一、中间件
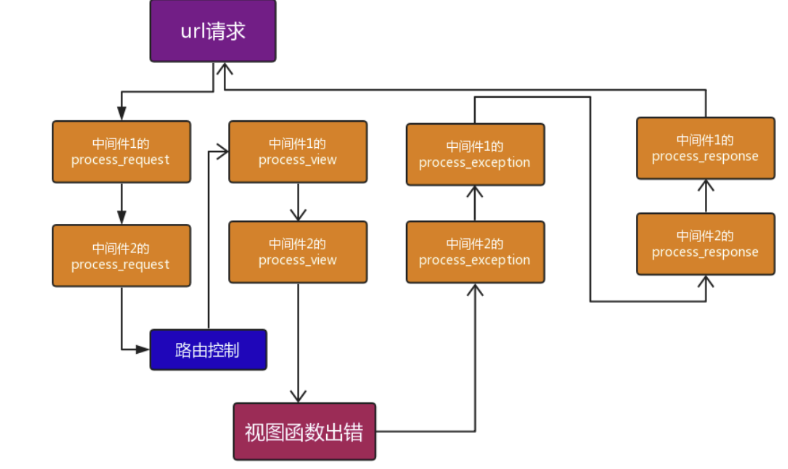
# 中间件可以对请求、响应...等功能完成过滤
#form表单完成csrf认证
<form action="/get_money/" method="post">
{% csrf_token %} #可以通过本域的安全认证
<input type="submit" value="取钱">
</form>
#ajax完成csrf认证
<button class="btn">ajax取钱</button>
<script src="/static/jquery-3.3.1.js"></script>
<script src="/static/jquery.cookie.js"></script>
#tokrn通过data来携带
<script>
token = $.cookie('csrftoken');
$('.btn').click(function () {
$.ajax({
url:'/get_money/',
type:'post',
data:{
'csrfmiddlewaretoken': token
},
success:function (data) {
console.log(data)
}
})
})
</script>
#token通过头来携带
<script>
token = $.cookie('csrftoken');
$('.btn').click(function () {
$.ajax({
url:'/get_money/',
type:'post',
headers:{'X-CSRFToken':token},
success:function (data) {
console.log(data)
}
})
})
</script>
'''
# 自定义中间件
# 1. 在某一应用下(eg:app),建立某一py文件(eg: mymiddleware.py)
# 2. 在文件中自定义类,继承django.utils.deprecation下的MiddlewareMixin类
# 3. 实现要过滤的方法,完成具体的过滤条件
'''
from django.utils.deprecation import MiddlewareMixin
class MyMiddleware1(MiddlewareMixin):
def process_request(self, request):
''' 请求处理
:param request:请求对象
:return: 一般没有返回值,但可以返回HttpResponse对象
'''
def process_view(self, request, callback, callback_args, callback_kwargs):
''' 视图函数预处理
:param request: 请求对象
:param callback: 路由返回的视图函数地址
:param callback_args: 视图函数的位置参数(元组)
:param callback_kwargs: 视图函数的关键字参数(字典)
:return: 一般没有返回值,但可以返回HttpResponse对象
'''
def process_exception(self, request, exception):
''' 视图函数异常处理
:param request: 请求对象
:param exception: 视图函数的异常对象
:return: 一般没有返回值,但可以返回HttpResponse对象
'''
def process_template_response(self, request, response):
''' 视图函数返回值为拥有render方法的对象,该方法会执行
:param request: 请求对象
:param response: 响应对象
:return: 一定要返回response
'''
return response
def process_response(self, request, response):
''' 响应处理
:param request: 请求对象
:param response: 响应对象
:return: 一定要返回response
'''
return response
class MyMiddleware2(MiddlewareMixin):
def process_request(self, request):
pass
#例子:
# 1. 在某一应用下(eg:app),建立某一py文件(eg: mymiddleware.py)
# 2.在mymiddleware.py下完成如下代码
from django.utils.deprecation import MiddlewareMixin
class MyMiddleware1(MiddlewareMixin):
def process_request(self, request):
print('MyMiddleware1 request')
def process_view(self, request, callback, callback_args, callback_kwargs):
pass
def process_exception(self, request, exception):
pass
def process_template_response(self, request, response):
return response
def process_response(self, request, response):
print('MyMiddleware1 response')
return response
class MyMiddleware2(MiddlewareMixin):
def process_request(self, request):
print('MyMiddleware2 request')
def process_response(self, request, response):
print('MyMiddleware2 response')
return response
# 3.在settings中配置中间件
MIDDLEWARE = [
'app.mymiddleware.MyMiddleware1',
'app.mymiddleware.MyMiddleware2',
]
#发送url请求
#先调用中间件中的process_request,顺序按照配置顺序从上往下
#然后到路由层进行路由分发,随后到中间件中的process_view,顺序按照配置顺序从上往下,然后process_view会调用视图函数views
#如果视图函数发生异常会调用中间件中的process_exception,顺序按照配置顺序从下到上处理,没有处理成功会报错
#最后调用中间件中的process_response,顺序按照配置顺序从下到上
#如果MyMiddleware1中的process_request直接return,那么请求会直接从这个中间件向上response,不会经过下面的中间件
#要执行中间件中process_template_response要求视图函数返回值是含有render方法的对象
class TTT:
def render(self):
return HttpResponse('TTT 的render')
def test(request):
return TTT()
二、接口规则
'''
演示:
http://api.map.baidu.com/place/v2/search?ak=6E823f587c95f0148c19993539b99295&output=json&query=%E8%82%AF%E5%BE%B7%E5%9F%BA®ion=%E5%8C%97%E4%BA%AC
状态码:
SUCCESS("0000", "查询成功"),
NODATA("0001", "查询成功无记录"),
FEAILED("0002", "查询失败"),
ACCOUNT_ERROR("1000", "账户不存在或被禁用"),
API_NOT_EXISTS("1001", "请求的接口不存在"),
API_NOT_PER("1002", "没有该接口的访问权限"),
PARAMS_ERROR("1004", "参数为空或格式错误"),
SIGN_ERROR("1005", "数据签名错误")
UNKNOWN_IP("1099", "非法IP请求"),
SYSTEM_ERROR("9999", "系统异常");
规范制定:
{
'statue': 0,
'msg': 'ok',
'data': {}
}
0:成功,1:成功没结果,2:失败
'''