构建一个应用,并在docker compose里运行起来
利用python3.7+flask+redis实现一个点击计数器
1.定义一个应用依赖
- 新建一个项目文件夹,并进入项目目录,以下无特殊说明都表示在当前项目目录下的操作
$ mkdir composetest
$ cd composetest
- 编写应用代码app.py
import time
import redis
from flask import Flask
app = Flask(__name__)
cache = redis.Redis(host='redis', port=6379)
def get_hit_count():
retries = 5
while True:
try:
return cache.incr('hits')
except redis.exceptions.ConnectionError as exc:
if retries == 0:
raise exc
retries -= 1
time.sleep(0.5)
@app.route('/')
def hello():
count = get_hit_count()
return 'Hello World! I have been seen {} times.\n'.format(count)
- 创建requirements.txt,并写入flask,redis
flask
redis
2.创建一个Dockerfile
FROM python:3.7-alpine
WORKDIR /code
ENV FLASK_APP=app.py
ENV FLASK_RUN_HOST=0.0.0.0
RUN apk add --no-cache gcc musl-dev linux-headers
COPY requirements.txt requirements.txt
RUN pip install -r requirements.txt
EXPOSE 5000
COPY . .
CMD ["flask", "run"]
This tells Docker to:
- Build an image starting with the Python 3.7 image.
- Set the working directory to
/code
. - Set environment variables used by the
flask
command. - Install gcc and other dependencies
- Copy
requirements.txt
and install the Python dependencies. - Add metadata to the image to describe that the container is listening on port 5000
- Copy the current directory
.
in the project to the workdir.
in the image. - Set the default command for the container to
flask run
.
3.创建docker-compose.yml,定义services,这里定义2个services web 和redis
version: "3.9"
services:
web:
build: .
ports:
- "5000:5000"
redis:
image: "redis:alpine"
web和redis是服务名
ports建立宿主机(第一个5000)的5000端口和容器(第二个5000)的5000端口的映射
4.使用docker-compose构建和运行上面定义的应用
$ docker-compose up
composetest_web_1 is up-to-date
composetest_redis_1 is up-to-date
Attaching to composetest_web_1, composetest_redis_1
web_1 | * Serving Flask app "app.py"
web_1 | * Environment: production
web_1 | WARNING: This is a development server. Do not use it in a production deployment.
web_1 | Use a production WSGI server instead.
web_1 | * Debug mode: off
web_1 | * Running on http://0.0.0.0:5000/ (Press CTRL+C to quit)
redis_1 | 1:C 13 Jan 2021 01:57:55.601 # oO0OoO0OoO0Oo Redis is starting oO0OoO0OoO0Oo
redis_1 | 1:C 13 Jan 2021 01:57:55.601 # Redis version=6.0.9, bits=64, commit=00000000, modified=0, pid=1, just started
redis_1 | 1:C 13 Jan 2021 01:57:55.601 # Warning: no config file specified, using the default config. In order to specify a config file use redis-server /path/to/redis.conf
redis_1 | 1:M 13 Jan 2021 01:57:55.602 * Running mode=standalone, port=6379.
redis_1 | 1:M 13 Jan 2021 01:57:55.602 # WARNING: The TCP backlog setting of 511 cannot be enforced because /proc/sys/net/core/somaxconn is set to the lower value of 128.
redis_1 | 1:M 13 Jan 2021 01:57:55.602 # Server initialized
redis_1 | 1:M 13 Jan 2021 01:57:55.602 # WARNING overcommit_memory is set to 0! Background save may fail under low memory condition. To fix this issue add 'vm.overcommit_memory = 1' to /etc/sysctl.conf and then reboot or run the command 'sysctl vm.overcommit_memory=1' for this to take effect.
redis_1 | 1:M 13 Jan 2021 01:57:55.602 # WARNING you have Transparent Huge Pages (THP) support enabled in your kernel. This will create latency and memory usage issues with Redis. To fix this issue run the command 'echo madvise > /sys/kernel/mm/transparent_hugepage/enabled' as root, and add it to your /etc/rc.local in order to retain the setting after a reboot. Redis must be restarted after THP is disabled (set to 'madvise' or 'never').
redis_1 | 1:M 13 Jan 2021 01:57:55.603 * Ready to accept connections
现在通过curl 测试一下我们的app
[root@kpc ~]# curl http://0.0.0.0:5000/
Hello World! I have been seen 1 times.
[root@kpc ~]# curl http://0.0.0.0:5000/
Hello World! I have been seen 2 times.
