2.字段Field
2.1.通过Class实例获取字段field信息:
- getField(name): 获取某个public的field,包括父类
- getDeclaredField(name): 获取当前类的某个field,不包括父类
- getFields(): 获取所有public的field,包括父类
- getDeclaredFields(): 获取当前类的所有field,不包括父类
Hello.java
package com.reflection;
import java.util.ArrayList;
public interface Hello {
ArrayList[] listname = new ArrayList[5];
public void hello();
}
Student.java
package com.reflection;
public class Student implements Hello{
private String name;
public int age ;
public Student(){
this("unNamed");
}
public Student(String name){
this.name=name;
}
public void hello(){
System.out.println(name+" is Student");
}
}
Main.java
package com.reflection;
import java.lang.reflect.Field;
public class Main {
public static void main(String[] args) throws NoSuchFieldException{
Class cls = Student.class;
System.out.println("获取age:"+cls.getField("age"));
System.out.println();
System.out.println("获取父类的listname:"+cls.getField("listname"));
System.out.println();
System.out.println("获取age:"+cls.getDeclaredField("age"));
System.out.println();
try{
System.out.println("获取父类的listname:"+cls.getDeclaredField("listname"));
}catch (Exception e){
System.out.println("获取父类的listname:"+e);
}
System.out.println();
System.out.println("获取全部的field,包含父类:");
for(Field field:cls.getFields()){
System.out.println(field);
}
System.out.println();
