1.1 不死锁代码
#include <stdio.h>
#include <stdlib.h>
#include <memory.h>
#include <pthread.h>
#include <errno.h>
#include <math.h>
// chopstick as mutex
pthread_mutex_t chopstick[6];
void *eat_think(void *arg)
{
char phi = *(char *)arg;
int left, right;
switch(phi) {
case 'A':
left = 5;
right = 1;
break;
case 'B':
left = 1;
right = 2;
break;
case 'C':
left = 2;
right = 3;
break;
case 'D':
left = 3;
right = 4;
break;
case 'E':
left = 4;
right = 5;
break;
}
int j;
for (j = 0; j < 5; j++)
{
usleep(3);
pthread_mutex_lock(&chopstick[left]);
printf("Philosopher %c fetches chopstick %d\n", phi, left);
if (pthread_mutex_trylock(&chopstick[right]) == EBUSY)
{
pthread_mutex_unlock(&chopstick[left]);
continue;
}
// pthread_mutex_lock(&chopstick[right]);
printf("Philosopher %c fetches chopstick %d\n", phi, right);
printf("philosopher %c is eating. %d\n", phi, j+1);
usleep(3);
pthread_mutex_unlock(&chopstick[left]);
printf("philosopher %c release chopstick %d\n", phi, left);
pthread_mutex_unlock(&chopstick[right]);
printf("Philosopher %c release chopstick %d\n", phi, right);
}
}
int main()
{
pthread_t A, B, C, D, E;
int i;
for(i = 0; i < 5; i++)
{
pthread_mutex_init(&chopstick[i], NULL);
}
pthread_create(&A, NULL, eat_think, "A");
pthread_create(&B, NULL, eat_think, "B");
pthread_create(&C, NULL, eat_think, "C");
pthread_create(&D, NULL, eat_think, "D");
pthread_create(&E, NULL, eat_think, "E");
pthread_join(A, NULL);
pthread_join(B, NULL);
pthread_join(C, NULL);
pthread_join(D, NULL);
pthread_join(E, NULL);
return 0;
}
1.2 不死锁截图
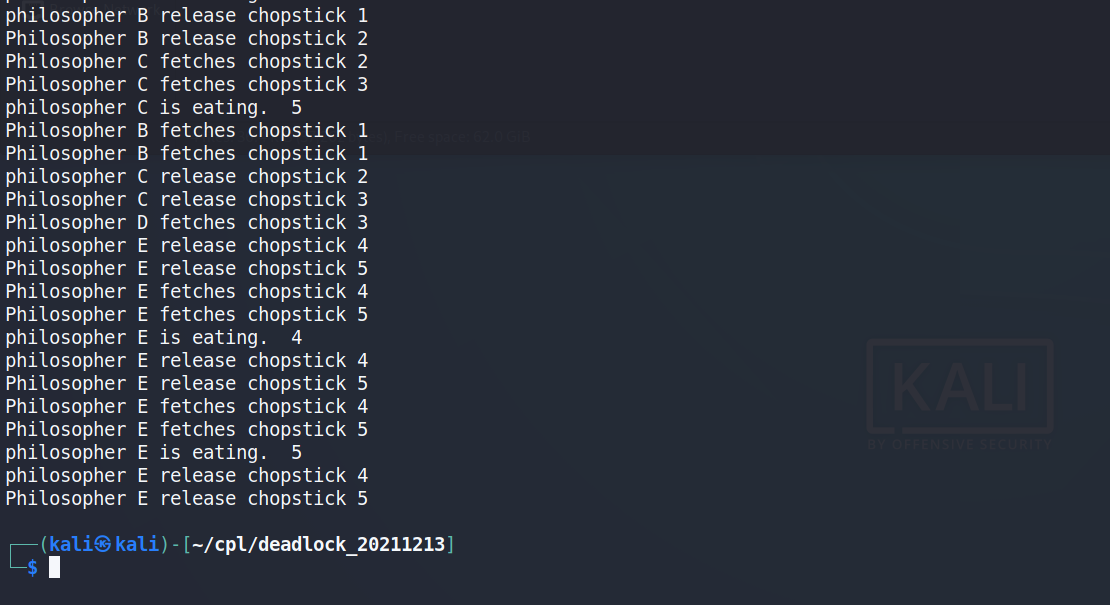
2.1 死锁代码
