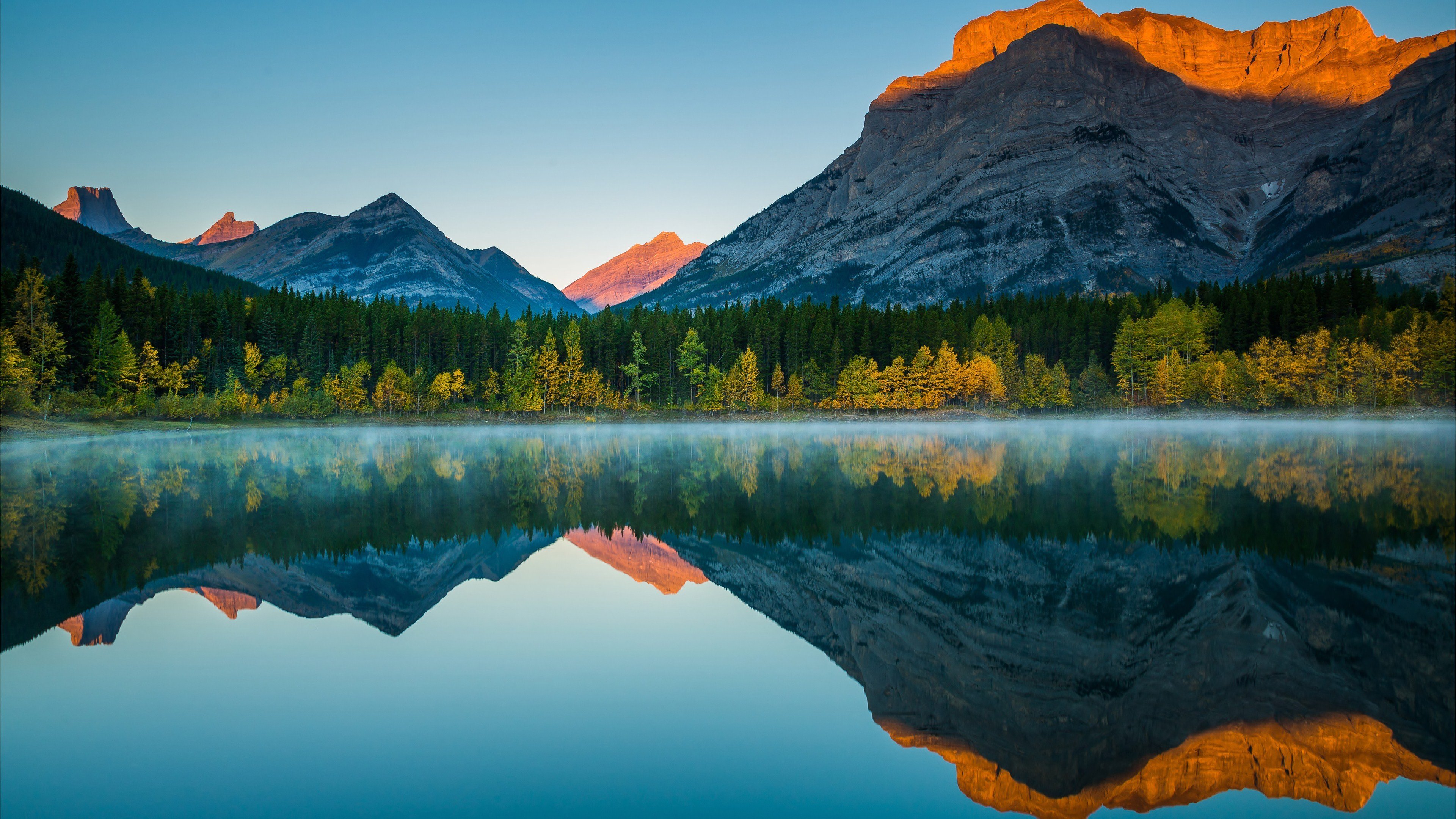
- std::map是一个包含了key-value键值对映射的排序分配容器。利用比较函数对key进行排序。搜索,删除和插入操作具有对数复杂性。 Maps通常实现为红黑树。标准库的每个地方都使用比较要求,唯一性通过使用等价关系来确定。
1、成员函数
- map::map:构造函数,用来构造映射;
- map::~map:析构函数,在对象撤销回收时调用;
- map::operator=:分配值到容器中;
- map::get_allocator:返回相关的分配值。
2、元素访问
- map::at:使用边界检查访问指定的元素;
- map::operator[]:接入或插入指定的元素。
3、迭代器Iterators(C++ 11)
- map::begin/cbegin:返回一个迭代器给开始beginning.
