css json格式化展示样式
<style>
.console {
display: block;
padding: 9.5px;
margin: 44px 0 0 0;
font-size: 13px;
line-height: 1.42857;
color: #333;
word-break: break-all;
word-wrap: break-word;
background-color: #F5F5F5;
border: 1px solid #CCC;
border-radius: 4px;
}
</style>
html 页面用到pre标签
<pre id="pre" class="console"></pre>
<form action="get">
<input type="text" name="path" id="path" value="">
<input type="file" name="file" id="file" value="">
<input type="button" name="button" value="提交">
</form>
<script>
$("input[name='button']").click(function () {
var fd = new FormData();
fd.append("path", $("#path").val());
fd.append("file", $('#file')[0].files[0]);
$.ajax({
url: 'http://xxx.com/upload',
type: 'post',
processData: false,
contentType: false,
data: fd,
success: function (res) {
$("#pre").html(JSON.stringify(res, null, 2));
console.log(res)
},
error: function (err) {
console.log(err)
}
})
});
</script>
java 后台mule接收java上传oss
package com.ssjf.esb.other.transform;
import cn.hutool.core.util.ObjectUtil;
import com.aliyun.oss.OSS;
import com.aliyun.oss.OSSClientBuilder;
import org.mule.api.MuleMessage;
import org.mule.api.transformer.TransformerException;
import org.mule.config.i18n.Message;
import org.mule.config.i18n.MessageFactory;
import org.mule.module.http.internal.multipart.HttpPart;
import org.mule.module.http.internal.multipart.HttpPartDataSource;
import org.mule.transformer.AbstractMessageTransformer;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Value;
import javax.activation.DataHandler;
import java.io.InputStream;
import java.io.UnsupportedEncodingException;
import java.nio.charset.StandardCharsets;
import java.text.SimpleDateFormat;
import java.util.Date;
/***
* 文件上传
* @author fuqian
* @date 2022/6/21
**/
public class FileUploadTransfrom extends AbstractMessageTransformer {
Logger log = LoggerFactory.getLogger(FileUploadTransfrom.class);
@Value("${oss.url}")
String ossUrl;
@Value("${oss.enabled}")
boolean ossEnabled;
@Value("${oss.name}")
String ossName;
@Value("${oss.tenant-mode}")
boolean ossTenantMode;
@Value("${oss.endpoint}")
String ossEndpoint;
@Value("${oss.access-key}")
String ossAccessKey;
@Value("${oss.secret-key}")
