Problem Description:
在一个二维平面,从左到右竖立n根高度分别为:a[1],a[2],....a[n],且宽度都为1的木板。试给出平面内最大的矩阵面积。
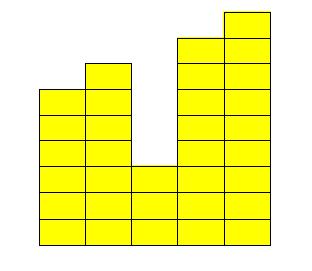
Input:
输入包含多组测试数据,每一组数据的第一行输入正整数n(<=5000),下面一行输入序列a[1],a[2],...a[n],其中1<=a[i]<=1000。
Output:
对于每一组测试数据,输出最大的矩形面积。
Sample Input:
5 6 7 3 8 9 3 2 1 4
Sample Output:
16 4
解题思路:题目的意思就是找出最大的矩形面积,思路就是每个当前高度向左向右所能伸长的最大宽度*当前高度,再跟最大值比较即可,数据比较小,水过!
AC代码一:
1 #include<bits/stdc++.h> 2 using namespace std; 3 int a[5005],n,Maxarea,len; 4 int main() 5 { 6 while(cin>>n){ 7 for(int i=0;i<n;++i)cin>>a[i]; 8 Maxarea=0; 9 for(int i=0;i<n;++i){ 10 len=1; 11 for(int j=i+1;j<n && a[i]<=a[j];++j)++len; 12 for(int j=i-1;j>=0 && a[i]<=a[j];--j)++len; 13 Maxarea=max(Maxarea,a[i]*len); 14 } 15 cout<<Maxarea<<endl; 16 } 17 return 0; 18 }
AC代码二:单调栈的运用。时间复杂度是O(n)。
1 #include<cstdio> 2 #include<iostream> 3 #include<algorithm> 4 #include<string.h> 5 #include<stack> 6 using namespace std; 7 typedef long long LL; 8 const int maxn=1e5+5; 9 int n,L[maxn],R[maxn],res,h[maxn]; 10 stack<int> st; 11 int main(){ 12 while(~scanf("%d",&n)){ 13 while(!st.empty())st.pop();memset(L,0,sizeof(L));memset(R,0,sizeof(R)); 14 for(int i=0;i<n;++i)scanf("%d",&h[i]); 15 for(int i=0;i<n;++i){ 16 while(!st.empty()&&h[st.top()]>=h[i])st.pop(); 17 L[i]=st.empty()?0:st.top()+1; 18 st.push(i); 19 } 20 while(!st.empty())st.pop();res=0; 21 for(int i=n-1;i>=0;--i){ 22 while(!st.empty()&&h[st.top()]>=h[i])st.pop(); 23 R[i]=st.empty()?n:st.top(); 24 st.push(i); 25 } 26 for(int i=0;i<n;++i) 27 res=max(res,h[i]*(R[i]-L[i])); 28 cout<<res<<endl; 29 } 30 return 0; 31 }