Prime Path
Time Limit: 1000MS | Memory Limit: 65536K | |
Total Submissions: 15325 | Accepted: 8634 |
Description
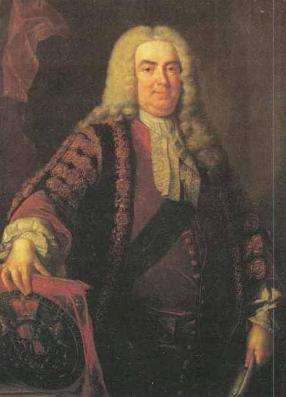
— It is a matter of security to change such things every now and then, to keep the enemy in the dark.
— But look, I have chosen my number 1033 for good reasons. I am the Prime minister, you know!
— I know, so therefore your new number 8179 is also a prime. You will just have to paste four new digits over the four old ones on your office door.
— No, it’s not that simple. Suppose that I change the first digit to an 8, then the number will read 8033 which is not a prime!
— I see, being the prime minister you cannot stand having a non-prime number on your door even for a few seconds.
— Correct! So I must invent a scheme for going from 1033 to 8179 by a path of prime numbers where only one digit is changed from one prime to the next prime.
Now, the minister of finance, who had been eavesdropping, intervened.
— No unnecessary expenditure, please! I happen to know that the price of a digit is one pound.
— Hmm, in that case I need a computer program to minimize the cost. You don't know some very cheap software gurus, do you?
— In fact, I do. You see, there is this programming contest going on... Help the prime minister to find the cheapest prime path between any two given four-digit primes! The first digit must be nonzero, of course. Here is a solution in the case above.
1033The cost of this solution is 6 pounds. Note that the digit 1 which got pasted over in step 2 can not be reused in the last step – a new 1 must be purchased.
1733
3733
3739
3779
8779
8179
Input
One line with a positive number: the number of test cases (at most 100). Then for each test case, one line with two numbers separated by a blank. Both numbers are four-digit primes (without leading zeros).
Output
One line for each case, either with a number stating the minimal cost or containing the word Impossible.
Sample Input
3 1033 8179 1373 8017 1033 1033
Sample Output
6 7 0
单纯的将所有情况都搜一遍
1 #include <iostream> 2 #include <cstring> 3 #include <algorithm> 4 #include <queue> 5 using namespace std; 6 bool prime[10000]; 7 bool vis[10000]; 8 int n[15]; 9 int a,b; 10 struct node 11 { 12 int num,t; 13 }; 14 void init() 15 { 16 int i,j; 17 memset(prime,0,sizeof(prime)); 18 for(i=1000;i<9999;i++) 19 { 20 for(j=2;j<i;j++) 21 { 22 if(i%j==0) 23 break; 24 } 25 if(j==i) 26 { 27 prime[i]=1; //1代表质数 28 //cout<<i<<endl; 29 } 30 } 31 for(i=0;i<10;i++) 32 n[i]=i; 33 n[10]=1,n[11]=3,n[12]=7,n[13]=9; 34 return; 35 } 36 int bfs() 37 { 38 int i,j; 39 queue<node> Q; 40 node tem,u; 41 int k; 42 tem.num=a,tem.t=0; 43 memset(vis,0,sizeof(vis)); 44 Q.push(tem); 45 while(!Q.empty()) 46 { 47 tem=Q.front(); 48 Q.pop(); 49 //cout<<tem.num<<endl; 50 /*if(tem.num==3733) 51 printf("adsfadsf ");*/ 52 if(tem.num==b) 53 return tem.t; 54 for(i=10;i<=13;i++) 55 { 56 if(tem.num%10==n[i]) 57 continue; 58 u.num=tem.num/10; 59 u.num=u.num*10+n[i]; 60 //cout<<u.num<<" "<<prime[u.num]<<" "<<vis[u.num]<<endl; 61 if(vis[u.num]==0&&prime[u.num]) 62 { 63 u.t=tem.t+1; 64 vis[u.num]=1; 65 Q.push(u); 66 } 67 } 68 for(i=0;i<=9;i++) 69 { 70 k=(tem.num/10)%10; 71 if(k==n[i]) 72 continue; 73 k=tem.num%10; 74 u.num=((tem.num/100)*10+n[i])*10+k; 75 if(vis[u.num]==0&&prime[u.num]) 76 { 77 u.t=tem.t+1; 78 vis[u.num]=1; 79 Q.push(u); 80 } 81 } 82 for(i=0;i<=9;i++) 83 { 84 k=(tem.num/100)%10; 85 if(k==n[i]) 86 continue; 87 k=tem.num%100; 88 u.num=((tem.num/1000)*10+n[i])*100+k; 89 if(vis[u.num]==0&&prime[u.num]) 90 { 91 u.t=tem.t+1; 92 vis[u.num]=1; 93 Q.push(u); 94 } 95 } 96 for(i=1;i<=9;i++) 97 { 98 k=tem.num/1000; 99 if(k==n[i]) 100 continue; 101 //cout<<k<<" "<<n[i]<<endl; 102 k=tem.num%1000; 103 u.num=n[i]*1000+k; 104 // cout<<u.num<<endl; 105 if(vis[u.num]==0&&prime[u.num]) 106 { 107 u.t=tem.t+1; 108 vis[u.num]=1; 109 //cout<<u.num<<endl; 110 Q.push(u); 111 } 112 } 113 //break; 114 } 115 return -1; 116 } 117 int main() 118 { 119 int T,ans; 120 init(); 121 freopen("in.txt","r",stdin); 122 //freopen("ou.txt","w",stdin); 123 scanf("%d",&T); 124 while(T--) 125 { 126 scanf("%d%d",&a,&b); 127 ans=bfs(); 128 if(ans==-1) printf("Impossible "); 129 else printf("%d ",ans); 130 } 131 }