一、具体分工(2分)
>###何佳琳主要负责图片按钮界面设计,我负责代码实现。
二、PSP表格(2分)
PSP2.1 |
Personal Software Process Stages |
预估耗时(分钟) |
实际耗时(分钟) |
Planning |
计划 |
50 |
50 |
Estimate |
估计这个任务需要多少时间 |
50 |
50 |
Development |
**开发 ** |
2230 |
2735 |
Analysis |
需求分析 (包括学习新技术) |
700 |
800 |
Design Spec |
生成设计文档 |
50 |
50 |
Design Review |
设计复审 |
30 |
30 |
Coding Standard |
代码规范 (为目前的开发制定合适的规范) |
50 |
55 |
Design |
具体设计 |
300 |
450 |
Coding |
具体编码 |
800 |
900 |
Code Review |
代码复审 |
100 |
150 |
Test |
测试(自我测试,修改代码,提交修改) |
200 |
300 |
Reporting |
报告 |
140 |
150 |
Test Repor |
测试报告 |
50 |
60 |
Size Measurement |
计算工作量 |
30 |
30 |
Postmortem & Process Improvement Plan |
事后总结, 并提出过程改进计划 |
60 |
60 |
|
合计 |
2420 |
2935 |
三、解题思路描述与设计实现说明(15分)
>* ###网络接口的使用(3分)
因为用的是vue算法,所以接口选择了axios。一部分实现的代码如下:
```
import axios from 'axios'
...
axios.interceptors.request.use(
config => {
if (
config.url === 'https://api.shisanshui.rtxux.xyz/auth/login' ||
config.url === 'https://api.shisanshui.rtxux.xyz/auth/login'
) {
} else {
if (localStorage.getItem('Authorization')) {
config.headers['X-Auth-Token'] = localStorage.getItem('Authorization')
}
}
return config
},
error => {
return Promise.reject(error)
}
)
```
>* ###代码组织与内部实现设计(类图)(6分)
| 主要页面 | 功能实现 | 文件名 | 功能实现 |
| :------: | :------: | :------: | :------: |
| login | 登录界面 |build | 构建脚本 |
| startinterface | 开始界面 | config | 实现配置 |
|history | 历史记录界面 | node_moudles | nodejs依赖包 |