System.out.println("获取全部的field,不包括父类:");
for(Field field:cls.getDeclaredFields()){
System.out.println(field);
}
}
}
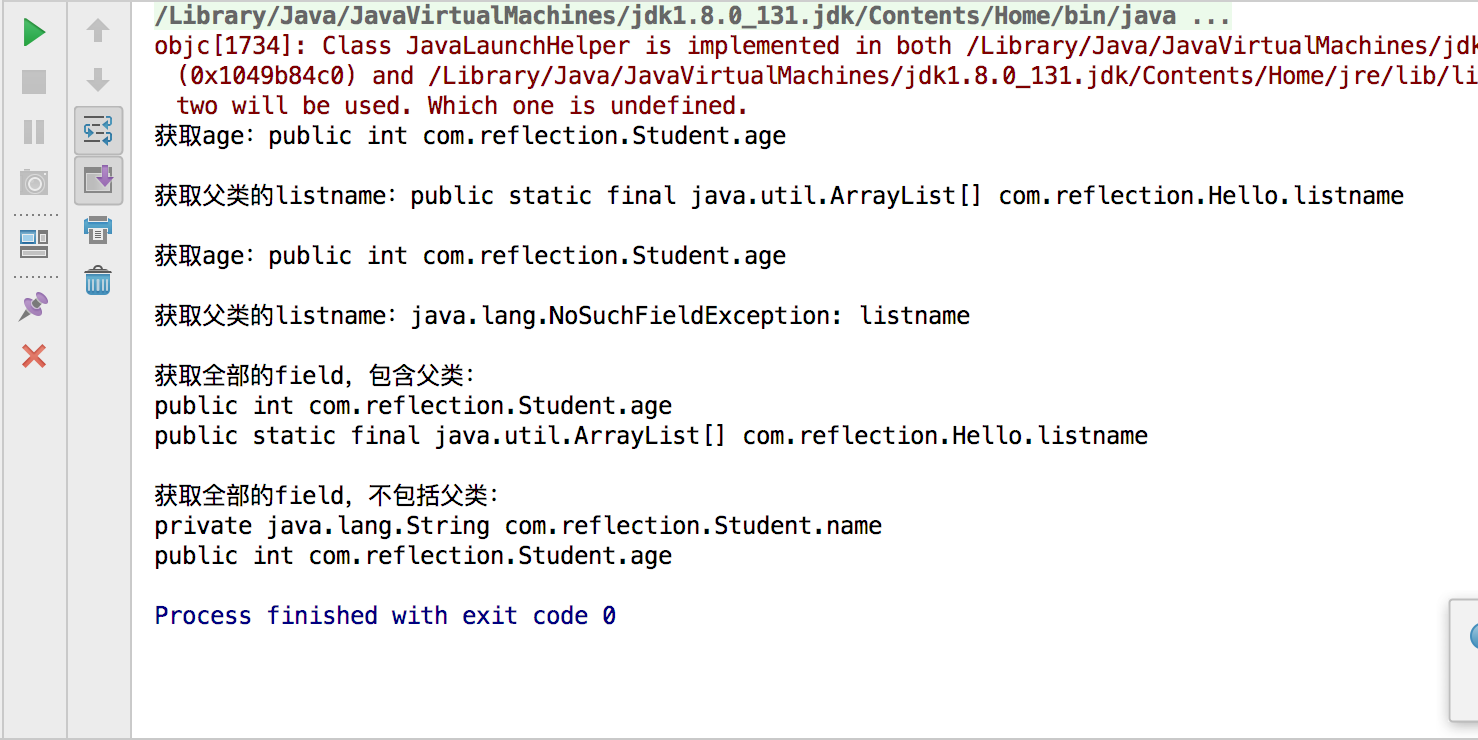
2.2.Field对象包含一个field的所有信息:
- getName()
- getType()
- getModifiers()
JAVA 反射机制中,Field的getModifiers()方法返回int类型值表示该字段的修饰符。
其中,该修饰符是java.lang.reflect.Modifier的静态属性。
对应表如下:
PUBLIC: 1 PRIVATE: 2 PROTECTED: 4 STATIC: 8
FINAL: 16 SYNCHRONIZED: 32 VOLATILE: 64
TRANSIENT: 128 NATIVE: 256 INTERFACE: 512
ABSTRACT: 1024 STRICT: 2048
Main.java
package com.reflection;
import java.lang.reflect.Field;
public class Main {
public static void main(String[] args) throws NoSuchFieldException{
Class cls = Student.class;
Field field1 = cls.getField("age");
System.out.println(field1.getName());
System.out.println(field1.getType());
System.out.println(field1.getModifiers());
}
}
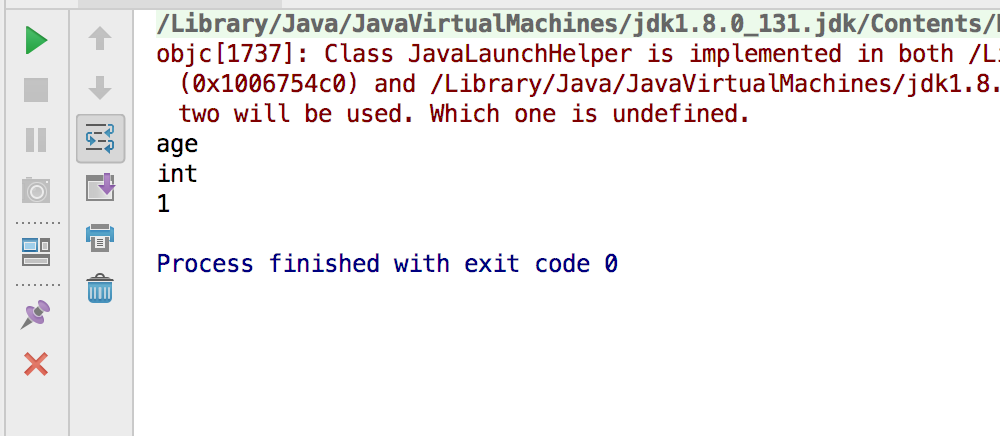
2.3.获取和设置field的值:
- get(Object obj):
- set(Object,Object)
//疯狂Java840页
import java.lang.reflect.Field;
class Person {
private String name;
private int age;
public String toString(){
return "Person[name:" + name + ", age:" +age +"]";
}
}
public class FieldTest{
public static void main(String[] args) throws Exception{
Class cls = Person.class;
Person p = (Person) cls.newInstance();
Field nameField = cls.getDeclaredField("name");
nameField.setAccessible(true);
nameField.set(p,"小明");
