Gold Transportation
Time Limit: 2000MS | Memory Limit: 65536K | |
Total Submissions: 2407 | Accepted: 858 |
Description
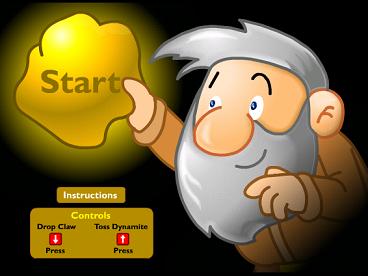
Input
The input contains several test cases. For each case, the first line is integer N(1<=N<=200). The second line is N integers associated with the storage of the gold mine in every towns .The third line is also N integers associated with the storage of the storehouses in every towns .Next is integer M(0<=M<=(n-1)*n/2).Then M lines follow. Each line is three integers x y and d(1<=x,y<=N,0<d<=10000), means that there is a road between x and y for distance of d. N=0 means end of the input.
Output
For each case, output the minimum of the maximum adjacent distance on the condition that all the gold has been transported to the storehouses or "No Solution".
Sample Input
4 3 2 0 0 0 0 3 3 6 1 2 4 1 3 10 1 4 12 2 3 6 2 4 8 3 4 5 0
Sample Output
6
Source
题意:有N座town 每座town都有一定数量gold和仓库 仓库的容量是有限的 有M条双向路径 求把所有的gold 运到仓库最小的最大距离是多少。
思路:跟前几天做的那道poj 2112 Optimal Milking 的思想是一样的,建图时,超级源点与gold相连 容量为gold的数量,超级汇点与仓库相连,容量为仓库的容量,其余边为无穷。用二分枚举求出最小的最距离
思路:跟前几天做的那道poj 2112 Optimal Milking 的思想是一样的,建图时,超级源点与gold相连 容量为gold的数量,超级汇点与仓库相连,容量为仓库的容量,其余边为无穷。用二分枚举求出最小的最距离
#include<iostream> #include<cstdio> #include<cstring> #include<queue> using namespace std; const int VM=220; const int EM=50010; const int INF=0x3f3f3f3f; int n,m,src,des,map[VM][VM],dis[VM][VM]; int total,gold[VM],store[VM],dep[VM]; void buildgraph(int x){ memset(map,0,sizeof(map)); for(int i=1;i<=n;i++) for(int j=1;j<=n;j++) if(dis[i][j]<=x) map[i][j]=INF; for(int i=1;i<=n;i++){ map[src][i]=gold[i]; map[i][des]=store[i]; } } int BFS(){ queue<int> q; while(!q.empty()) q.pop(); memset(dep,-1,sizeof(dep)); dep[src]=0; q.push(src); while(!q.empty()){ int u=q.front(); q.pop(); for(int v=src;v<=des;v++) if(map[u][v]>0 && dep[v]==-1){ dep[v]=dep[u]+1; q.push(v); } } return dep[des]!=-1; } int DFS(int u,int minx){ if(u==des) return minx; int tmp; for(int v=src;v<=des;v++) if(map[u][v]>0 && dep[v]==dep[u]+1 && (tmp=DFS(v,min(minx,map[u][v])))){ map[u][v]-=tmp; map[v][u]+=tmp; return tmp; } dep[u]=-1; return 0; } int Dinic(){ int ans=0,tmp; while(BFS()){ while(1){ tmp=DFS(src,INF); if(tmp==0) break; ans+=tmp; } } return ans; } int main(){ //freopen("input.txt","r",stdin); while(~scanf("%d",&n) && n){ memset(dis,0x3f,sizeof(dis)); total=0; src=0, des=n+1; for(int i=1;i<=n;i++){ scanf("%d",&gold[i]); total+=gold[i]; //宝藏的总数 } for(int i=1;i<=n;i++) scanf("%d",&store[i]); scanf("%d",&m); int u,v,w; while(m--){ scanf("%d%d%d",&u,&v,&w); dis[u][v]=dis[v][u]=w; } int l=0,r=100010; int ans=-1,tmp; while(l<=r){ int mid=(l+r)>>1; buildgraph(mid); tmp=Dinic(); if(tmp==total){ ans=mid; r=mid-1; }else l=mid+1; } if(ans==-1) printf("No Solution\n"); else printf("%d\n",ans); } return 0; }
#include<iostream> #include<cstdio> #include<cstring> using namespace std; const int INF=0x3f3f3f3f; const int VM=250; const int EM=20010; int pre[VM],mat[VM][VM],dis[VM][VM]; int gold[VM],stor[VM]; int n,m,total,src,des; void build(int num){ //在当前距离下 给通过的路径 memset(mat,0,sizeof(mat)); int i,j; for(i=1;i<=n;i++) for(j=1;j<=n;j++) if(dis[i][j]<=num) mat[i][j]=INF; for(i=1;i<=n;i++){ mat[src][i]=gold[i]; mat[i][des]=stor[i]; } } bool BFS(){ int q[VM+5]; memset(pre,0xff,sizeof(pre)); //-1 int front=0,rear=0; pre[src]=0; q[rear++]=src; while(front!=rear){ int u=q[front++]; front=front%VM; for(int v=1;v<=des;v++){ if(pre[v]!=-1 || mat[u][v]==0) continue; pre[v]=u; if(v==des) return 1; q[rear++]=v; rear=rear%VM; } } return 0; } int EK(){ int res=0; while(BFS()){ int tmp=INF; for(int i=des;i!=src;i=pre[i]) tmp=min(tmp,mat[pre[i]][i]); res+=tmp; for(int i=des;i!=src;i=pre[i]){ mat[pre[i]][i]-=tmp; mat[i][pre[i]]+=tmp; } } return res; } int main(){ //freopen("input.txt","r",stdin); int u,v,w; while(~scanf("%d",&n) && n){ memset(dis,0x3f,sizeof(dis)); total=0; //total是总的gold数 src=0,des=n+1; for(int i=1;i<=n;i++){ scanf("%d",&gold[i]); total+=gold[i]; } for(int i=1;i<=n;i++) scanf("%d",&stor[i]); scanf("%d",&m); while(m--){ scanf("%d%d%d",&u,&v,&w); dis[u][v]=w; dis[v][u]=w; } int l=0,r=10001; int ans=-1; while(l<=r){ int mid=(l+r)>>1; build(mid); int sum=EK(); if(sum>=total){ ans=mid; r=mid-1; }else l=mid+1; } if(ans==-1) printf("No Solution\n"); else printf("%d\n",ans); } return 0; }