|ranking | 排名总榜界面 | src | 图片、源码 |
|play | 发牌界面 | package.json | 描述需要下载的部分 |
###内部按钮位置设计代码:
```
```
###利用分牌函数以及组牌函数来实现前中后墩的牌型:
```
setpokers();
getpokers()
firstdun();
seconddun();
thirddun();
```
###按钮页面跳转部分代码:
```
methods: {
skipranking(){
this.$router.push({path:'/ranking'});
},
skiphistory(){
this.$router.push({path:'/history'});
}
```
因为我们没有用到类,所以没有类图,以上部分即重要组织文件和内部实现设计。
>* ###说明算法的关键与关键实现部分流程图(6分)
###算法的关键应该就是选择最优牌型,特殊牌型先不管的情况下,我们需要把所有可能的情形遍历一遍,这个实现结果真滴很难,所以我们的算法就实现得比较浅显,肯定没办法达到最优策略。
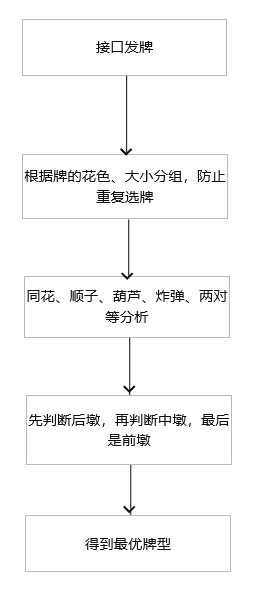
四、关键代码解释(3分)
###接口部分,请求token认证:
```
axios.interceptors.response.use(
response => {
return response
},
error => {
if (error.response) {
switch (error.response.status) {
case 401:
localStorage.removeItem('Authorization')
this.$router.push('/')
}
}
}
)
router.beforeEach((to, from, next) => {
if (to.path === '/') {
next()
} else {
let token = localStorage.getItem('Authorization')
console.log('本地缓存token: ' + token)
if (token === 'null' || token === '') {
next('/')
} else {
next()
}
}
})
```
###分牌部分,通过接口来分发扑克,不重复:
```
setpokers(){
for (var i = this.pokers_Degree.length - 1; i > 0; i--) {
for (var j = 0; j < i; j++) {
if (this.pokers_Degree[j] < this.pokers_Degree[j + 1]) {
[this.pokers_Degree[j], this.pokers_Degree[j + 1]] = [this.pokers_Degree[j + 1], this.pokers_Degree[j]];
[this.pokers_Total[j], this.pokers_Total[j + 1]] = [this.pokers_Total[j + 1], this.pokers_Total[j]];
