关注我的微信公众号,学习更多
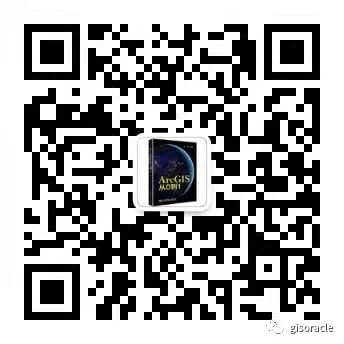
1 axTOCControl有关
1.1 右键弹出菜单
using ESRI.ArcGIS.Controls;
using ESRI.ArcGIS.Carto;
private void axTOCControl1_OnMouseDown(object sender, ESRI.ArcGIS.Controls.ITOCControlEvents_OnMouseDownEvent e)
{
if (e.button != 2) //响应右键点击事件
return;
System.Drawing.Point p = new System.Drawing.Point(e.x, e.y);
System.Drawing.Point pPoint = axTOCControl1.PointToScreen(p);
contextMenuStrip1.Show(pPoint.X, pPoint.Y);
}
1.2 axTOCControl1中隐藏图例
private void HideLayerInfo(ILayer layer)
{
ILegendInfo pLInfo;
pLInfo = layer as ILegendInfo;
ILegendGroup pLGroup;
int i;
for (i = 0; i < pLInfo.LegendGroupCount; i++)
{
pLGroup = pLInfo.get_LegendGroup(i);
pLGroup.Visible = false;
}
axTOCControl1.Update();
}
1.3 axTOCControl1中显示图例
private void ShowLayerInfo(ILayer layer)
{
ILegendInfo pLInfo;
pLInfo = layer as ILegendInfo;
ILegendGroup pLGroup;
int i;
for (i = 0; i < pLInfo.LegendGroupCount; i++)
{
pLGroup = pLInfo.get_LegendGroup(i);
pLGroup.Visible = true;
}
axTOCControl1.Update();
}
1.4 axTOCControl1刷新图例
axTOCControl1.Update();
1.5 axTOCControl1选中某个图层
axTOCControl1.SelectItem(layer, null);
1.6 axTOCControl1图层拖动
axTOCControl1.EnableLayerDragDrop = true;
1.7 axTOCControl1获得当前的图层
private void axTOCControl1_OnMouseDown(object sender, ESRI.ArcGIS.Controls.ITOCControlEvents_OnMouseDownEvent e)
{
esriTOCControlItem item = esriTOCControlItem.esriTOCControlItemNone;
IBasicMap map = null;
ILayer layer = null;
object other = null;
object index = null;
//Determine what kind of item has been clicked on
axTOCControl1.HitTest(e.x, e.y, ref item, ref map, ref layer, ref other, ref index);
MessageBox.Show(layer.Name);
}
1.8 axTOCControl1自定义
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
using ESRI.ArcGIS.Controls;
namespace lesson10
{
public partial class Form1 : Form
{
//定义全局的自定义对话框变量
private ICustomizeDialog m_CustomizeDialog = new CustomizeDialogClass();
//定义全局的自定义对话框启动事件委托
private ICustomizeDialogEvents_OnStartDialogEventHandler startDialogEventHandler;
//定义全局的自定义对话框关闭事件委托
private ICustomizeDialogEvents_OnCloseDialogEventHandler closeDialogEventHandler;
public Form1()
{
InitializeComponent();
createCustomizeDialog();
}
//该方法对委托和定制对话框进行设置
private void createCustomizeDialog()
{
startDialogEventHandler = new ICustomizeDialogEvents_OnStartDialogEventHandler(onStartDialog);
((ICustomizeDialogEvents_Event)m_CustomizeDialog).OnStartDialog += startDialogEventHandler;
closeDialogEventHandler = new ICustomizeDialogEvents_OnCloseDialogEventHandler(onCloseDialog);
((ICustomizeDialogEvents_Event)m_CustomizeDialog).OnCloseDialog += closeDialogEventHandler;
m_CustomizeDialog.DialogTitle = "自定义工具栏项";
m_CustomizeDialog.SetDoubleClickDestination(this.axToolbarControl1);
}
private void onStartDialog()
{
this.axToolbarControl1.Customize = true;
}
private void onCloseDialog()
{
}
private void cbCustomize_CheckedChanged(object sender, EventArgs e)
{
if (this.cbCustomize.Checked == false)
{
m_CustomizeDialog.CloseDialog();
}
else
{
m_CustomizeDialog.StartDialog(this.axToolbarControl1.hWnd);
}
}
}
}