Field ageField = cls.getDeclaredField("age");
ageField.setAccessible(true);
ageField.set(p,12);
System.out.println(p.toString());
System.out.println(ageField.get(p));
System.out.println(nameField.get(p));
}
}
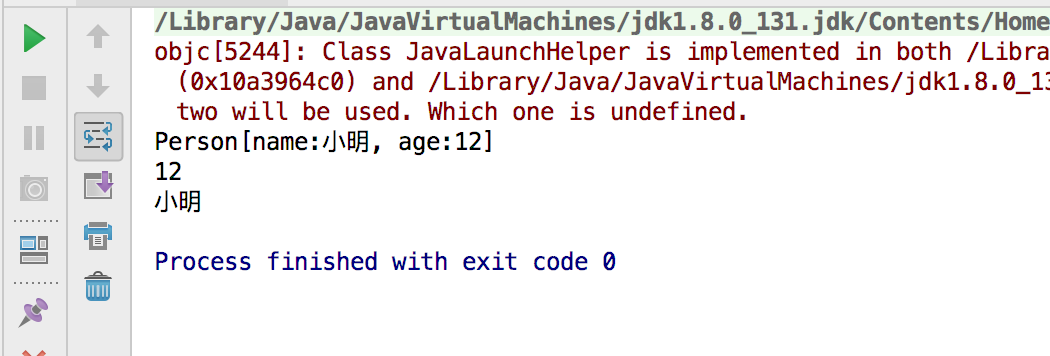
2.4.通过反射访问Field需要通过SecurityManager设置的规则。
通过设置setAccessible(true)来访问非public字段。
3.调用方法Method
3.1通过class实例获取方法Method信息:
- getMethod(name,Class...):获取某个public的method(包括父类)
- getDeclaredMethod(name,Class...):获取当前类的某个method(不包括父类)
- getMethods():获取所有public的method(包括父类)
- getDeclaredMethods():获取当前类的所有method(不包括父类)
Animal.java
package com.reflection;
public class Animal {
public void run(){
System.out.println("动物再跑");
}
private void eat(String s,String i){
System.out.println("动物每天能吃"+i+"Kg"+s);
}
}
Dog.java
package com.reflection;
public class Dog extends Animal {
public void bar(){
System.out.println("狗在叫");
}
private void func(){
System.out.println("狗能看家");
}
}
Go.java
package com.reflection;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
public class Go {
public static void main(String[] args) throws ClassNotFoundException,NoSuchMethodException,IllegalAccessException,InstantiationException,InvocationTargetException {
Class cls = Class.forName("com.reflection.Dog");
Dog dog = (Dog)cls.newInstance();
System.out.println("getMethod获取某个public的method,包括父类");
Method pubMethod1 = cls.getMethod("run");
