1. Object.prototype.toString.call()
每一个继承 Object 的对象都有
toString
方法,如果 toString
方法没有重写的话,会返回 [Object type]
,其中 type 为对象的类型。但当除了 Object 类型的对象外,其他类型直接使用 toString
方法时,会直接返回都是内容的字符串,所以我们需要使用call或者apply方法来改变toString方法的执行上下文const an = ['Hello','An']; an.toString(); // "Hello,An" Object.prototype.toString.call(an); // "[object Array]"
优点:这种方法对于所有基本的数据类型都能进行判断,即使是 null和defined,且和下面的Array.isArray方法一样都
检测出 iframes
;
Object.prototype.toString.call('An') // "[object String]" Object.prototype.toString.call(1) // "[object Number]" Object.prototype.toString.call(Symbol(1)) // "[object Symbol]" Object.prototype.toString.call(null) // "[object Null]" Object.prototype.toString.call(undefined) // "[object Undefined]" Object.prototype.toString.call(function(){}) // "[object Function]" Object.prototype.toString.call({name: 'An'}) // "[object Object]"
缺点:不能精准判断自定义对象,对于自定义对象只会返回[object Object]
function f(name) { this.name=name; } var f1=new f('martin'); console.log(Object.prototype.toString.call(f1));//[object Object]
Object.prototype.toString.call()
常用于判断浏览器内置对象。
2. instanceof
instanceof
的内部机制是通过判断对象的原型链中是不是能找到类型的 prototype
。
使用 instanceof
判断一个对象是否为数组,instanceof
会判断这个对象的原型链上是否会找到对应的 Array
的原型,找到返回 true
,否则返回 false
[] instanceof Array; // true
缺点: instanceof
只能用来判断对象类型,原始类型不可以。并且所有对象类型 instanceof Object 都是 true,且不同于其他两种方法的是它不能检测出iframes
。
优点:instanceof可以弥补Object.prototype.toString.call()不能判断自定义实例化对象的缺点。
function f(name) { this.name=name; } var f1=new f('martin'); console.log(f1 instanceof f);//true
3.Array.isArray()
-
功能:用来判断对象是否为数组
-
instanceof 与 isArray
优点:当检测Array实例时,
Array.isArray
优于instanceof
,因为Array.isArray
和Object.prototype.toString.call可以检测出iframes,而instanceof不能
var iframe = document.createElement('iframe'); document.body.appendChild(iframe); xArray = window.frames[window.frames.length-1].Array; var arr = new xArray(1,2,3); // [1,2,3] // Correctly checking for Array Array.isArray(arr); // true Object.prototype.toString.call(arr); // true // Considered harmful, because doesn't work though iframes arr instanceof Array; // false
缺点:只能判别数组
Array.isArray()
与Object.prototype.toString.call()
Array.isArray()
是ES5新增的方法,当不存在 Array.isArray()
,可以用 Object.prototype.toString.call()
实现。
if (!Array.isArray) { Array.isArray = function(arg) { return Object.prototype.toString.call(arg) === '[object Array]'; }; }
总结:
方法 | Array.isArray | instanceof | Object.prototype.toString.call |
检测数据类型 | 数组对象 | 对象(包括自定义实例化对象)和所有基本类型 | 对象(不包括自定义实例化对象)和所有基本类型 |
能否检测iframes | 能 | 不能 | 能 |
[-_-]眼睛累了吧,注意劳逸结合呀[-_-]
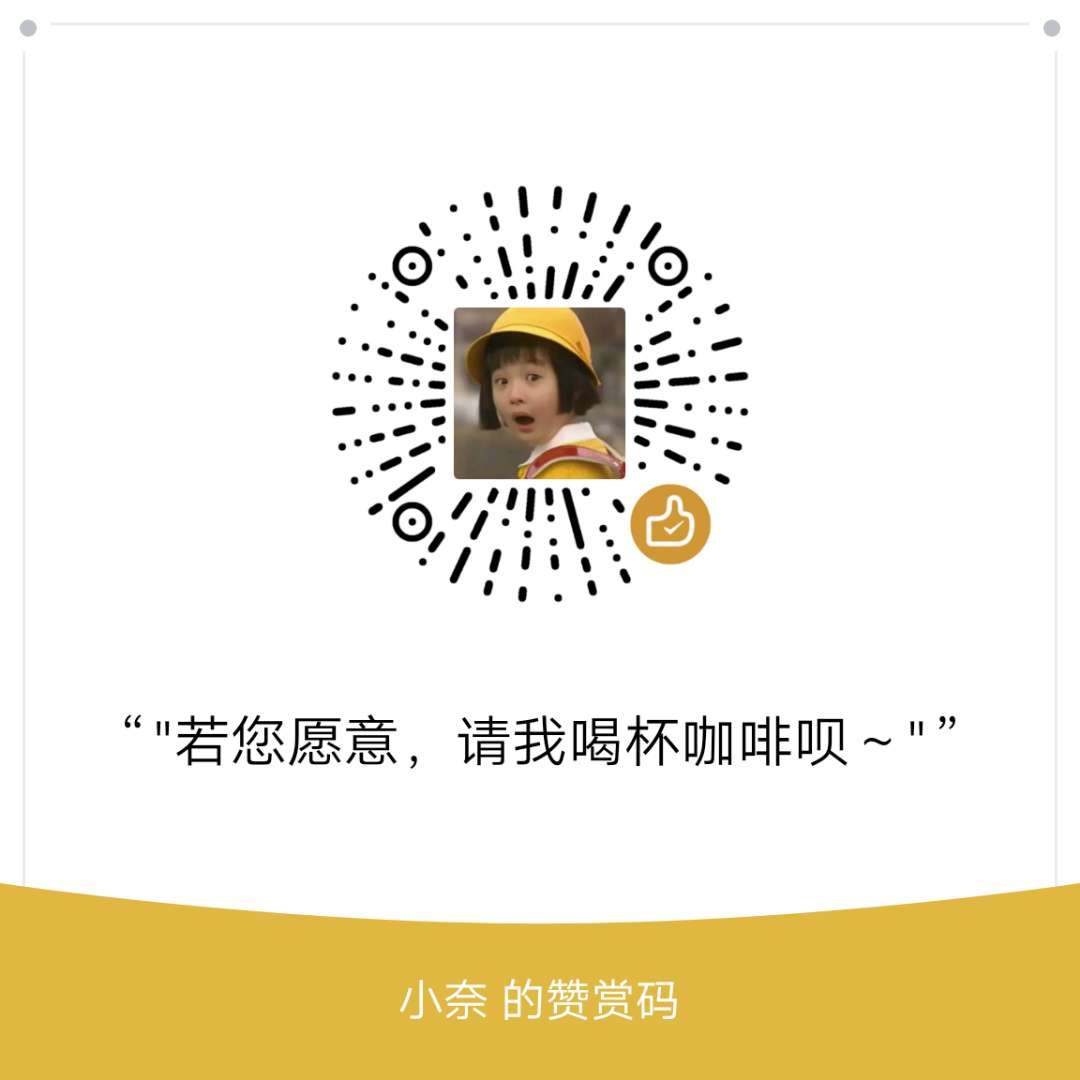