一、难点突破
1 知识梳理
二、综合练习
1 阶段1:练习——分析业务,创建用户类
1.1 需求说明
-
分析业务,抽象出类、类的特征和行为
-
创建用户类
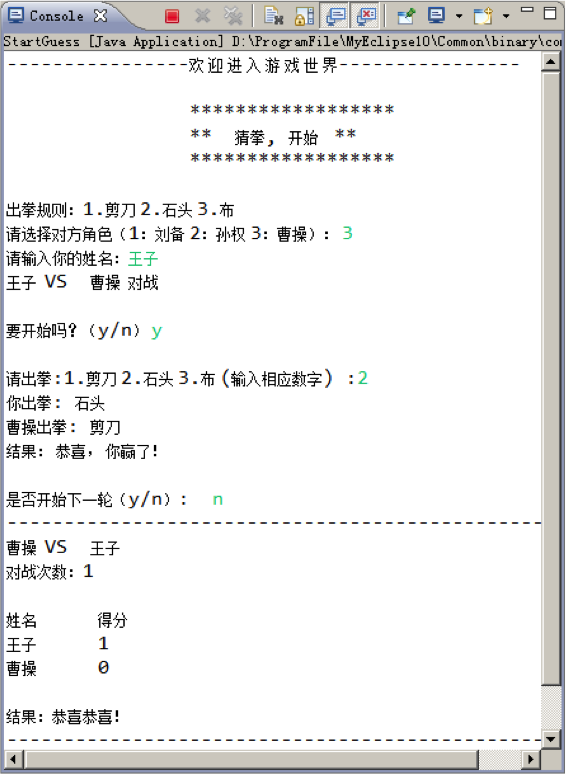
1.2 分析
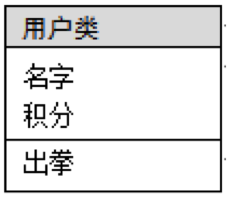
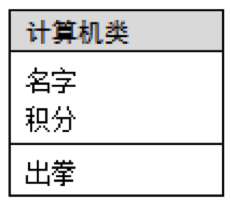
1.3 代码
-
创建用户:定义用户类Person,定义类的属性(name、score)和类的方法(showFirst())
/**
*
*
*
*/
public class Person {
String name = "匿名"; // 名字
int score = 0; // 积分
/**
* 出拳
*
*
*/
public int showFist() {
// 接收用户的选择
Scanner input = new Scanner(System.in);
System.out.print(" 请出拳:1.剪刀 2.石头 3.布 (输入相应数字) :");
int show = input.nextInt();
// 输出出拳结果,并返回
switch (show) {
case 1:
System.out.println("你出拳: 剪刀");
break;
case 2:
System.out.println("你出拳: 石头");
break;
case 3:
System.out.println("你出拳: 布");
break;
}
return show;
}
}
- 测试用户出拳
/**
* 人机互动版猜拳游戏
* 阶段1:测试用户出拳
*
*/
public class TestPerson {
public static void main(String[] args) {
Person person = new Person();
System.out.println(person.showFist());
}
}
2 阶段2:练习——创建计算机类
2.1 需求说明
创建计算机类Computer。实现计算机出拳2.2 分析
产生一个1~3的随机数,模拟计算机的出拳结果,例如,产生2,显示“电脑出拳:石头”2.3 代码
- 计算机类
/**
* 计算机类
* 阶段2完成
*/
public class Computer {
String name = "电脑"; // 名字
int score = 0;; // 积分
/**
* 出拳
*
*/
public int showFist(){
// 产生随机数
int show = (int)(Math.random()*10)%3 + 1; //产生随机数,表示电脑出拳
// 输出出拳结果并返回
switch(show){
case 1:
System.out.println(name+"出拳: 剪刀");
break;
case 2:
System.out.println(name+"出拳: 石头");
break;
case 3:
System.out.println(name+"出拳: 布");
break;
}
return show;
}
}
- 测试计算机类
/**
* 人机互动版猜拳游戏
* 阶段2:测试电脑出拳
*/
public class TestComputer {
public static void main(String[] args) {
Computer computer = new Computer();
System.out.println(computer.showFist());
}
}
3 阶段3 练习——创建游戏类,选择对战对手
3.1 需求说明
- 创建游戏类Game
- 编写游戏类的初始化方法initial()
- 编写游戏类的开始游戏方法startGame()编写游戏类的开始游戏方法startGame()
3.2 分析
3.3 代码
- 游戏类
/**
* 游戏类
*/
public class Game1 {
Person person; //甲方
Computer computer; //乙方
int count; //对战次数
/**
* 初始化
*/
public void initial(){
person = new Person();
computer = new Computer();
count = 0;
}
/**
* 开始游戏
*/
public void startGame() {
initial();
System.out.println("----------------欢 迎 进 入 游 戏 世 界---------------- ");
System.out.println(" ******************");
System.out.println (" ** 猜拳, 开始 **");
System.out.println (" ******************");
System.out.println(" 出拳规则:1.剪刀 2.石头 3.布");
/*选择对方角色*/
System.out.print("请选择对方角色(1:刘备 2:孙权 3:曹操): ");
Scanner input = new Scanner(System.in);
int role = input.nextInt();
if(role == 1){
computer.name = "刘备";
}else if(role == 2){
computer.name = "孙权";
}else if(role == 3){
computer.name = "曹操";
}
System.out.print("你选择了"+computer.name+"对战");
}
}
- 测试开始游戏:选择对战角色
/**
* 人机互动版猜拳游戏
* 阶段3:测试开始游戏:选择对战角色
*/
public class TestGame1 {
public static void main(String[] args) {
Game1 game = new Game1();
game.startGame();
}
}
4 阶段4:练习——实现一局对战
4.1 需求说明分别调用用户类和计算机类的出拳方法showFist(),接受返回值并比较,给出胜负结果
4.2 分析
4.3 代码- 实现一局对战
/**
* 游戏类
* 阶段4:实现一局对战
*/
public class Game2 {
Person person; //甲方