#include <stdio.h>
#include <stdlib.h>
#include <memory.h>
#include <pthread.h>
#include <errno.h>
#include <math.h>
// chopstick as mutex
pthread_mutex_t chopstick[6];
void *eat_think(void *arg)
{
char phi = *(char *)arg;
int left, right;
switch(phi) {
case 'A':
left = 5;
right = 1;
break;
case 'B':
left = 1;
right = 2;
break;
case 'C':
left = 2;
right = 3;
break;
case 'D':
left = 3;
right = 4;
break;
case 'E':
left = 4;
right = 5;
break;
}
int j;
for (j = 0; j < 500; j++)
{
usleep(3);
pthread_mutex_lock(&chopstick[left]);
printf("Philosopher %c fetches chopstick %d\n", phi, left);
// if (pthread_mutex_trylock(&chopstick[right] == EBUSY))
// {
// pthread_mutex_unlock(&chopstick[left]);
// continue;
// }
pthread_mutex_lock(&chopstick[right]);
printf("Philosopher %c fetches chopstick %d\n", phi, right);
printf("philosopher %c is eating. %d\n", phi, j+1);
usleep(3);
pthread_mutex_unlock(&chopstick[left]);
printf("philosopher %c release chopstick %d\n", phi, left);
pthread_mutex_unlock(&chopstick[right]);
printf("Philosopher %c release chopstick %d\n", phi, right);
}
}
int main()
{
pthread_t A, B, C, D, E;
int i;
for(i = 0; i < 5; i++)
{
pthread_mutex_init(&chopstick[i], NULL);
}
pthread_create(&A, NULL, eat_think, "A");
pthread_create(&B, NULL, eat_think, "B");
pthread_create(&C, NULL, eat_think, "C");
pthread_create(&D, NULL, eat_think, "D");
pthread_create(&E, NULL, eat_think, "E");
pthread_join(A, NULL);
pthread_join(B, NULL);
pthread_join(C, NULL);
pthread_join(D, NULL);
pthread_join(E, NULL);
return 0;
}
2.2 死锁截图
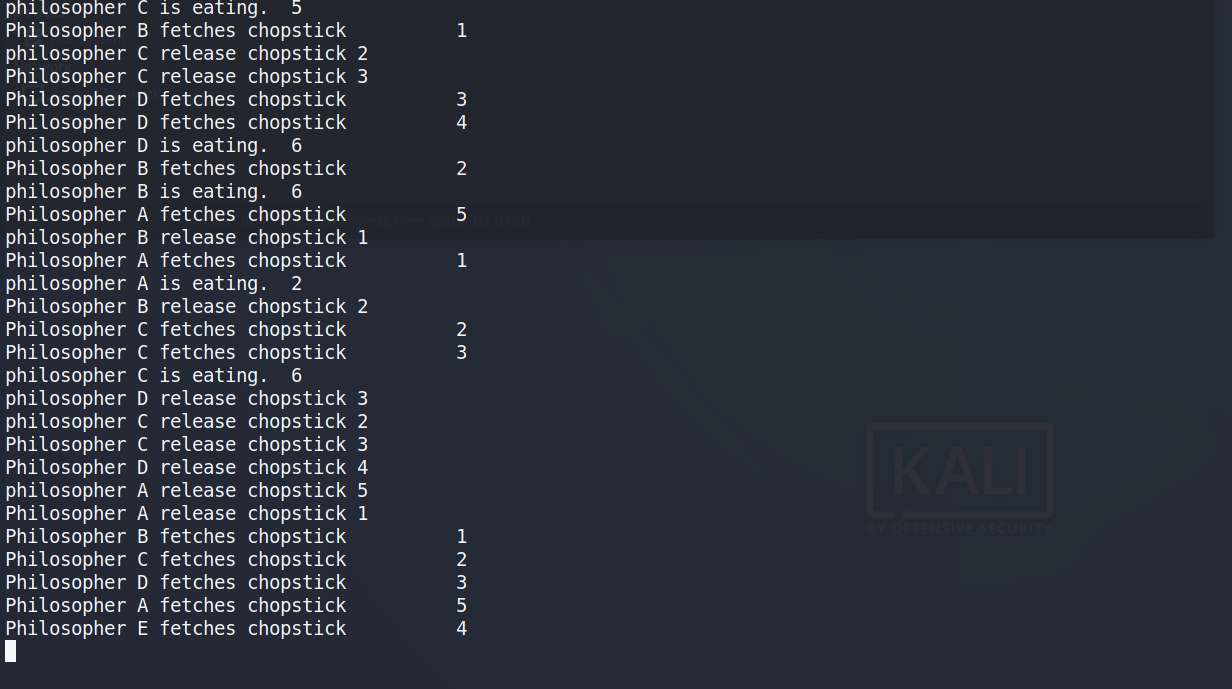