System.out.print(pubMethod1.getName()+":");
pubMethod1.invoke(dog);
Method pubMethod2 = cls.getMethod("bar");
System.out.print(pubMethod2.getName()+":");
pubMethod2.invoke(dog);
System.out.println();
System.out.println("getDeclaredMethod:获取当前类的某个method,不包括父类");
Method subMethod1 = cls.getDeclaredMethod("func");
System.out.println(subMethod1.getName());
Method subMethod2 = cls.getDeclaredMethod("bar");
System.out.println(subMethod2.getName());
System.out.println();
System.out.println("getMethods():获取所有public的method(包括父类)");
for(Method m:cls.getMethods()){
System.out.println(m.getName());
}
System.out.println();
System.out.println("getDeclaredMethods():获取当前类的所有method(不包括父类)");
for(Method m:cls.getDeclaredMethods()){
System.out.println(m.getName());
}
}
}
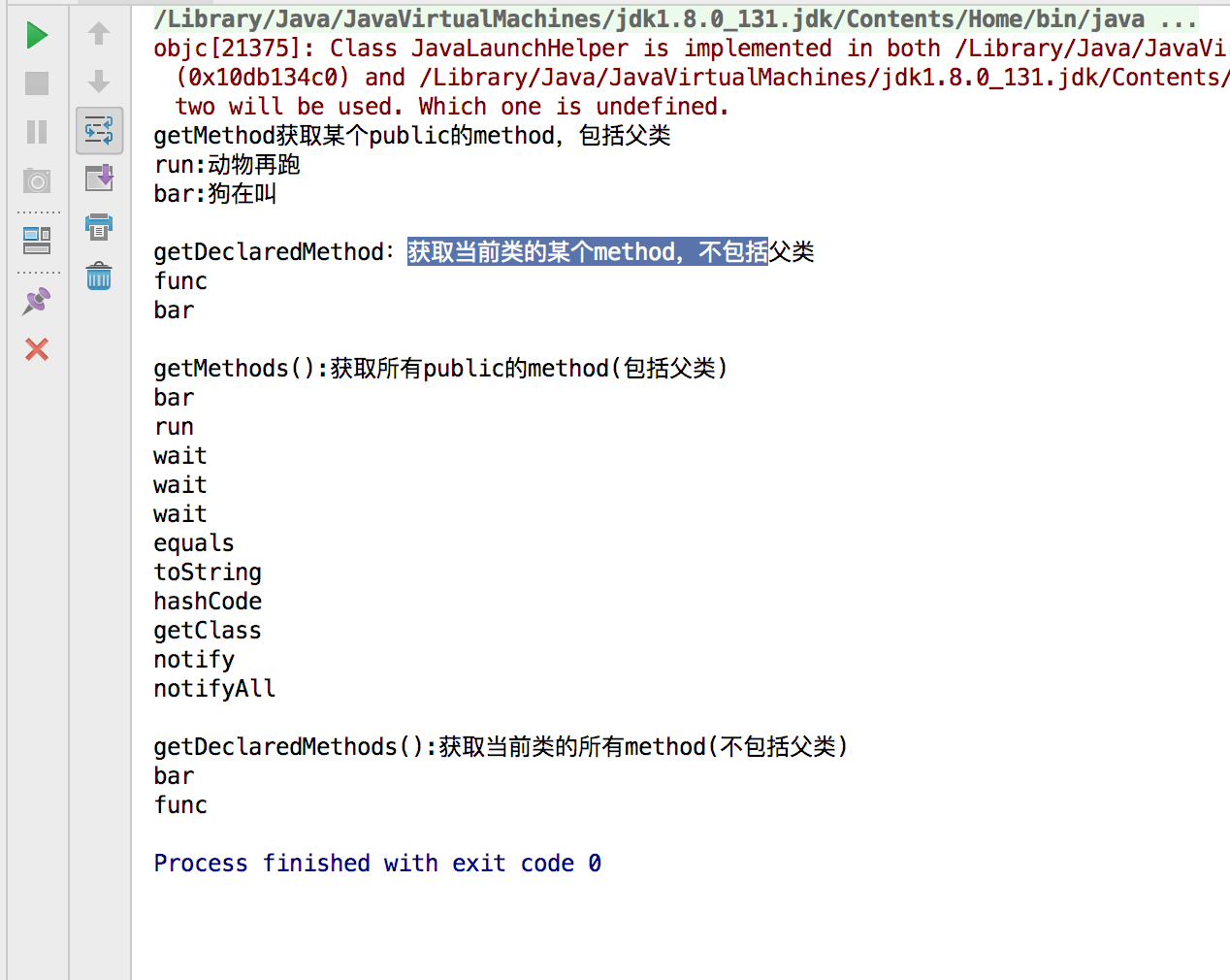
}
}
<img src="https://img2018.cnblogs.com/blog/1418970/201902/1418970-20190219195137187-650653198.png" width="500" />
## 3.3调用Method:
* Object invoke(Object obj,Object...args)
通过设置setAccessible(true)来访问非public方法
反射调用Method也遵守多态的规则
<font color=#9400D3>参见3.1中代码</font>
参考博客:
[https://blog.csdn.net/z69183787/article/details/23852517](https://blog.csdn.net/z69183787/article/details/23852517)
[https://blog.csdn.net/GhostFromHeaven/article/details/6498350](https://blog.csdn.net/GhostFromHeaven/article/details/6498350)