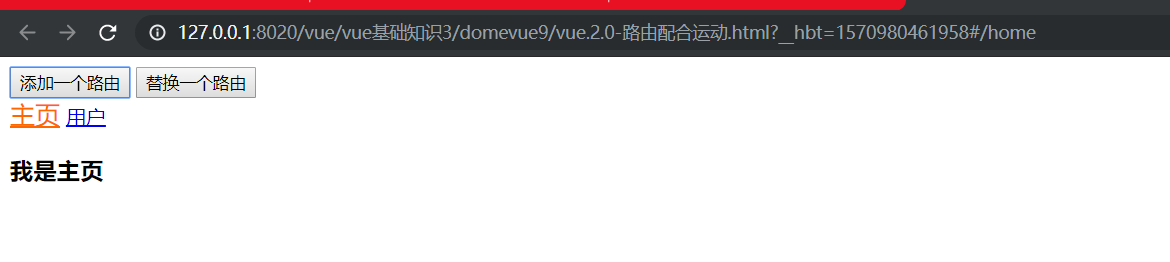
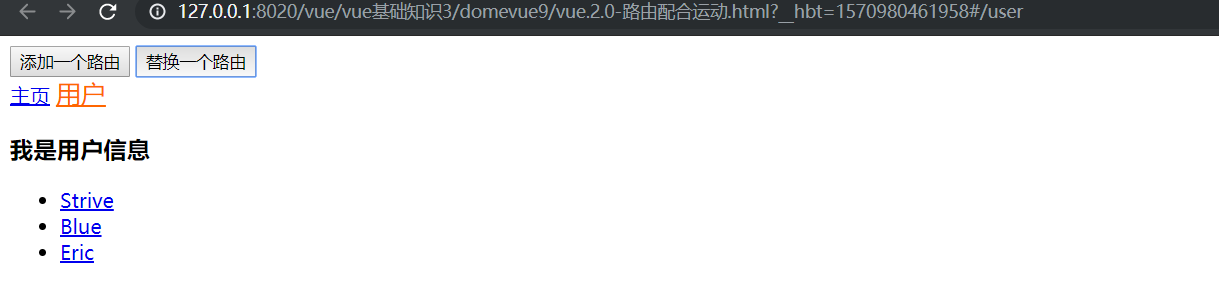
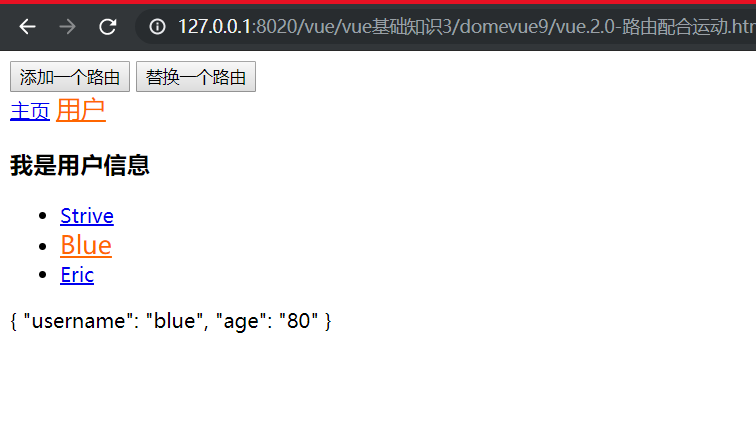
5. 路由在项目简单中使用 (下载vue-router)
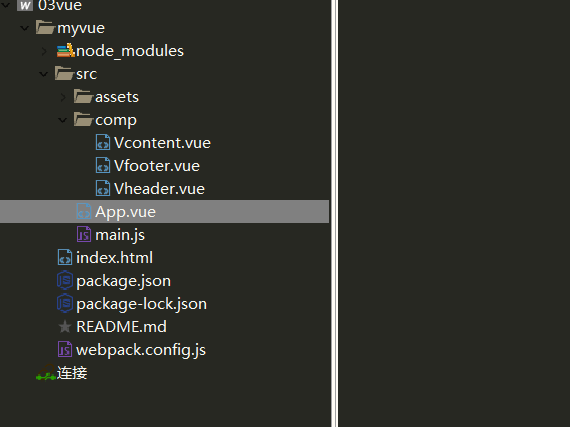
Vcontent.vue
<template> <div class="warp"> <h1>我是内容 {{msg}}</h1> </div> </template> <script> //页面的业务逻辑 export default { name: 'Vcontent', data () { return { msg: '这是content欢迎啦啦啦' } },methods:{ } } </script> <style scoped> .warp{ height: 400px; 600px; background-color: burlywood; } </style>
Vfooter.vue
<template> <footer class="footer"> <h1>我是底部 {{msg}}</h1> </footer> </template> <script> export default { name: 'Vfooter', data () { return { msg: '这是footer欢迎啦啦啦' } } } </script> <style scoped> h1{ color: blue; } .footer{ height: 150px; 500px; background-color: yellow; } </style>
Vheader.vue
<template> <header class="header"> <img :src="imgSrc" /> {{msg}} </header> </template> <!--页面逻辑--> <script> import imgSrc from '../assets/logo.png' export default { name: 'Vheader', data () { return { msg: '这是hearer欢迎啦啦啦啦啦', imgSrc: imgSrc } } } </script> <!--页面样式--> <!--scoped 这个属性表示对当前主组样式起作用--> <style scoped> .header{ 300px; height: 150px; background-color:brown; } </style>
main.js