当然浏览器上访问也是一样的
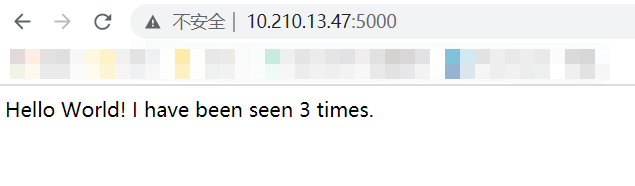
再看一下web服务的日志,可以看到如下2次请求
web_1 | xx.18.0.1 - - [13/Jan/2021 02:21:41] "GET / HTTP/1.1" 200 -
web_1 | xx.18.0.1 - - [13/Jan/2021 02:21:48] "GET / HTTP/1.1" 200 -
说明我们的应用构建并运行的很健康。
列出本地images
$ docker image ls
REPOSITORY TAG IMAGE ID CREATED SIZE
composetest_web latest e2c21aa48cc1 4 minutes ago 93.8MB
python 3.4-alpine 84e6077c7ab6 7 days ago 82.5MB
redis alpine 9d8fa9aa0e5b 3 weeks ago 27.5MB
5.在docker-compose.yml给web挂载一个卷,
version: "3.9"
services:
web:
build: .
ports:
- "5000:5000"
volumes:
- .:/code
environment:
FLASK_ENV: development
redis:
image: "redis:alpine"
6.重新构建运行app
$ docker-compose up
Recreating composetest_web_1 ...
Recreating composetest_web_1 ... done
Attaching to composetest_redis_1, composetest_web_1
redis_1 | 1:C 13 Jan 2021 01:57:55.601 # oO0OoO0OoO0Oo Redis is starting oO0OoO0OoO0Oo
redis_1 | 1:C 13 Jan 2021 01:57:55.601 # Redis version=6.0.9, bits=64, commit=00000000, modified=0, pid=1, just started
redis_1 | 1:C 13 Jan 2021 01:57:55.601 # Warning: no config file specified, using the default config. In order to specify a config file use redis-server /path/to/redis.conf
redis_1 | 1:M 13 Jan 2021 01:57:55.602 * Running mode=standalone, port=6379.
redis_1 | 1:M 13 Jan 2021 01:57:55.602 # WARNING: The TCP backlog setting of 511 cannot be enforced because /proc/sys/net/core/somaxconn is set to the lower value of 128.
redis_1 | 1:M 13 Jan 2021 01:57:55.602 # Server initialized
redis_1 | 1:M 13 Jan 2021 01:57:55.602 # WARNING overcommit_memory is set to 0! Background save may fail under low memory condition. To fix this issue add 'vm.overcommit_memory = 1' to /etc/sysctl.conf and then reboot or run the command 'sysctl vm.overcommit_memory=1' for this to take effect.
redis_1 | 1:M 13 Jan 2021 01:57:55.602 # WARNING you have Transparent Huge Pages (THP) support enabled in your kernel. This will create latency and memory usage issues with Redis. To fix this issue run the command 'echo madvise > /sys/kernel/mm/transparent_hugepage/enabled' as root, and add it to your /etc/rc.local in order to retain the setting after a reboot. Redis must be restarted after THP is disabled (set to 'madvise' or 'never').
redis_1 | 1:M 13 Jan 2021 01:57:55.603 * Ready to accept connections
redis_1 | 1:M 13 Jan 2021 02:57:56.068 * 1 changes in 3600 seconds. Saving...
redis_1 | 1:M 13 Jan 2021 02:57:56.071 * Background saving started by pid 14
redis_1 | 14:C 13 Jan 2021 02:57:56.077 * DB saved on disk
redis_1 | 14:C 13 Jan 2021 02:57:56.078 * RDB: 4 MB of memory used by copy-on-write
redis_1 | 1:M 13 Jan 2021 02:57:56.174 * Background saving terminated with success
web_1 | * Serving Flask app "app.py" (lazy loading)
web_1 | * Environment: development
web_1 | * Debug mode: on
web_1 | * Running on http://0.0.0.0:5000/ (Press CTRL+C to quit)
web_1 | * Restarting with stat
web_1 | * Debugger is active!
web_1 | * Debugger PIN: 712-138-018
访问一下,检查hello world 消息
$ curl http://0.0.0.0:5000/
Hello World! I have been seen 4 times.
$ curl http://0.0.0.0:5000/
Hello World! I have been seen 5 times.
$ curl http://0.0.0.0:5000/
Hello World! I have been seen 6 times.
7.更新一下app.py
return 'Hello from Docker! I have been seen {} times.\n'.format(count)
再访问一下
$ curl http://0.0.0.0:5000/
Hello World! I have been seen 10 times.