概念的东西就不多写 直接上个实例
数据库sql05
数据库sql05
create database test
go
use test
go
create table Animals(
AnimalID int primary key,
AnimalType varchar(50)
)
go
go
use test
go
create table Animals(
AnimalID int primary key,
AnimalType varchar(50)
)
go
实体层Model


using System;
using System.Collections.Generic;
using System.Text;
namespace Model
{
public class Animals
{
public int _Animalsid;
public string _Animalsname;
public int AnimalID
{
set { this._Animalsid = value; }
get { return this._Animalsid; }
}
public string AnimalType
{
get { return this._Animalsname; }
set { this._Animalsname = value; }
}
}
}
实体映射文件(最讨厌的东西)Animals.hbm.xml


<?xml version="1.0" encoding="utf-8" ?>
<hibernate-mapping xmlns="urn:nhibernate-mapping-2.2">
<class name ="Model.Animals,Model" table="Animals" lazy="false" >
<id name="AnimalID" column="AnimalID" type="Int32">
<generator class ="native" />
</id>
<property name="AnimalType" column ="AnimalType" type="String" length="50" not-null="true"/>
</class>
</hibernate-mapping>
ps:XML文件的默认生成操作为“内容”,这里需要修改为“嵌入的资源”生成
数据层DAL
在这里写一个session管理类 SessionManager


using System;
using System.Collections.Generic;
using System.Text;
using NHibernate.Cfg;
using NHibernate;
namespace DAL
{
public class SessionManger
{
static readonly object key = new object();
static Configuration config;
static ISessionFactory sessionfactory;
public static ISession GetIsession()
{
if (sessionfactory == null)
{
lock (key)
{
if (sessionfactory == null)
{
config = new Configuration().Configure();
sessionfactory = config.BuildSessionFactory();
}
}
}
return sessionfactory.OpenSession();
}
}
}


using System;
using System.Collections.Generic;
using System.Text;
using NHibernate;
namespace DAL
{
public class AnimalsDAL
{
ISession session;
public AnimalsDAL()
{
session = SessionManger.GetIsession();
}
public bool Add(Model.Animals animal)
{
bool flag = false;
ITransaction tran = session.BeginTransaction();
try
{
session.Save(animal);
tran.Commit();
flag = true;
}
catch(Exception ex) {
tran.Rollback();
throw ex;
}
finally {
session.Close();
}
return flag;
}
}
}
也没什么逻辑啦 就添加方法


using System;
using System.Collections.Generic;
using System.Text;
namespace BLL
{
public class AnimalsBLL
{
public bool Add(Model.Animals animal)
{
return new DAL.AnimalsDAL().Add(animal);
}
}
}
表现层:调用
Animals a = new Animals();
a.AnimalType = "pig";
AnimalsBLL animalbll = new AnimalsBLL();
animalbll.Add(a);
别忘记了配置文件:a.AnimalType = "pig";
AnimalsBLL animalbll = new AnimalsBLL();
animalbll.Add(a);


<?xml version="1.0" encoding="utf-8" ?>
<hibernate-configuration xmlns="urn:nhibernate-configuration-2.2" >
<session-factory name="NHibernate.Test">
<!-- properties -->
<property name="connection.provider">NHibernate.Connection.DriverConnectionProvider</property>
<property name="connection.driver_class">NHibernate.Driver.SqlClientDriver</property>
<property name="connection.connection_string">Server=localhost;initial catalog=test;Integrated Security=SSPI</property>
<property name="show_sql">true</property>
<property name="dialect">NHibernate.Dialect.MsSql2005Dialect</property>
<property name="use_outer_join">true</property>
<property name="query.substitutions">true 1, false 0, yes 'Y', no 'N'</property>
<property name="proxyfactory.factory_class">NHibernate.ByteCode.LinFu.ProxyFactoryFactory, NHibernate.ByteCode.LinFu</property>
<!-- mapping files -->
<mapping assembly="Model" />
</session-factory>
</hibernate-configuration>
调试通过
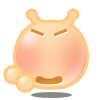