#include <windows.h>
#include <iostream.h>
DWORD WINAPI Fun1Proc(
LPVOID lpParameter
);
DWORD WINAPI Fun2Proc(
LPVOID lpParameter
);
int index = 0;
int tickets = 100;
HANDLE hMutex;
void main()
{
HANDLE hThread1;
HANDLE hThread2;
hMutex = CreateMutex(NULL, FALSE, "tickets");
if (hMutex){
DWORD dw = GetLastError();
if (ERROR_ALREADY_EXISTS == dw){
cout<<"only one instance can run!!"<<endl;
return;
}
}
hThread1 = CreateThread(NULL,0,Fun1Proc, NULL, 0, NULL);
hThread2 = CreateThread(NULL,0,Fun2Proc, NULL, 0, NULL);
CloseHandle(hThread1);
CloseHandle(hThread2);
Sleep(4000);
}
DWORD WINAPI Fun1Proc(LPVOID lpParameter ){
while(TRUE){
WaitForSingleObject(hMutex, INFINITE);
if (tickets > 0){
cout<<"thread1 sell ticket:"<<tickets--<<endl;
}else{
break;
}
ReleaseMutex(hMutex);
}
return 0;
}
DWORD WINAPI Fun2Proc(LPVOID lpParameter ){
while(TRUE){
WaitForSingleObject(hMutex, INFINITE);
if (tickets > 0){
cout<<"thread2 sell ticket:"<<tickets--<<endl;
}else{
break;
}
ReleaseMutex(hMutex);
}
return 0;
}
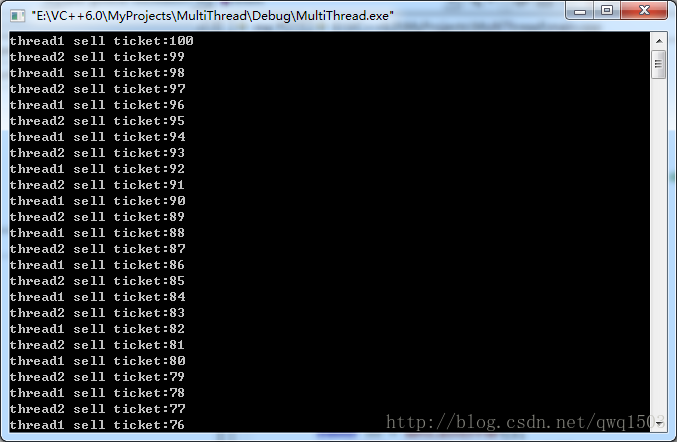