import Vue from 'vue' import App from './App.vue' //引入组件 import Vheader from './comp/Vheader.vue' import Vcontent from './comp/Vcontent.vue' import Vfooter from './comp/Vfooter.vue' // 动态导入图片 import imgSrc from './assets/logo.png' // 引入路由组件 import vueRouter from 'vue-router' import $ from 'jquery' Vue.use(vueRouter); //配置定义路由 const routes=[ {path:'/', component:Vheader}, {path:'/content', component:Vcontent}, {path:'/foot', component:Vfooter}, ]; //生成路由实例 const router=new vueRouter({ mode:'history', routes, }); new Vue({ el: '#app', router, // 挂载路由 render: h => h(App) })
App.vue
<template> <div id="app"> <ul> <li> <!--路由必须用v-link来实现这固定死了的 路由提供的--> <a v-link="{path:'/'}"> >npm install bootstrap@3 --save主页</a> </li> <li> <a v-link="{path:'/content'}">内容</a> </li> <li> <a v-link="{path:'/foot'}">脚部</a> </li> </ul> <br /> <hr /> <router-link to="/">主页</router-link> <router-link to="/content">内容</router-link> <router-link to="/foot">脚部</router-link> <!--//路由必须提供一个div展示 必须包含<router-view></router-view> 展示内容--> <div> <router-view></router-view> </div> </div> </template> <script> // import 'bootstrap/dist/css/bootstrap.min.css' </script> <style> </style>
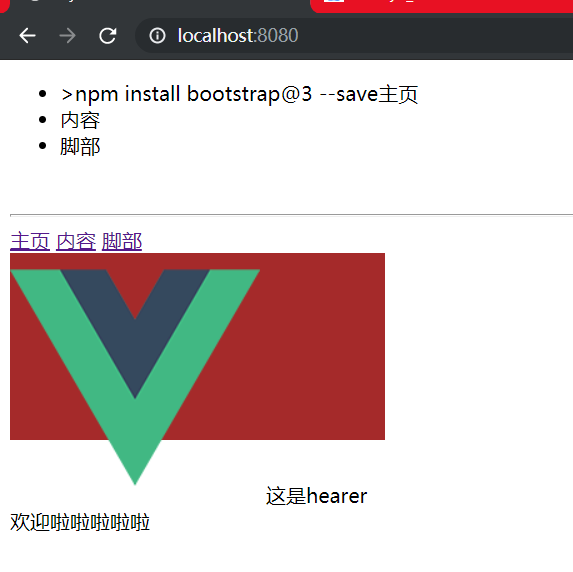
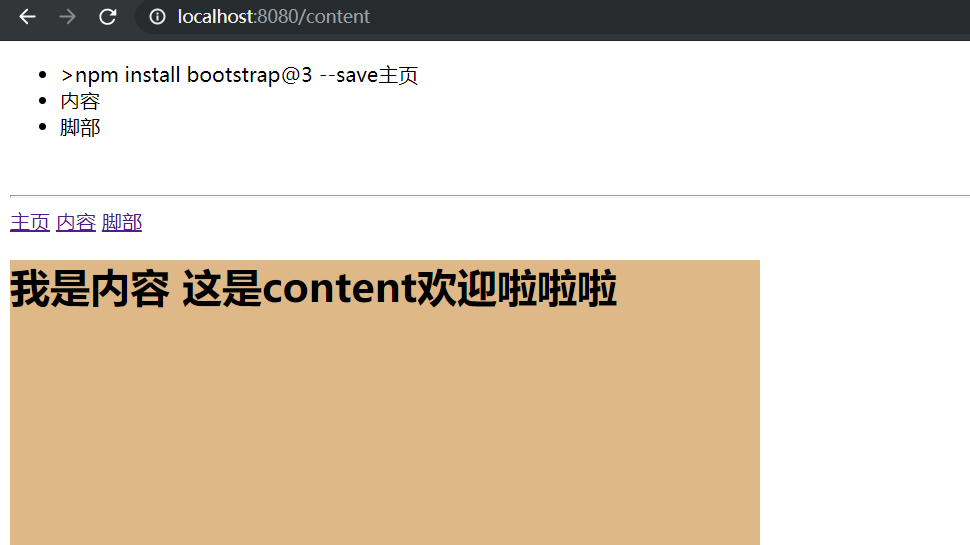
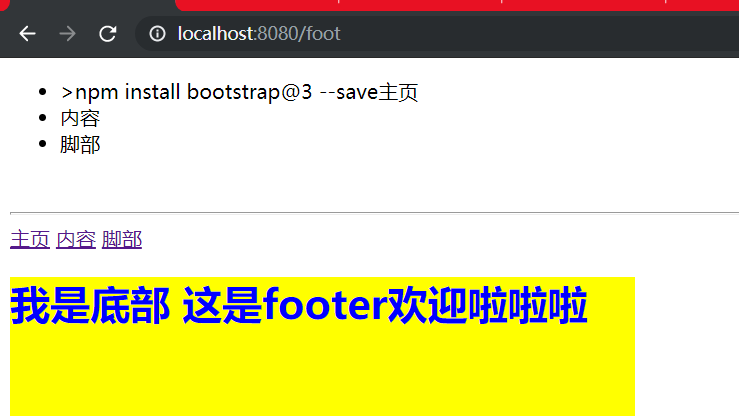