- map::end/cend:返回一个迭代器给末尾end.
- map::rbegin/:crbegin:返回一个反向迭代器给开始beginning.
- map::rend/crend:返回一个反向迭代器给末尾end.
4、容量Capacity
- map::empty:检查容器是否为空。
- map::size:返回元素的数量。
- map::max_size:返回最大的可能元素数量。
5、修改函数(C++ 11和C++ 17)
- map::clear:清楚元素内容。
- map::insert:插入元素或节点。
- map::insert_or_assign:插入一个元素,当key存在时,就分配给当前的元素。
- map::emplace: 就地构造元素。
- map::emplace_hint:使用提示就地构造元素。
- map::try_emplace:如果key不存在,则就地插入元素;如果key存在,则不进行任何操作。
- map::erase:擦除元素。
- map::swap:交换内容。
- map::extract:从容器中抽取节点。
- map:merge:从另一个容器中拼接节点。
6、查找表Lookup
- map::count:返回匹配具体key的元素数量。
- map::find:查找具体key的元素。
- map::contains:检查容器是否包含具体key的元素。
- map::equal_range:返回匹配具体key的元素范围。
- map::lower_bound:将迭代器返回给第一个不小于给定键的元素。
- map::upper_bound:将迭代器返回给第一个大于给定键的元素
7、观察Observers
- map::key_comp:返回比较key的函数。
- map::value_comp:返回比较value_type类型的对象中的键的函数。
举例:
#include <iostream>
#include <string>
#include <iomanip>
#include <map>
template<typename Map>
void print_map(Map& m) {
std::cout << '{';
for(auto& p: m)
std::cout << p.first << ':' << p.second << ' '; //first表示key, second表示value
std::cout << "}
";
}
struct Point {double x,y;};
struct PointCmp {
bool operator()(const Point& lhs, const Point& rhs) const {
return lhs.x < rhs.x;
}
};
int main() {
// 默认构造函数
std::map<std::string, int> map1;
map1["something"] = 69;
map1["anything"] = 199;
map1["that thing"] = 50;
std::cout << "map1 = ";
print_map(map1);
// 范围构造
std::map<std::string, int> iter(map1.find("something"), map1.end());
std::cout << "
iter = ";
print_map(iter);
std::cout << "map1 = ";
print_map(map1);
// 复制构造函数
std::map<std::string, int> copied(map1); //复制之后,原对象还存在数据
std::cout << "
copied = ";
print_map(copied);
std::cout << "map1 = ";
print_map(map1);
// 移动构造函数
std::map<std::string, int> moved(std::move(map1)); //移动之后,原对象数据消失
std::cout << "
moved = ";
print_map(moved);
std::cout << "map1 = ";
print_map(map1);
// 初始化构造列表
const std::map<std::string, int> init {
{"this", 100},
{"can", 100},
{"be", 100},
{"const", 100},
};
std::cout << "
init = ";
print_map(init);
// 自定义键类选项1
// 使用比较构造
std::map<Point, double, PointCmp> mag = {
{{5, -12}, 13},
{{3, 4}, 5},
{{-8, -15}, 17}
};
for(auto p : mag)
std::cout << "The magnitude of {" << p.first.x
<< ", " << p.first.y << ") is "
<< p.second << "
";
// 自定义键类选项1
// 使用lambda比较
auto cmpLambda = [&mag](const Point &lhs, const Point &rhs) { return mag[lhs] < mag[rhs]; };
std::map<Point, double, decltype(cmpLambda)> magy(cmpLambda);
// 插入各种元素的各种方法~
magy.insert(std::pair<Point, double> ({5, -12}, 13));
magy.insert({{3, 4}, 5});
magy.insert({Point{-8.0, -15.0}, 17});
std::cout << "
";
for(auto p : magy)
std::cout << "The magnitude of (" << p.first.x
<< ", " << p.first.y << ") is "
<< p.second << "
";
}
输出为:
map1 = {anything:199 something:69 that thing:50 }
iter = {anything:199 something:69 that thing:50 }
map1 = {anything:199 something:69 that thing:50 }
copied = {anything:199 something:69 that thing:50 }
map1 = {anything:199 something:69 that thing:50 }
moved = {anything:199 something:69 that thing:50 }
map1 = {}
init = {be:100 can:100 const:100 this:100 }
The magnitude of (-8, -15) is 17
The magnitude of (3, 4) is 5
The magnitude of (5, -12) is 13
The magnitude of (3, 4) is 5
The magnitude of (5, -12) is 13
The magnitude of (-8, -15) is 17