String ossSecretKey;
@Value("${oss.bucket-name}")
String ossBucketName;
@Override
public Object transformMessage(MuleMessage message, String outputEncoding) throws TransformerException {
// file是表单文件上传所设置的name值
DataHandler dataHandler = message.getInboundAttachment("file");
// 获取文件名称
HttpPartDataSource dataSource = (HttpPartDataSource) dataHandler.getDataSource();
HttpPart httpPart = (HttpPart) dataSource.getPart();
String contentDisposition = httpPart.getHeader("Content-Disposition");
String tempFileName = contentDisposition.substring(contentDisposition.indexOf("filename=\"") + 10, contentDisposition.lastIndexOf("\""));
// file是表单文件上传所设置的name值
DataHandler pathHandler = message.getInboundAttachment("path");
InputStream inputStream = null;
String url = null;
String path = null;
try {
// JAVA 设置字符串编码 上传文件文件名乱码问题解决
String fileName = new String(tempFileName.getBytes(StandardCharsets.ISO_8859_1), StandardCharsets.UTF_8);
log.info("上传文件fileName = {}", fileName);
inputStream = dataSource.getInputStream();
// 创建OSSClient实例
OSS ossClient = new OSSClientBuilder().build(ossEndpoint, ossAccessKey, ossSecretKey);
if (ObjectUtil.isNull(pathHandler)) {
// 获得当前时间作为文件夹
Date date = new Date();
SimpleDateFormat simpleDateFormat = new SimpleDateFormat("yyyy/MM/dd");
path = simpleDateFormat.format(date);
} else {
path = (String) pathHandler.getContent();
}
String objectName = "mule" + "/" + path + "/" + fileName;
ossClient.putObject(ossBucketName, objectName, inputStream);
url = ossUrl + path + "/" + fileName;
} catch (Exception e) {
log.error("上传文件: 异常:{}", e.getMessage(), e);
String msgInfo = String.format("处理请求参数: 异常:%s", e.getLocalizedMessage());
try {
msgInfo = new String(msgInfo.getBytes(), "UTF-8");
} catch (UnsupportedEncodingException e1) {
e1.printStackTrace();
}
Message msg = MessageFactory.createStaticMessage(msgInfo);
throw new TransformerException(msg);
}
return url;
}
}
maven java aliyun oss 依赖
<!--阿里云oss sdk依赖-->
<dependency>
<groupId>com.aliyun.oss</groupId>
<artifactId>aliyun-sdk-oss</artifactId>
<version>3.1.0</version>
</dependency>
效果 上传文件后,响应结果打印到前台页面展示
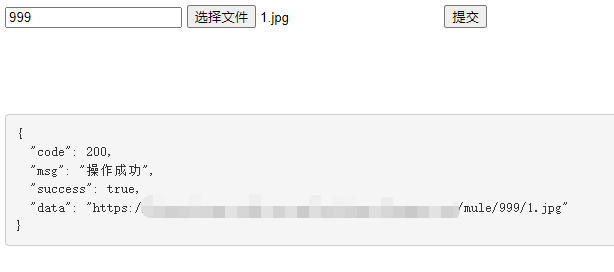
注意 配置返回结果的请求头 解决跨域
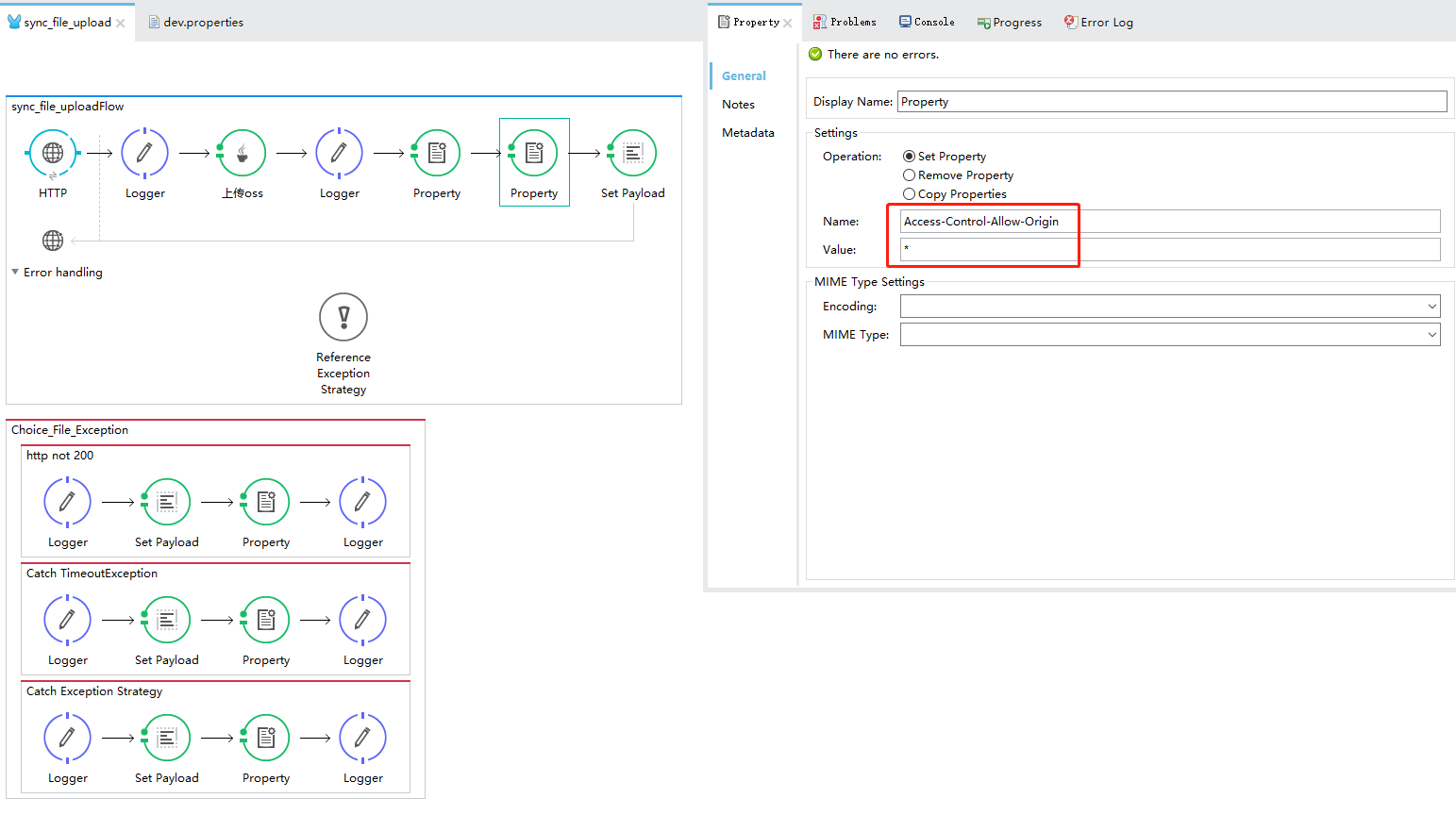