function Dictionary() {
var items = {};
//判断是否包含Key值
this.has = function(key) {
return key in items;
};
//赋值,添加键值对
this.set = function(key, value) {
items[key] = value;
};
//移除Key值
this.remove = function(key) {
if (this.has(key)) {
delete items[key];
return true;
}
return false;
};
//通过Key值获取Value值
this.get = function(key) {
return this.has(key) ? items[key] : undefined;
};
//获取所有的Key值
this.keys = function() {
var keys = [];
for (var k in items) {
keys.push(k);
}
return keys;
};
//获取所有的Value值
this.values = function() {
var values = [];
for (var k in items) {
if (this.has(k)) {
values.push(items[k]);
}
}
return values;
};
//初始化键值对
this.clear = function() {
items = {};
};
//获取键值对的个数
this.size = function() {
var count = 0;
for (var prop in items) {
if (items.hasOwnProperty(prop)) {++count;
}
}
return count;
};
//获取所有的键值对
this.getItems = function() {
return items;
};
}
使用方法如下图:
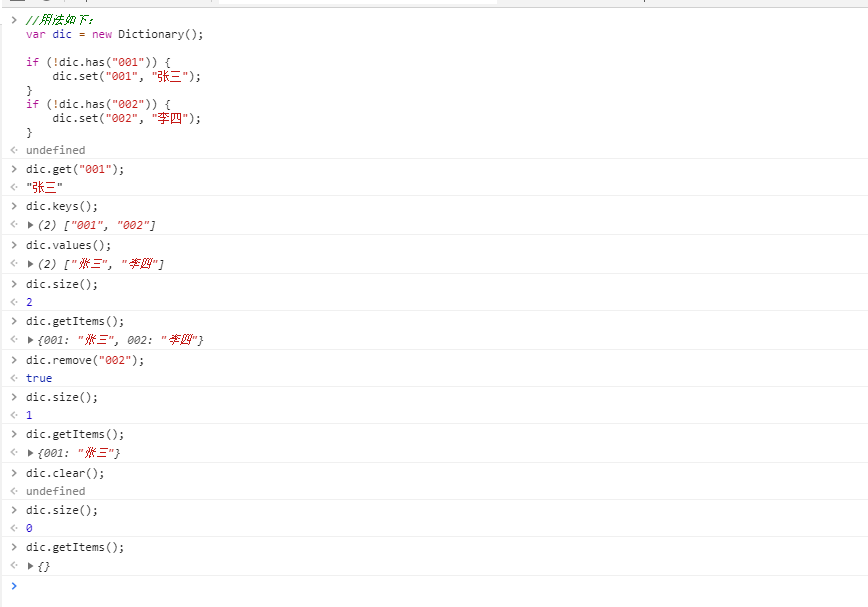