Computer computer; //乙方
int count; //对战次数
/**
* 初始化
*/
public void initial(){
person = new Person();
computer = new Computer();
count = 0;
}
/**
* 开始游戏
*/
public void startGame() {
initial(); // 初始化
System.out.println("----------------欢 迎 进 入 游 戏 世 界---------------- ");
System.out.println(" ******************");
System.out.println (" ** 猜拳, 开始 **");
System.out.println (" ******************");
System.out.println(" 出拳规则:1.剪刀 2.石头 3.布");
/*选择对方角色*/
System.out.print("请选择对方角色(1:刘备 2:孙权 3:曹操): ");
Scanner input = new Scanner(System.in);
int role = input.nextInt();
if(role == 1){
computer.name = "刘备";
}else if(role == 2){
computer.name = "孙权";
}else if(role == 3){
computer.name = "曹操";
}
System.out.println("你选择了 "+computer.name+"对战");
/*开始游戏*/
System.out.print(" 要开始吗?(y/n) ");
String con = input.next();
int perFist; //用户出的拳
int compFist; //计算机出的拳
if(con.equals("y")){
/*出拳*/
perFist = person.showFist();
compFist = computer.showFist();
/*裁决*/
if((perFist == 1 && compFist == 1) || (perFist == 2 && compFist == 2) || (perFist == 3 && compFist == 3)){
System.out.println("结果:和局,真衰! "); //平局
}else if((perFist == 1 && compFist == 3) || (perFist == 2 && compFist == 1) || (perFist == 3 && compFist == 2)){
System.out.println("结果: 恭喜, 你赢了!"); //用户赢
}else{
System.out.println("结果说:^_^,你输了,真笨! "); //计算机赢
}
}
}
}
- 测试开始游戏:实现1局对战
/**
* 人机互动版猜拳游戏
* 阶段4:测试开始游戏:实现1局对战
*/
public class TestGame2 {
public static void main(String[] args) {
Game2 game = new Game2();
game.startGame();
}
}
5 阶段5:练习——实现循环对战,并累计得分
5.1 需求说明
实现循环对战,
并且累加赢家的得分5.2 分析
5.3 代码- 实现循环对战
/**
* 游戏类
* 阶段5:实现循环对战
*
*/
public class Game3 {
Person person; //甲方
Computer computer; //乙方
int count; //对战次数
/**
* 初始化
*/
public void initial(){
person = new Person();
computer = new Computer();
count = 0;
}
/**
* 开始游戏
*/
public void startGame() {
initial(); // 初始化
System.out.println("----------------欢 迎 进 入 游 戏 世 界---------------- ");
System.out.println(" ******************");
System.out.println (" ** 猜拳, 开始 **");
System.out.println (" ******************");
System.out.println(" 出拳规则:1.剪刀 2.石头 3.布");
/*选择对方角色*/
System.out.print("请选择对方角色(1:刘备 2:孙权 3:曹操): ");
Scanner input = new Scanner(System.in);
int role = input.nextInt();
if(role == 1){
computer.name = "刘备";
}else if(role == 2){
computer.name = "孙权";
}else if(role == 3){
computer.name = "曹操";
}
System.out.println("你选择了 "+computer.name+"对战");
/*开始游戏*/
System.out.print(" 要开始吗?(y/n) ");
String con = input.next();
int perFist; //用户出的拳
int compFist; //计算机出的拳
while(con.equals("y")){
/*出拳*/
perFist = person.showFist();
compFist = computer.showFist();
/*裁决*/
if((perFist == 1 && compFist == 1) || (perFist == 2 && compFist == 2) || (perFist == 3 && compFist == 3)){
System.out.println("结果:和局,真衰!嘿嘿,等着瞧吧 ! "); //平局
}else if((perFist == 1 && compFist == 3) || (perFist == 2 && compFist == 1) || (perFist == 3 && compFist == 2)){
