Problem Description
On the way to school, Karen became fixated on the puzzle game on her phone!
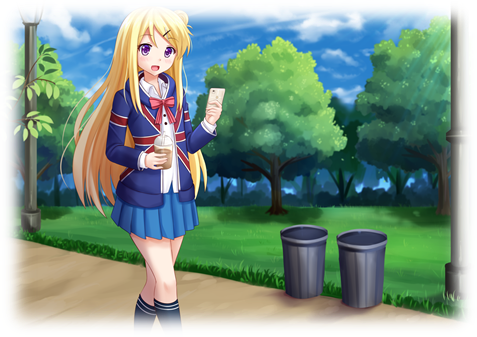
The game is played as follows. In each level, you have a grid with n rows and m columns. Each cell originally contains the number 0.
One move consists of choosing one row or column, and adding 1 to all of the cells in that row or column.
To win the level, after all the moves, the number in the cell at the i-th row and j-th column should be equal to gi, j.
Karen is stuck on one level, and wants to know a way to beat this level using the minimum number of moves. Please, help her with this task!
Input
The first line of input contains two integers, n and m (1 ≤ n, m ≤ 100), the number of rows and the number of columns in the grid, respectively.
The next n lines each contain m integers. In particular, the j-th integer in the i-th of these rows contains gi, j (0 ≤ gi, j ≤ 500).
Output
If there is an error and it is actually not possible to beat the level, output a single integer -1.
Otherwise, on the first line, output a single integer k, the minimum number of moves necessary to beat the level.
The next k lines should each contain one of the following, describing the moves in the order they must be done:
- row x, (1 ≤ x ≤ n) describing a move of the form "choose the x-th row".
- col x, (1 ≤ x ≤ m) describing a move of the form "choose the x-th column".
If there are multiple optimal solutions, output any one of them.
input
3 5
2 2 2 3 2
0 0 0 1 0
1 1 1 2 1
output
4
row 1
row 1
col 4
row 3
input
3 3
0 0 0
0 1 0
0 0 0
output
-1
input
3 3
1 1 1
1 1 1
1 1 1
output
3
row 1
row 2
row 3
In the first test case, Karen has a grid with 3 rows and 5 columns. She can perform the following 4 moves to beat the level:
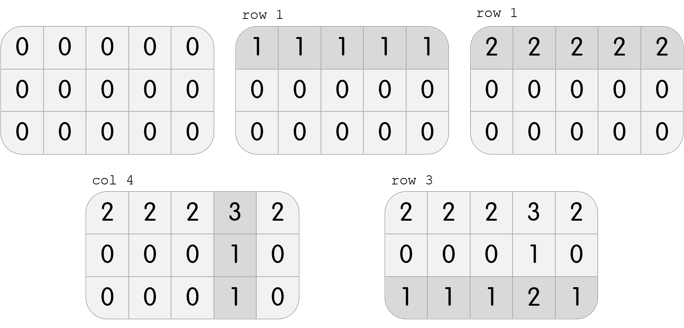
In the second test case, Karen has a grid with 3 rows and 3 columns. It is clear that it is impossible to beat the level; performing any move will create three 1s on the grid, but it is required to only have one 1 in the center.
In the third test case, Karen has a grid with 3 rows and 3 columns. She can perform the following 3 moves to beat the level:

Note that this is not the only solution; another solution, among others, is col 1, col 2, col 3.
解题思路:贪心模拟,行操作完再进行列操作。注意:题目要求用最少的步数,因此当n>m时,应先对列进行贪心减操作,再对行进行操作;否则先对行进行贪心减操作,最后如果减不完,则直接输出-1.
AC代码:
1 #include<bits/stdc++.h> 2 using namespace std; 3 const int maxn=105; 4 const int inf=500; 5 int n,m,cnt1,cnt2,ans,sum,mp[maxn][maxn],min_row[maxn],min_col[maxn]; 6 pair<int,int> paii_row[maxn],paii_col[maxn]; 7 int main(){ 8 while(cin>>n>>m){ 9 ans=sum=cnt1=cnt2=0; 10 for(int i=1;i<=n;++i)min_row[i]=inf; 11 for(int j=1;j<=m;++j)min_col[j]=inf; 12 for(int i=1;i<=n;++i){ 13 for(int j=1;j<=m;++j){ 14 cin>>mp[i][j],sum+=mp[i][j]; 15 min_row[i]=min(min_row[i],mp[i][j]); 16 min_col[j]=min(min_col[j],mp[i][j]); 17 } 18 } 19 if(n>m){///如果行大于列,则从列开始操作 20 for(int j=1;j<=m;++j){ 21 for(int i=1;i<=n;++i){ 22 mp[i][j]-=min_col[j]; 23 min_row[i]=min(min_row[i],mp[i][j]); 24 } 25 if(min_col[j])sum-=min_col[j]*n,ans+=(paii_col[cnt2].first=min_col[j]),paii_col[cnt2++].second=j,min_col[j]=0; 26 } 27 for(int i=1;i<=n;++i){///从行开始操作 28 for(int j=1;j<=m;++j){ 29 mp[i][j]-=min_row[i]; 30 min_col[j]=min(min_col[j],mp[i][j]); 31 } 32 if(min_row[i])sum-=min_row[i]*m,ans+=(paii_row[cnt1].first=min_row[i]),paii_row[cnt1++].second=i,min_row[i]=0; 33 } 34 } 35 else{ 36 for(int i=1;i<=n;++i){///否则先从行开始操作 37 for(int j=1;j<=m;++j){ 38 mp[i][j]-=min_row[i]; 39 min_col[j]=min(min_col[j],mp[i][j]); 40 } 41 if(min_row[i])sum-=min_row[i]*m,ans+=(paii_row[cnt1].first=min_row[i]),paii_row[cnt1++].second=i,min_row[i]=0; 42 } 43 for(int j=1;j<=m;++j){ 44 for(int i=1;i<=n;++i){ 45 mp[i][j]-=min_col[j]; 46 min_row[i]=min(min_row[i],mp[i][j]); 47 } 48 if(min_col[j])sum-=min_col[j]*n,ans+=(paii_col[cnt2].first=min_col[j]),paii_col[cnt2++].second=j,min_col[j]=0; 49 } 50 } 51 if(sum)cout<<-1<<endl; 52 else{ 53 cout<<ans<<endl; 54 for(int i=0;i<cnt1;++i) 55 for(int j=1;j<=paii_row[i].first;++j) 56 cout<<"row "<<paii_row[i].second<<endl; 57 for(int i=0;i<cnt2;++i) 58 for(int j=1;j<=paii_col[i].first;++j) 59 cout<<"col "<<paii_col[i].second<<endl; 60 } 61 } 62 return 0; 63 }