[this.pokers_Type[j], this.pokers_Type[j + 1]] = [this.pokers_Type[j + 1], this.pokers_Type[j]];
}
}
}
}
```
###组牌部分,把各类牌型牌型的函数从methodd{}中引入并遍历,选出合适的牌型:
```
getpokers(){
let _this=this;
let userid;
axios.post('https://api.shisanshui.rtxux.xyz/game/open', {})
.then(function (response) {
console.log(response.data.data.poker)
_this.poker=response.data.data.poker
userid=response.data.data.userid
})
.catch(function (error) {
console.log(error);
})
.then(function(data){
console.log(_this.poker)
_this.pokers_Degree=[]
_this.pokers_Use=[];
_this.pokers_Total=[]
_this.pokers_Type=[]
_this.pokers_Number=[0,0,0,0,0,0,0,0,0,0,0,0,0,0,0],
_this.pokerstypenum=[0,0,0,0]
_this.firetdun=["","",""]
_this.seconddun=[]
_this.thirddun=["","","","",""]
_this.setpokers();
_this.decidethirddun();
_this.decideseconddun();
_this.decidefirstdun();
_this.getpokers()
})
.then(function(data){
axios.post('https://api.shisanshui.rtxux.xyz/game/submit', {
userid:userid,
poker: _this.sendpokers
})
.then(function (response) {
console.log(response)
})
.catch(function (error) {
console.log(error);
})
})
}
```
五、性能分析与改进(6分)
>* ###我的改进思路
考虑特殊牌型
尝试得到最优解
从整体来考虑获胜的范围,不要执着于炸弹和葫芦这种需要运气成分才能得到的牌
>* ###性能分析图和程序中消耗最大的函数
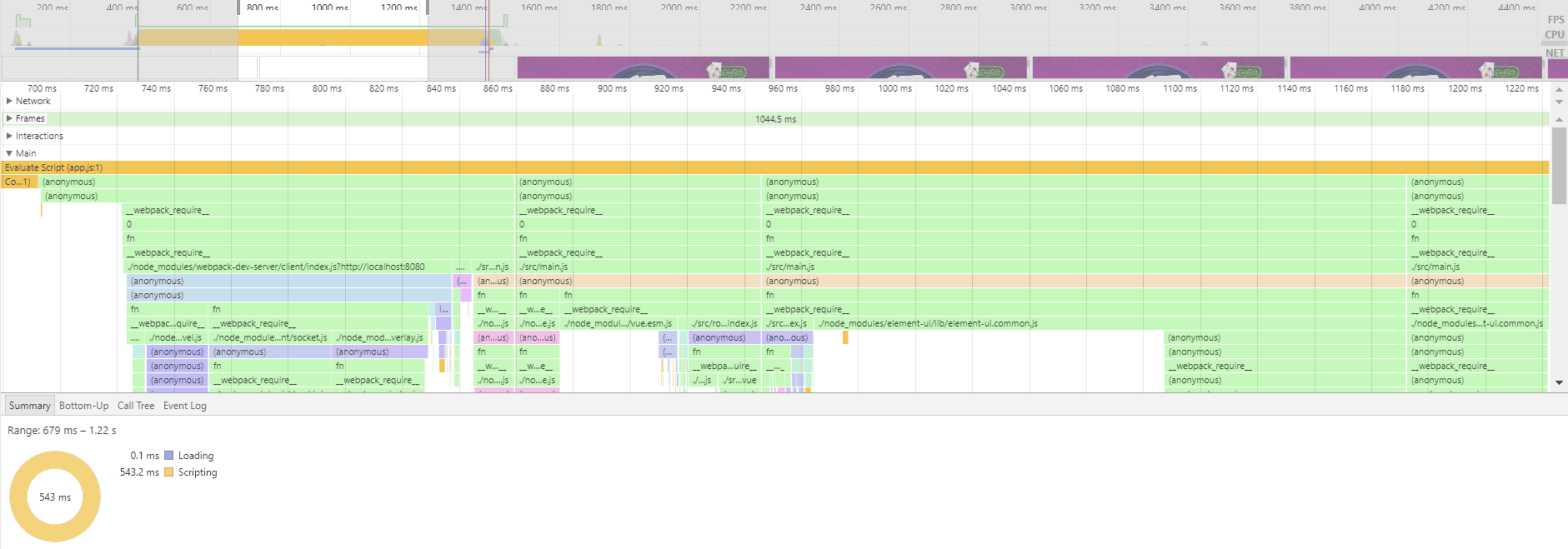
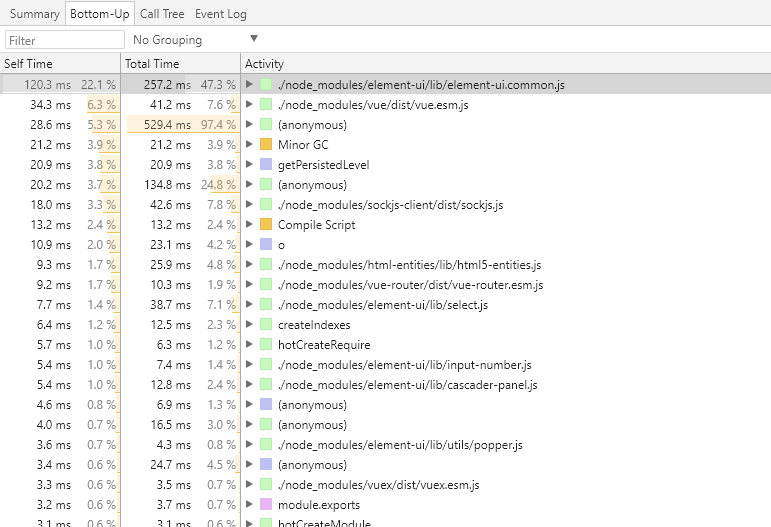
###消耗最大的函数是分牌getpokers()和setpokers(),因为是分牌组牌核心。
六、单元测试(5分)
测试用户登录界面
```
