一、codable功能
OC常用模式:
示例代码如下:
NSDictionary *metaDic = [NSJSONSerialization JSONObjectWithData:metaData options:0 error:&error]; - (instancetype)initWithContentDictionaty:(NSDictionary *)contentDic { self = [super init]; if (self) { //_blockId = [[contentDic valueForKey:@"block_id"] integerValue]; //V4去除 _blockType = [[contentDic valueForKey:@"block_type"] integerValue]; _blockIndex = [[contentDic valueForKey:@"block_index"] integerValue]; _blockStyle = [contentDic valueForKey:@"block_style"]; _blockContent = [contentDic valueForKey:@"block_content"]; _imgUUID = [contentDic valueForKey:@"img_uuid"]; //_storyId = [[contentDic valueForKey:@"story_id"] integerValue]; //v4去除 } return self; }
swift codable实现从json字符串 直接转 model
二、原理
codable协议分为 解码codable和编码codable
typealias Codable = Decodable & Encodable
协议实现
protocol Encodable {
func encode(to encoder: Encoder) throws
}
protocol Decodable {
init(from decoder: Decoder) throws
}
使用方法
class Person:Codable { var name:String var age:Int var quest:String } let dic = ["name":"huahua","age":10,"quest":"1"] as [String : Any] let JSONString = "{"name":"xiaoming","age":10,"quest":"xiaoming"}" guard let jsonData = JSONString.data(using: .utf8)else{ return } let decoder = JSONDecoder() guard let obj = try?decoder.decode(Person.self, from: jsonData)else{ return } print(obj.name) print(obj.age) print(obj四、问题实战
1、json.key > model.property, 成功解析
2、json.key < model.property,解析失败,只要class中又一个属性在json.key中找不到,解析失败
解决方案一:sever有些字段可传可不传,将model中多出来的字段 设置为可选
解决方案二:sever有些字段永远不传入,重写codingKeys
3、sever字段与前端 关键字冲突解决方案
前端字段加上'default'
4、对象引用的枚举类型数量 和 sever不匹配
重写枚举类型初始化方法
enum RequstState:String,Codable,RawRepresentable{ case success = "success" case failed = "failed" case unkown } extension RequstState{ //可失败的初始化构造器 init?(rawValue: Self.RawValue){ switch rawValue { case "failed": self = .success case "success": self = .failed default: self = .unkown } } }
5、何时需要写codingKey
前端定制字段名称
6、何时重写decoder
前端定制decode方案,默认值赋值等
7、何时重写encoder
前端定制enCode方案,默认值赋值等,如下为重写decode示例代码
前端定制字段名称
6、何时重写decoder
前端定制decode方案,默认值赋值等
7、何时重写encoder
前端定制enCode方案,默认值赋值等,如下为重写decode示例代码
struct Student:Codable{ var id:String // var name:String var grade:Int? var state:RequstState init(from decoder: Decoder) throws{ let container = try decoder.container(keyedBy: CodingKeys.self) do { state = try container.decodeIfPresent(RequstState.self, forKey: .state) } catch { state = nil } do { grade = try container.decodeIfPresent(Int.self, forKey: .grade) } catch { grade = nil } do { id = try container.decodeIfPresent(String.self, forKey: .id) ?? "" } catch { id = "" } } }
五、项目遇到特殊问题
1、codable json串中 value为string ,string包含 转义字符的 json 字典格式串,这种形式只能先解包成string,然后特殊处理

code中的样子大致如下图
注:"""三引号表示 中间的字符串 保留换行格式
六、扩展
1、string Dictionay 相互转化
2、model to string
var snsDescription: String { guard let data = try? JSONEncoder().encode(self)else{ return "(type(of: self)) 模型转 json字符串失败" } guard let str = String.init(data: data, encoding: .utf8) else { return "(type(of: self)) 模型转 json字符串失败" } return str }
理论上讲 请求发送 也可以封装成 model 传入 请求模型,请求库负责转成string 或 dic 发送网络请求,目前实际使用中,使用的是Dic ,code如下图,组装字典过程中,有使用到model to string 的例子,UgcDynamicPic ---> Dic
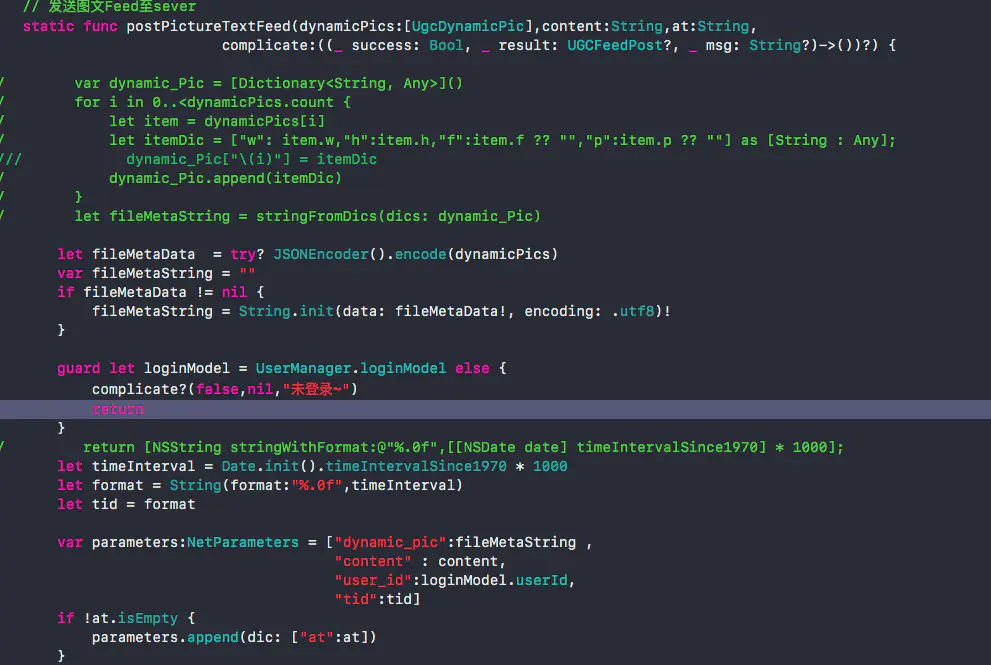
参考:https://www.jianshu.com/p/36eccbacfb0a