工程目录
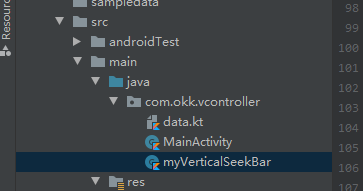
垂直SeekBar实现代码
package com.okk.vcontroller
import android.content.Context
import android.graphics.Canvas
import android.graphics.Rect
import android.graphics.drawable.Drawable
import android.util.AttributeSet
import android.view.MotionEvent
class myVerticalSeekBar : androidx.appcompat.widget.AppCompatSeekBar{
private var mThumb: Drawable? = null
private var mOnSeekBarChangeListener: OnSeekBarChangeListener? = null
constructor(context: Context):super(context)
constructor(context: Context, attrs: AttributeSet):super(context, attrs)
constructor(context: Context, attrs: AttributeSet, style: Int):super(context, attrs, style)
override fun setOnSeekBarChangeListener(l: OnSeekBarChangeListener?) {
mOnSeekBarChangeListener = l
}
override fun onSizeChanged(w: Int, h: Int, oldw: Int, oldh: Int) {
super.onSizeChanged(h, w, oldh, oldw)
}
override fun onMeasure(widthMeasureSpec: Int, heightMeasureSpec: Int) {
super.onMeasure(heightMeasureSpec, widthMeasureSpec)
setMeasuredDimension(measuredHeight, measuredWidth)
}
override fun onDraw(c: Canvas) {
c.rotate(-90f)
c.translate(-height.toFloat(), 0f)
super.onDraw(c)
}
fun onProgressRefresh(scale: Float, fromUser: Boolean) {
val thumb = mThumb
if (thumb != null) {
setThumbPos(height, thumb, scale, Int.MIN_VALUE)
invalidate()
}
if (mOnSeekBarChangeListener != null) {
mOnSeekBarChangeListener!!.onProgressChanged(this, progress, fromUser)
}
}
private fun setThumbPos(w: Int, thumb: Drawable, scale: Float, gap: Int) {
val available = w - paddingLeft - paddingRight
val thumbWidth = thumb.intrinsicWidth
val thumbHeight = thumb.intrinsicHeight
val thumbPos = (scale * available + 0.5f).toInt()
// int topBound = getWidth() / 2 - thumbHeight / 2 - getPaddingTop();
// int bottomBound = getWidth() / 2 + thumbHeight / 2 - getPaddingTop();
val topBound: Int
val bottomBound: Int
if (gap == Int.MIN_VALUE) {
val oldBounds: Rect = thumb.bounds
topBound = oldBounds.top
bottomBound = oldBounds.bottom
} else {
topBound = gap
bottomBound = gap + thumbHeight
}
thumb.setBounds(thumbPos, topBound, thumbPos + thumbWidth, bottomBound)
}
override fun setThumb(thumb: Drawable?) {
mThumb = thumb
super.setThumb(thumb)
}
// fun onStartTrackingTouch() {
// if (mOnSeekBarChangeListener != null) {
// mOnSeekBarChangeListener!!.onStartTrackingTouch(this)
// }
// }
//
// fun onStopTrackingTouch() {
// if (mOnSeekBarChangeListener != null) {
// mOnSeekBarChangeListener!!.onStopTrackingTouch(this)
// }
// }
//
// private fun attemptClaimDrag() {
// if (parent != null) {
// parent.requestDisallowInterceptTouchEvent(true)
// }
// }
override fun onTouchEvent(event: MotionEvent): Boolean {
if (!isEnabled) {
return false
}
// when (event.action) {
// MotionEvent.ACTION_DOWN -> {
// isPressed = true
// onStartTrackingTouch()
// }
// MotionEvent.ACTION_MOVE -> {
// attemptClaimDrag()
// progress = max - (max * event.y / height).toInt()
// }
// MotionEvent.ACTION_UP -> {
// onStopTrackingTouch()
// isPressed = false
// }
// MotionEvent.ACTION_CANCEL -> {
// onStopTrackingTouch()
// isPressed = false
// }
// }
when (event.action) {
MotionEvent.ACTION_DOWN, MotionEvent.ACTION_MOVE, MotionEvent.ACTION_UP -> {
//progress = max - (max * event.y / height).toInt()
//将浮点数 强制类型转换为整数 是只取整数部分
//正数+0.5,负数-0.5,然后取整即可得到四舍五入
//实现滑条拖动半拉可以选择
progress = max - ((max * event.y / height)+0.5).toInt()
onSizeChanged(width, height, 0, 0)
}
MotionEvent.ACTION_CANCEL -> {
}
}
return true
}
}
引用方式
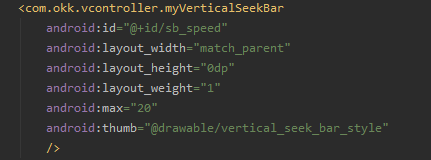
<com.okk.vcontroller.myVerticalSeekBar
android:id="@+id/sb_speed"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:max="20"
android:thumb="@drawable/vertical_seek_bar_style"
/>