System.out.println("结果: 恭喜, 你赢了!"); //用户赢
person.score++;
}else{
System.out.println("结果说:^_^,你输了,真笨! "); //计算机赢
computer.score++;
}
count++;
System.out.print(" 是否开始下一轮(y/n): ");
con = input.next();
}
}
}
- 测试循环对战
public class TestGame3 {
public static void main(String[] args) {
Game3 game = new Game3();
game.startGame();
}
}
6 阶段6:练习——显示对战结果
6.1 需求说明
游戏结束后,显示对战结果
6.2 分析
编写showResult( )方法,比较二者的得分情况,给出对战结果6.3 代码
- 实现对战结果显示
/**
* 游戏类
* 阶段6:实现对战结果显示
*/
public class Game4 {
Person person; //甲方
Computer computer; //乙方
int count; //对战次数
/**
* 初始化
*/
public void initial(){
person = new Person();
computer = new Computer();
count = 0;
}
/**
* 开始游戏
*/
public void startGame() {
initial(); // 初始化
System.out.println("----------------欢 迎 进 入 游 戏 世 界---------------- ");
System.out.println(" ******************");
System.out.println (" ** 猜拳, 开始 **");
System.out.println (" ******************");
System.out.println(" 出拳规则:1.剪刀 2.石头 3.布");
/*选择对方角色*/
System.out.print("请选择对方角色(1:刘备 2:孙权 3:曹操): ");
Scanner input = new Scanner(System.in);
int role = input.nextInt();
if(role == 1){
computer.name = "刘备";
}else if(role == 2){
computer.name = "孙权";
}else if(role == 3){
computer.name = "曹操";
}
System.out.println("你选择了 "+computer.name+"对战");
System.out.print(" 要开始吗?(y/n) ");
String con = input.next();
int perFist; //用户出的拳
int compFist; //计算机出的拳
while(con.equals("y")){
/*出拳*/
perFist = person.showFist();
compFist = computer.showFist();
/*裁决*/
if((perFist == 1 && compFist == 1) || (perFist == 2 && compFist == 2) || (perFist == 3 && compFist == 3)){
System.out.println("结果:和局,真衰!嘿嘿,等着瞧吧 ! "); //平局
}else if((perFist == 1 && compFist == 3) || (perFist == 2 && compFist == 1) || (perFist == 3 && compFist == 2)){
System.out.println("结果: 恭喜, 你赢了!"); //用户赢
person.score++;
}else{
System.out.println("结果说:^_^,你输了,真笨! "); //计算机赢
computer.score++;
}
count++;
System.out.print(" 是否开始下一轮(y/n): ");
con = input.next();
}
/*显示结果*/
showResult();
}
/**
* 显示比赛结果
*/
public void showResult(){
/*显示最后结果*/
System.out.println("---------------------------------------------------");
System.out.println(computer.name + " VS " + person.name);
System.out.println("对战次数:"+ count);
int result = calcResult();
if(result == 1){
System.out.println("结果:打成平手,下次再和你一分高下!");
}else if(result == 2){
System.out.println("结果:恭喜恭喜!"); //用户获胜
}else{
System.out.println("结果:呵呵,笨笨,下次加油啊!"); //计算机获胜
}
System.out.println("---------------------------------------------------");
}
/**
* 计算比赛结果
*
*/
public int calcResult(){
if(person.score == computer.score){
return 1; // 战平
}else if(person.score > computer.score){
return 2; // 用户赢
}else{
return 3; // 电脑赢
}
}
}
- 测试
public class TestGame4 {
/**
* 人机互动版猜拳游戏
*/
public static void main(String[] args) {
Game4 game = new Game4();
game.initial();
game.startGame();
}
}
7 阶段7:练习——完善游戏类的startGame()
7.1 需求说明输入并保存用户姓名,游戏结束后显示双方的各自得分
