1.对基础类型进行排序
调用Sort()方法,如果需要降序
1 List<int> intList = new List<int>() { 1, 2, 3 }; 2 intList.Sort(); 3 foreach (int number in intList) 4 { 5 Console.WriteLine(number); 6 } 7 intList.Reverse(); 8 foreach (int number in intList) 9 { 10 Console.WriteLine(number); 11 } 12 Console.ReadKey();
2.对非基本类型进行排序,里面有两个属性,重写了ToString方法
1 class Student 2 { 3 private int studentId; 4 private string studentName; 5 6 public int StudentId { get => studentId; set => studentId = value; } 7 public string StudentName { get => studentName; set => studentName = value; } 8 //重写构造方法 9 public override string ToString() 10 { 11 return "studentId:" + studentId + " studentName:" + studentName; 12 } 13 }
然后添加一些数据,仍希望用Sort排序
1 List<Student> studentList = new List<Student>(); 2 Student objStu1 = new Student() { StudentId = 1, StudentName = "小王" }; 3 Student objStu2 = new Student() { StudentId = 2, StudentName = "小李" }; 4 Student objStu3 = new Student() { StudentId = 3, StudentName = "小范" }; 5 studentList.Add(objStu1); 6 studentList.Add(objStu2); 7 studentList.Add(objStu3); 8 studentList.Sort(); 9 foreach (Student objStu in studentList) 10 { 11 Console.WriteLine(objStu); 12 } 13 Console.ReadKey();
然后就出错了,出错信息如下:
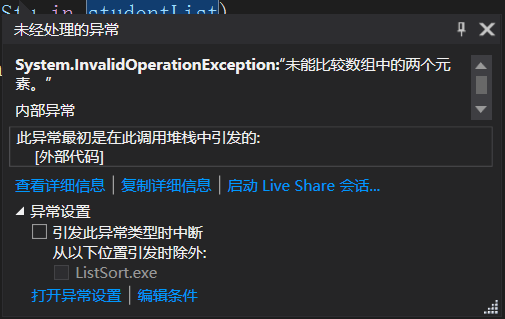
查看Sort源码可知它有如下几个重载

3.实现IComparable接口(默认排序)
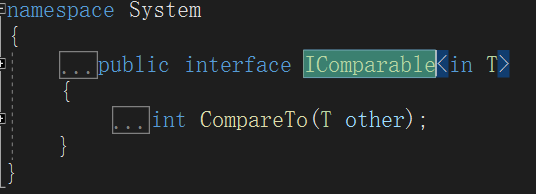
可以看到它只有一个方法,我们只需要修改类本身
1 class Student:IComparable<Student> 2 { 3 private int studentId; 4 private string studentName; 5 6 public int StudentId { get => studentId; set => studentId = value; } 7 public string StudentName { get => studentName; set => studentName = value; } 8 9 //实现接口 10 // 返回结果: 11 // 一个值,指示要比较的对象的相对顺序。 返回值的含义如下: 12 // 值 含义 小于零 此实例在排序顺序中位于 other 之前。 13 //零 此实例中出现的相同位置在排序顺序中是 14 // other。 大于零 此实例在排序顺序中位于 other 之后。 15 public int CompareTo(Student other) 16 { 17 return this.studentId.CompareTo(other.studentId); 18 } 19 20 //重写构造方法 21 public override string ToString() 22 { 23 return "studentId:" + studentId + " studentName:" + studentName; 24 } 25 }
运行结果:
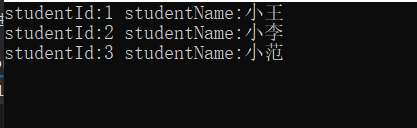
4.集合的动态排序
定义4个排序类,并且分别实现排序接口
1 class Student : IComparable<Student> 2 { 3 private int studentId; 4 private string studentName; 5 6 public int StudentId { get => studentId; set => studentId = value; } 7 public string StudentName { get => studentName; set => studentName = value; } 8 9 //实现接口 10 // 返回结果: 11 // 一个值,指示要比较的对象的相对顺序。 返回值的含义如下: 值 含义 小于零 此实例在排序顺序中位于 other 之前。 零 此实例中出现的相同位置在排序顺序中是 12 // other。 大于零 此实例在排序顺序中位于 other 之后。 13 public int CompareTo(Student other) 14 { 15 return this.studentId.CompareTo(other.studentId); 16 } 17 18 //重写构造方法 19 public override string ToString() 20 { 21 return "studentId:" + studentId + " studentName:" + studentName; 22 } 23 } 24 //添加4个排序类,并且分别实现排序接口 25 class StuNameASC : IComparer<Student> 26 { 27 public int Compare(Student x, Student y) 28 { 29 return x.StudentName.CompareTo(y.StudentName); 30 } 31 } 32 class StuNameDESC : IComparer<Student> 33 { 34 public int Compare(Student x, Student y) 35 { 36 return y.StudentName.CompareTo(x.StudentName); 37 } 38 } 39 40 class StudentIdASC : IComparer<Student> 41 { 42 public int Compare(Student x, Student y) 43 { 44 return x.StudentId.CompareTo(y.StudentId); 45 } 46 } 47 class StudentIdDESC : IComparer<Student> 48 { 49 public int Compare(Student x, Student y) 50 { 51 return y.StudentId.CompareTo(x.StudentId); 52 } 53 }
1 在排序时使用Sort的重载方法 2 public void Sort(IComparer<T> comparer); 3 传递的是实现这个接口的类的对象 4 studentList.Sort(new StudentIdASC):表示的是按照学号升序排列
结果如下:
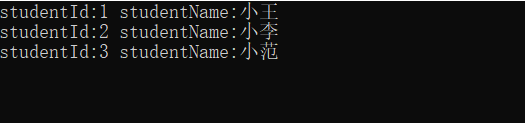
5.总结
泛型集合中基本数据类型可以直接排序,非基本数据类型要自定义排序方法