import Vue from 'vue'
import login from '@/components/login'
describe('login.vue', () => {
it('should render correct contents', () => {
const Constructor = Vue.extend(login)
const mytest = new Constructor().$mount()
expect(mytest.$el.querySelector('.bg .username').textContent)
.toEqual('用户名')
expect(mytest.$el.querySelector('.bg .password').textContent)
.toEqual('密码')
})
})
```
七、贴出Github的代码签入记录(1分)
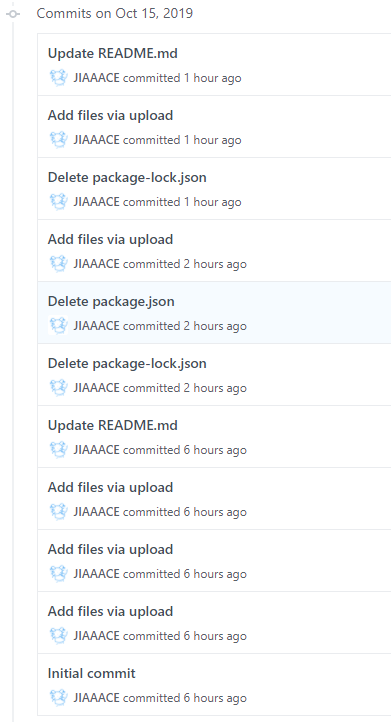
八、遇到的代码模块异常或结对困难及解决方法(8分)
>* ###问题描述(2分)
>####①因为前端没有办法做到网页自适应,所以这次的网页做出来一直是在360浏览器的版本,可能放到别的浏览器就会发现按钮偏了或者界面偏了的问题。
>####②表单table没办法换颜色
>####③一直找不到比较优秀的算法来实现牌型组合
>* ###做过的尝试(2分)
>####1.前端学习的时候我们就想用html,css,js来写,但是我们对前端都不太了解,就只能硬着头皮学下去啦,边写边用搜索引擎。
>####2.因为前段选择完以后,后端如果用vue的话配合度会大一点,所以我们后端选择用vue,仍然是从头开始学。
>####3.居中、全局、覆盖都试过了,图片像素也改了很多遍,可是就是没有办法适应网页,希望看的人多包涵。
>####4.我们发现手动缩放网页的话,界面就可以调整了,所以现在还不能解决的就是网页自动缩放,但是视觉上的问题已经解决了。
>####5.改背景来适应白色的表单。
>####6.vue想实现优秀的算法感觉还是没有java和python方便,但是我们在尝试。
>* ###是否解决(2分)
>####大体上是解决了吧,就是网页不能自适应有点憨
>* ###有何收获(2分)
>**真的要善用搜索引擎!还有很多东西真的要上手写才会熟能生巧,学习的目的还是实践,我们在学习中实践,在实践中学习。**
九、评价你的队友(4分)
>###我队友
值得学习的地方:ps大佬,想要的图告诉她分分钟搞定,创作能力一级棒
值得改进的地方:我的队友温柔体贴,聪明伶俐,没啥要改进的
十、学习进度条(2分)
第N周 |
新增代码(行) |
累计代码(行) |
本周学习耗时(小时) |
累计学习耗时(小时) |
重要成长 |
1 |
100+ |
100+ |
6 |
6 |
学习了Axure RP的用法 |
| 2 | 300+ | 400+ | 8 |14 | 学习了js |
|3 | 1000+ | 1400+ | 30 |44|学习了vue,css,html,js的基本用法|
|4 | | | | ||
十一、游戏界面
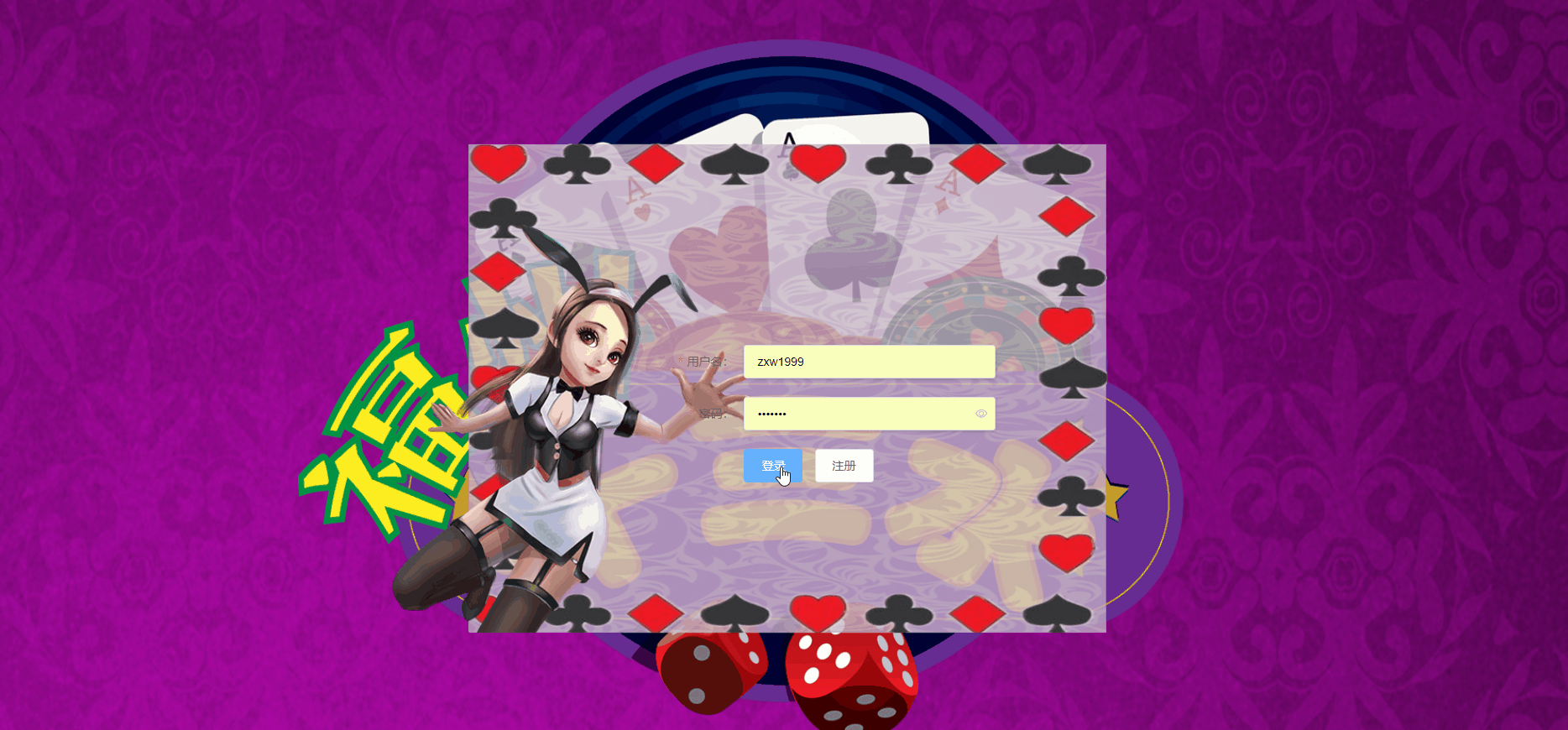