1. UI 书写
最基本创建一个label 标签 写一个first rate :
UILabel *label = [[UILabel alloc] initWithFrame:CGRectMake(10, 50, 300, 50)];
label.backgroundColor = [UIColor orangeColor];
label.text = @"first rate";
label.textColor = [UIColor blueColor];
label.textAlignment = NSTextAlignmentCenter;
[self.window addSubview:label];
label.backgroundColor = [UIColor orangeColor];
label.text = @"first rate";
label.textColor = [UIColor blueColor];
label.textAlignment = NSTextAlignmentCenter;
[self.window addSubview:label];
遍历所有字体:
NSArray *fontArray = [UIFont familyNames];
for (int i=0; i<fontArray.count; ++i) {
NSLog(@"font:%@", fontArray[i]);
}
label.font = [UIFont fontWithName:@"Menlo" size:30];
for (int i=0; i<fontArray.count; ++i) {
NSLog(@"font:%@", fontArray[i]);
}
label.font = [UIFont fontWithName:@"Menlo" size:30];
写一个带有阴影的字体:
UILabel *secondLabel = [[UILabel alloc] initWithFrame:CGRectMake(10, 150, 300, 50)];
secondLabel.backgroundColor = [UIColor yellowColor];
secondLabel.text = @"second rate";
secondLabel.shadowColor = [UIColor lightGrayColor];
secondLabel.shadowOffset=CGSizeMake(5, 5);
secondLabel.backgroundColor = [UIColor yellowColor];
secondLabel.text = @"second rate";
secondLabel.shadowColor = [UIColor lightGrayColor];
secondLabel.shadowOffset=CGSizeMake(5, 5);
写一个 adjustsFontSizeToFitWidth 方法的函数
UILabel *thirdLabel = [[UILabel alloc] initWithFrame:CGRectMake(10, 250, 300, 100)];
thirdLabel.backgroundColor = [UIColor greenColor];
thirdLabel.text = @"alsdfjalksdfjlas;dfalsjksdfsdfsfdfsdff;lasdjfksaklfj";
thirdLabel.adjustsFontSizeToFitWidth=YES;
//adjustsFontSizeToFitWidth 会自动缩小字体而刚好在这个Label框框中,但不会主动换行
thirdLabel.backgroundColor = [UIColor greenColor];
thirdLabel.text = @"alsdfjalksdfjlas;dfalsjksdfsdfsfdfsdff;lasdjfksaklfj";
thirdLabel.adjustsFontSizeToFitWidth=YES;
//adjustsFontSizeToFitWidth 会自动缩小字体而刚好在这个Label框框中,但不会主动换行
写一个文本再框框中可以主动换行的例子
UILabel *forthLabel = [[UILabel alloc] initWithFrame:CGRectMake(10, 350, 300, 100)];
forthLabel.textColor = [UIColor orangeColor];
forthLabel.text = @"adjustsFontSizeToFitWidth 会自动缩小字体而刚好在这个Label框框中,但不会主动换行";
forthLabel.font = [UIFont boldSystemFontOfSize:30];
forthLabel.numberOfLines = 0; //主动换行
forthLabel.backgroundColor = [UIColor blueColor];
//在框框能允许的范围内可以主动换行,如果框框无法包容文本大小,则会用..省略
forthLabel.textColor = [UIColor orangeColor];
forthLabel.text = @"adjustsFontSizeToFitWidth 会自动缩小字体而刚好在这个Label框框中,但不会主动换行";
forthLabel.font = [UIFont boldSystemFontOfSize:30];
forthLabel.numberOfLines = 0; //主动换行
forthLabel.backgroundColor = [UIColor blueColor];
//在框框能允许的范围内可以主动换行,如果框框无法包容文本大小,则会用..省略
写一个文本框框自动按照字体的多少自动延伸的例子
NSString *str = @"这个Label可以根据字体的多少无限延长,如同如意金箍棒!";
UIFont *font = [UIFont boldSystemFontOfSize:30];
CGSize size = [str sizeWithFont:font constrainedToSize:CGSizeMake(300, 480)];
UILabel *autoLabel = [[UILabel alloc] initWithFrame:CGRectMake(10, 220, size.width, size.height)];
autoLabel.text = str;
autoLabel.numberOfLines = 0;
autoLabel.textColor = [UIColor redColor];
autoLabel.backgroundColor = [UIColor blueColor];
autoLabel.font = font;
UIFont *font = [UIFont boldSystemFontOfSize:30];
CGSize size = [str sizeWithFont:font constrainedToSize:CGSizeMake(300, 480)];
UILabel *autoLabel = [[UILabel alloc] initWithFrame:CGRectMake(10, 220, size.width, size.height)];
autoLabel.text = str;
