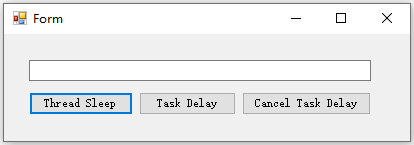
void PutThreadSleep() { Thread.Sleep(5000); } async Task PutTaskDelay() { await Task.Delay(5000); } private void btnThreadSleep_Click(object sender, EventArgs e) { PutThreadSleep(); MessageBox.Show("I am back"); } private async void btnTaskDelay_Click(object sender, EventArgs e) { await PutTaskDelay(); MessageBox.Show("I am back"); }
Basically there is nothing to describe about the code. (I am not going to explain the async and await keywords here; you can find many articles in MSDN and in my previous post explaining async/await). When I click both of these buttons, the message boxes will be displayed after 5 seconds. But there are significant differences between these two implementations. Let’s find out what's going on.
Thread.Sleep()
This is the classic way of suspending execution. This method will suspend the current thread until the given amount of time has elapsed. When you call Thread.Sleep in the above way, there is nothing you can do to abort this except waiting until the time elapses or by restarting the application. That’s because Thread.Sleep suspends the thread that's making the call. And because I'm calling Thread.Sleep in my button event handler (running in the UI thread), the UI is not responsive while it's sleeping.
Task.Delay()
Task.Delay acts in a very different way than Thread.Sleep. Basically, Task.Delay will create a task which will complete after a time delay. Task.Delay is not blocking the calling thread so the UI will remain responsive.
Behind the scenes there is a timer ticking until the specified time. Since the timer controls the delay, we can cancel the delay at any time simply by stopping the timer. To cancel, I am modifying the above PutTaskDelay method as follows:
CancellationTokenSource tokenSource = new CancellationTokenSource(); async Task PutTaskDelay() { try { await Task.Delay(5000, tokenSource.Token); } catch (TaskCanceledException ex) { } catch (Exception ex) { } }
In the call to Task.Delay I've added a cancellation token (more on that later). When the task gets cancelled, it will throw a TaskCanceledException. I am just catching the exception and suppressing it, because I don't want to show any message about that.
In my “Cancel Task Delay” button click event handler, I am using the cancellation token to signal the task to be cancelled:
private void btnCancelTaskDelay_Click(object sender, EventArgs e) { tokenSource.Cancel(); }
Once the task has been cancelled, the PutTaskDelay method returns immediately and the caller will show the message box.