An inorder binary tree traversal can be implemented in a non-recursive way with a stack. For example, suppose that when a 6-node binary tree (with the keys numbered from 1 to 6) is traversed, the stack operations are: push(1); push(2); push(3); pop(); pop(); push(4); pop(); pop(); push(5); push(6); pop(); pop(). Then a unique binary tree (shown in Figure 1) can be generated from this sequence of operations. Your task is to give the postorder traversal sequence of this tree.
Figure 1
Input Specification:
Each input file contains one test case. For each case, the first line contains a positive integer N (≤) which is the total number of nodes in a tree (and hence the nodes are numbered from 1 to N). Then 2 lines follow, each describes a stack operation in the format: "Push X" where X is the index of the node being pushed onto the stack; or "Pop" meaning to pop one node from the stack.
Output Specification:
For each test case, print the postorder traversal sequence of the corresponding tree in one line. A solution is guaranteed to exist. All the numbers must be separated by exactly one space, and there must be no extra space at the end of the line.
Sample Input:
6
Push 1
Push 2
Push 3
Pop
Pop
Push 4
Pop
Pop
Push 5
Push 6
Pop
Pop
Sample Output:
3 4 2 6 5 1
题意:按先序输入,pop出的顺序是中序,输出后序。
1 #include<cstdio> 2 #include<cstdlib> 3 #include<cstring> 4 #include<iostream> 5 #include<stack> 6 using namespace std; 7 struct TNode 8 { 9 int left; 10 int right; 11 } Tree[35];//树的节点最多不超过 30 12 int s;//用来保存树的根的值 因为好像第一个测试用例的树根不是 1 13 void Create() 14 { memset(Tree,0,sizeof(Tree)); 15 int n; 16 scanf("%d",&n); 17 cin.get(); 18 stack<int >S; 19 int top; 20 char str[10]; 21 int data; 22 scanf("%s %d ",str,&data); 23 S.push(data); 24 s=top=S.top(); 25 26 for (int i=1; i<2*n; i++)//树根已入栈,故从i=1开始 27 { 28 scanf("%s",str); 29 if(strlen(str)!=3)//注意这个if结构 30 { 31 scanf("%d",&data); 32 S.push(data); 33 if(Tree[top].left==0)//左子树空,将data放入左子树 34 Tree[top].left=data; 35 else //左子树已被使用 36 Tree[top].right=data; 37 top=S.top();//更新top 38 } 39 else 40 {//注意这个大括号里的代码 41 top=S.top(); 42 S.pop(); 43 } 44 } 45 } 46 int k=0; 47 void Merge( int n)//控制输入输出格式 48 { 49 50 if(Tree[n].left!=0) 51 Merge(Tree[n].left); 52 if(Tree[n].right!=0) 53 Merge(Tree[n].right); 54 if(k==0) 55 { 56 printf("%d",n); 57 k=1; 58 } 59 else 60 printf(" %d",n); 61 } 62 int main() 63 { 64 Create(); 65 Merge(s); 66 return 0; 67
}
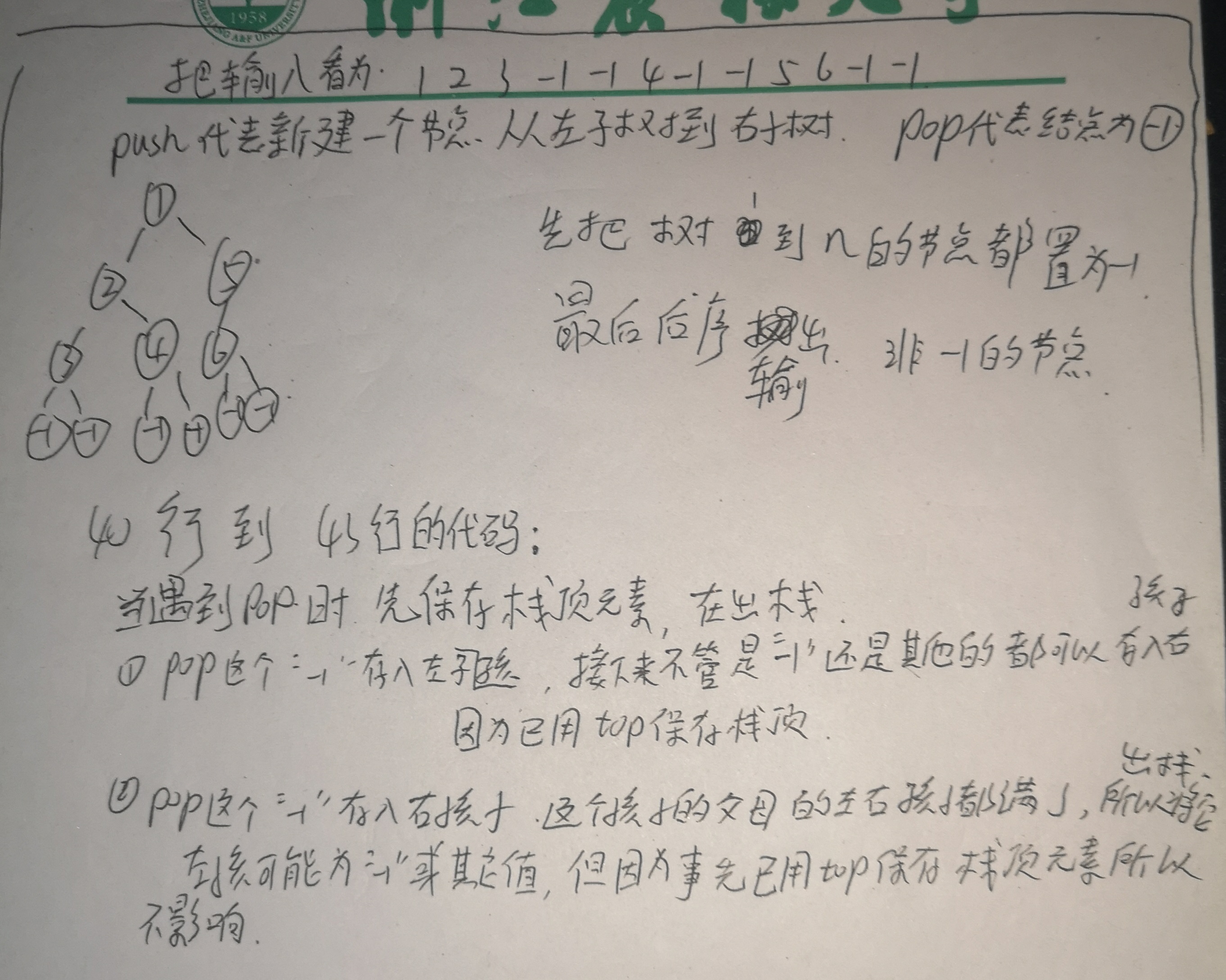