autoLabel.numberOfLines = 0;
autoLabel.textColor = [UIColor redColor];
autoLabel.backgroundColor = [UIColor blueColor];
autoLabel.font = font;
写一个小例子 打印出下面生成随机小框框 图片的框框
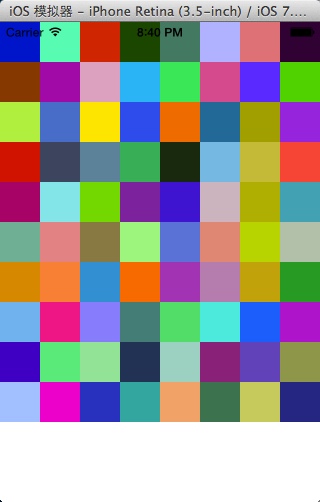
代码如下:
for (int i = 0; i< 320; i+=40) {
for (int j = 0; j<380; j+=40) {
CGRect rect = CGRectMake(i, j, 40, 40);
CGFloat red = rand()/(RAND_MAX*1.0);
CGFloat green = rand()/(RAND_MAX*1.0);
CGFloat blue = rand()/(RAND_MAX*1.0);
CGFloat alpha = rand()/(RAND_MAX*1.0);
UIColor *myColer = [UIColor colorWithRed:red green:green blue:blue alpha:1];
UIView *view = [[UIView alloc] initWithFrame:rect];
view.backgroundColor = myColer;
[self.window addSubview:view];
}
}
for (int j = 0; j<380; j+=40) {
CGRect rect = CGRectMake(i, j, 40, 40);
CGFloat red = rand()/(RAND_MAX*1.0);
CGFloat green = rand()/(RAND_MAX*1.0);
CGFloat blue = rand()/(RAND_MAX*1.0);
CGFloat alpha = rand()/(RAND_MAX*1.0);
UIColor *myColer = [UIColor colorWithRed:red green:green blue:blue alpha:1];
UIView *view = [[UIView alloc] initWithFrame:rect];
view.backgroundColor = myColer;
[self.window addSubview:view];
}
}
第二个小例子,完成下面图片的代码:
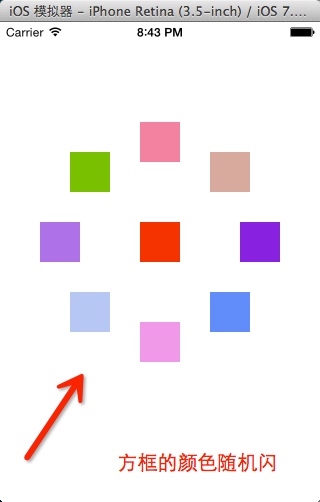
小方框可以闪动UI代码:
{
NSTimer *time;
}
time = [NSTimer scheduledTimerWithTimeInterval:1 target:self selector:@selector(ontime) userInfo:nil repeats:YES];
-(void)ontime
{
for (int i= 120; i< 400; i+=100) {
CGRect rect = CGRectMake(0, 0, 40, 40);
CGFloat red = arc4random()/(0xffffffff*1.0);
CGFloat green = arc4random()/(0xffffffff*1.0);
CGFloat blue = arc4random()/(0xffffffff*1.0);
CGFloat alpha = arc4random()/(0xffffffff*1.0);
UIColor *myColer = [UIColor colorWithRed:red green:green blue:blue alpha:1];
UIView *view = [[UIView alloc] initWithFrame:rect];
view.backgroundColor = myColer;
view.center = CGPointMake(160, i);
[self.window addSubview:view];
}
for (int i= 60; i< 320; i+=200) {
CGRect rect = CGRectMake(0, 0, 40, 40);
CGFloat red = arc4random()/(0xffffffff*1.0);
CGFloat green = arc4random()/(0xffffffff*1.0);
CGFloat blue = arc4random()/(0xffffffff*1.0);
CGFloat alpha = arc4random()/(0xffffffff*1.0);
UIColor *myColer = [UIColor colorWithRed:red green:green blue:blue alpha:1];
UIView *view = [[UIView alloc] initWithFrame:rect];
view.backgroundColor = myColer;
view.center = CGPointMake(i, 220);
[self.window addSubview:view];
}
for (int i= 90; i< 320; i+=140) {
CGRect rect = CGRectMake(0, 0, 40, 40);
CGFloat red = arc4random()/(0xffffffff*1.0);
CGFloat green = arc4random()/(0xffffffff*1.0);
CGFloat blue = arc4random()/(0xffffffff*1.0);
CGFloat alpha = arc4random()/(0xffffffff*1.0);
UIColor *myColer = [UIColor colorWithRed:red green:green blue:blue alpha:1];
UIView *view = [[UIView alloc] initWithFrame:rect];
view.backgroundColor = myColer;
view.center = CGPointMake(i, 150);
[self.window addSubview:view];
}
for (int i= 90; i< 320; i+=140) {
CGRect rect = CGRectMake(0, 0, 40, 40);
CGFloat red = arc4random()/(0xffffffff*1.0);
CGFloat green = arc4random()/(0xffffffff*1.0);
CGFloat blue = arc4random()/(0xffffffff*1.0);
CGFloat alpha = arc4random()/(0xffffffff*1.0);
UIColor *myColer = [UIColor colorWithRed:red green:green blue:blue alpha:1];
UIView *view = [[UIView alloc] initWithFrame:rect];
view.backgroundColor = myColer;
view.center = CGPointMake(i, 290);
[self.window addSubview:view];
}
}