初始效果
点击添加行
点击添加数据
点击打印数据
点击清空数据
TS 部分代码讲解
/**
* 获取数组对象
*/
get arrayList()
{
return this.fg.get('arrayList') as FormArray;
}
获取的 HTML页面的控件arrayList 对象
/**
* 添加数据
*/
addData(){
this.arrayList.controls.forEach((item,index)=>{
//此处的this.arrayList出自 get arrayList()方法获得的FormArray 对象
通过FormArray的controls属性来获取arrayList控件下的组件
angular2源码截图

item.patchValue({
firstName:'zhangsan'+index,
age:20+index,
profession:'java',
})
});
}
关于patchValue的解释参照 angular2的官方文档链接
1.导入模块
import { ReactiveFormsModule } from '@angular/forms'; 红线标记为需要引入代码
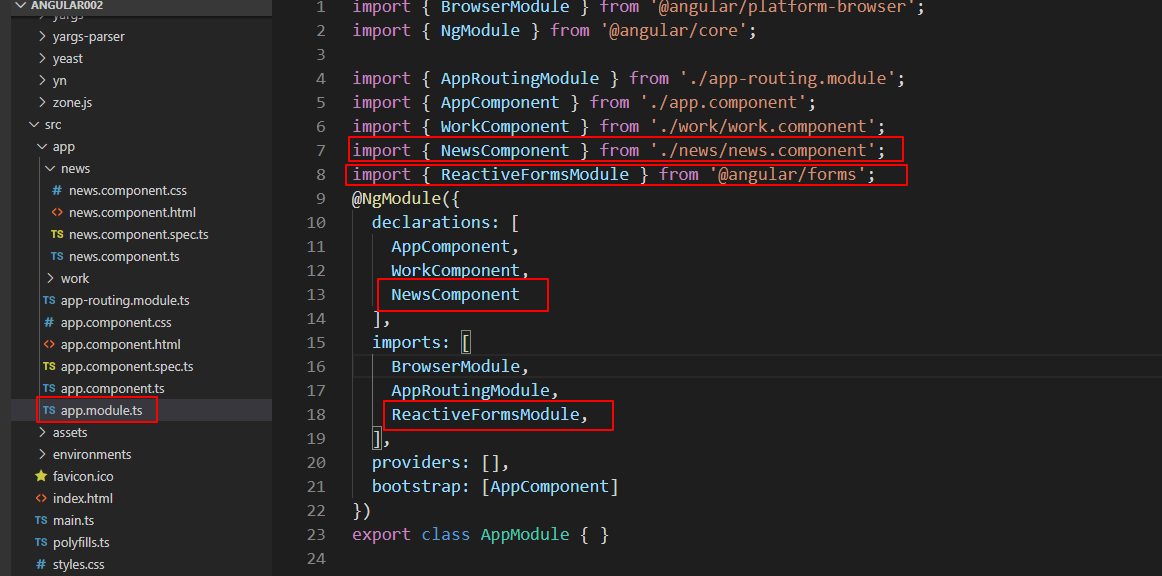
2.HTML 部分
HTML源码
<form [formGroup]="fg" >
<table class="table table-bordered">
<tr><td>姓名</td><td>年龄</td><td>职业</td><td></td></tr>
<ng-container formArrayName='arrayList'>
<ng-container *ngFor="let row of arrayList.controls;let i =index">
<tr>
<ng-container [formGroup]="row">
<td><input type="text" class='form-control' formControlName="firstName"></td>
<td><input type="text"class='form-control' formControlName="age"></td>
<td><input type="text" class='form-control' formControlName="profession"></td>
<td><button class='form-control' (click)="delBtn(i)">删除</button></td>
</ng-container>
</tr>
</ng-container>
</ng-container>
</table>
</form>
<button (click)="addBtn()">添加行</button>
<button (click)="addData()">添加数据</button>
<button (click)="printData()">打印数据</button>
<button (click)="clearData()">清空数据</button>
3.ts部分源码
import { Component } from '@angular/core';
import { FormGroup, FormBuilder ,FormArray } from '@angular/forms';
@Component({
selector: 'app-news',
templateUrl: './news.component.html',
styleUrls: ['./news.component.css']
})
export class NewsComponent {
constructor(private formBuilder: FormBuilder) { }
public fg:FormGroup =this.formBuilder.group(
{
// 创建数组对象
arrayList:this.formBuilder.array([])
}
);
/**
* 获取数组对象
*/
get arrayList()
{
return this.fg.get('arrayList') as FormArray;
}
/**
* 创建一行组件
*/
createRow(){
return this.formBuilder.group({
firstName:[''],
age:[''],
profession:[''],
});
}
/**
* 增加一行事件
*/
addBtn(){
this.arrayList.push(this.createRow());
}
/**
* 删除一行事件
*/
delBtn(i:number){
this.arrayList.removeAt(i);
}
/**
* 添加数据
*/
addData(){
this.arrayList.controls.forEach((item,index)=>{
item.patchValue({
firstName:'zhangsan'+index,
age:20+index,
profession:'java',
})
});
}
/**
* 打印数据
*/
printData(){
console.log(this.fg.get('arrayList').value);
}
/**
* 清空数据
*/
clearData(){
this.arrayList.controls.forEach(item=>{
item.patchValue({
firstName:'',
age:'',
profession:'',
})
})
}
}