7.2 分析
7.3 代码- 功能扩展
public class Game {
Person person; //甲方
Computer computer; //乙方
int count; //对战次数
/**
* 初始化
*/
public void initial(){
person = new Person();
computer = new Computer();
count = 0;
}
/**
* 开始游戏
*/
public void startGame() {
System.out.println("----------------欢 迎 进 入 游 戏 世 界----------------");
System.out.println(" ******************");
System.out.println (" ** 猜拳, 开始 **");
System.out.println (" ******************");
System.out.println(" 出拳规则:1.剪刀 2.石头 3.布");
Scanner input = new Scanner(System.in);
String exit = "n"; // 退出系统
do{
initial(); // 初始化
/*选择对方角色*/
System.out.print("请选择对方角色(1:刘备 2:孙权 3:曹操): ");
int role = input.nextInt();
if(role == 1){
computer.name = "刘备";
}else if(role == 2){
computer.name = "孙权";
}else if(role == 3){
computer.name = "曹操";
}
// 扩展功能1:输入用户姓名
/*输入用户姓名*/
System.out.print("请输入你的姓名:");
person.name = input.next();
System.out.println(person.name+" VS "+computer.name+" 对战 ");
// 扩展功能1结束
System.out.print("要开始吗?(y/n) ");
String start = input.next(); // 开始每一局游戏
int perFist; //用户出的拳
int compFist; //计算机出的拳
while(start.equals("y")){
/*出拳*/
perFist = person.showFist();
compFist = computer.showFist();
/*裁决*/
if((perFist == 1 && compFist == 1) || (perFist == 2 && compFist == 2) || (perFist == 3 && compFist == 3)){
System.out.println("结果:和局,真衰!嘿嘿,等着瞧吧 ! "); //平局
}else if((perFist == 1 && compFist == 3) || (perFist == 2 && compFist == 1) || (perFist == 3 && compFist == 2)){
System.out.println("结果: 恭喜, 你赢了!"); //用户赢
person.score++;
}else{
System.out.println("结果说:^_^,你输了,真笨! "); //计算机赢
computer.score++;
}
count++;
System.out.print(" 是否开始下一轮(y/n): ");
start = input.next();
}
/*显示结果*/
showResult();
// 扩展功能3:循环游戏,直到退出系统
System.out.print(" 要开始下一局吗?(y/n):");
exit = input.next();
System.out.println();
// 扩展功能3结束
}while(!exit.equals("n"));
System.out.println("系统退出!");
}
/**
* 显示比赛结果
*/
public void showResult(){
/*显示对战次数*/
System.out.println("---------------------------------------------------");
System.out.println(computer.name + " VS " + person.name);
System.out.println("对战次数:"+ count);
// 扩展功能2:显示最终的得分
System.out.println(" 姓名 得分");
System.out.println(person.name+" "+person.score);
System.out.println(computer.name+" "+computer.score+" ");
// 扩展功能2结束
/*显示对战结果*/
int result = calcResult();
if(result == 1){
System.out.println("结果:打成平手,下次再和你一分高下!");
}else if(result == 2){
System.out.println("结果:恭喜恭喜!"); //用户获胜
}else{
System.out.println("结果:呵呵,笨笨,下次加油啊!"); //计算机获胜
}
System.out.println("---------------------------------------------------");
}
/**
* 计算比赛结果
*
*/
public int calcResult(){
if(person.score == computer.score){
return 1; // 战平
}else if(person.score > computer.score){
return 2; // 用户赢
}else{
return 3; // 电脑赢
}
}
}
测试/**
* 人机互动版猜拳游戏
* 程序入口
*/
public class StartGuess {
public static void main(String[] args) {
Game game = new Game();
game.startGame();
}
}