Description
You are to find the length of the shortest path through a chamber containing obstructing walls. The chamber will always have sides at x = 0, x = 10, y = 0, and y = 10. The initial and final points of the path are always (0, 5) and (10, 5). There will also be from 0 to 18 vertical walls inside the chamber, each with two doorways. The figure below illustrates such a chamber and also shows the path of minimal length.
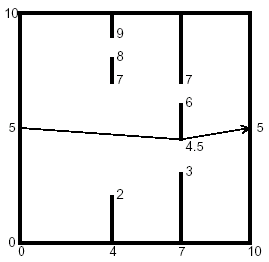
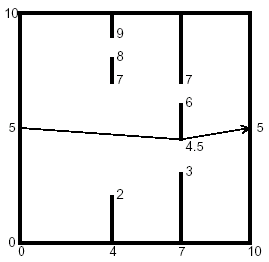
Input
The input data for the illustrated chamber would appear as follows.
2
4 2 7 8 9
7 3 4.5 6 7
The first line contains the number of interior walls. Then there is a line for each such wall, containing five real numbers. The first number is the x coordinate of the wall (0 < x < 10), and the remaining four are the y coordinates of the ends of the doorways in that wall. The x coordinates of the walls are in increasing order, and within each line the y coordinates are in increasing order. The input file will contain at least one such set of data. The end of the data comes when the number of walls is -1.
2
4 2 7 8 9
7 3 4.5 6 7
The first line contains the number of interior walls. Then there is a line for each such wall, containing five real numbers. The first number is the x coordinate of the wall (0 < x < 10), and the remaining four are the y coordinates of the ends of the doorways in that wall. The x coordinates of the walls are in increasing order, and within each line the y coordinates are in increasing order. The input file will contain at least one such set of data. The end of the data comes when the number of walls is -1.
Output
The
output should contain one line of output for each chamber. The line
should contain the minimal path length rounded to two decimal places
past the decimal point, and always showing the two decimal places past
the decimal point. The line should contain no blanks.
Sample Input
1
5 4 6 7 8
2
4 2 7 8 9
7 3 4.5 6 7
-1
Sample Output
10.00
10.06
题目大意
在一个长宽均为10,入口出口分别为(0,5)、(10,5)的房间里,有几堵墙,每堵墙上有两个缺口,求入口到出口的最短路经。
题解
计算几何+最短路。
将可行点之间建边,跑一遍最短路就好了。
用叉积判断,每条线段的两个端点是否在路径的两端,是则舍去这段路径。
1 #include<set> 2 #include<map> 3 #include<ctime> 4 #include<cmath> 5 #include<queue> 6 #include<stack> 7 #include<cstdio> 8 #include<string> 9 #include<vector> 10 #include<cstring> 11 #include<cstdlib> 12 #include<iostream> 13 #include<algorithm> 14 #define LL long long 15 #define RE register 16 #define IL inline 17 using namespace std; 18 const int N=1000; 19 20 int n; 21 double x,a,b,c,d; 22 23 struct Point 24 { 25 double x,y; 26 Point (){} 27 Point (double _x,double _y){x=_x;y=_y;} 28 Point operator - (const Point &b) 29 const{ 30 return Point(x-b.x,y-b.y); 31 } 32 double operator * (const Point &b) 33 const{ 34 return x*b.y-y*b.x; 35 } 36 }p[N+5]; 37 int top_p; 38 39 struct Segment 40 { 41 Point a,b; 42 Segment (){} 43 Segment (Point _a,Point _b) {a=_a;b=_b;} 44 }segment[N+5]; 45 int top_segment; 46 47 struct Edge 48 { 49 int to,next; 50 double cost; 51 }edge[2*N+5]; 52 int top_edge,path[N+5]; 53 54 IL bool judge(Point a,Point b); 55 IL void Add_edge(int u,int v); 56 IL double Dist(Point a,Point b); 57 IL double SPFA(); 58 59 int main() 60 { 61 while (~scanf("%d",&n)) 62 { 63 if (n==-1) break; 64 memset(path,0,sizeof(path)); 65 top_p=top_segment=top_edge=0; 66 p[top_p++]=Point(0,5); 67 for (RE int i=1;i<=n;i++) 68 { 69 scanf("%lf%lf%lf%lf%lf",&x,&a,&b,&c,&d); 70 p[top_p++]=Point(x,a); 71 for (RE int j=0;j<top_p-1;j++) if (judge(p[j],Point(x,a))) Add_edge(j,top_p-1); 72 p[top_p++]=Point(x,b); 73 for (RE int j=0;j<top_p-1;j++) if (judge(p[j],Point(x,b))) Add_edge(j,top_p-1); 74 p[top_p++]=Point(x,c); 75 for (RE int j=0;j<top_p-1;j++) if (judge(p[j],Point(x,c))) Add_edge(j,top_p-1); 76 p[top_p++]=Point(x,d); 77 for (RE int j=0;j<top_p-1;j++) if (judge(p[j],Point(x,d))) Add_edge(j,top_p-1); 78 segment[top_segment++]=Segment(Point(x,0),Point(x,a)); 79 segment[top_segment++]=Segment(Point(x,b),Point(x,c)); 80 segment[top_segment++]=Segment(Point(x,d),Point(x,10)); 81 } 82 p[top_p++]=Point(10,5); 83 for (RE int j=0;j<top_p-1;j++) if (judge(p[j],Point(10,5))) Add_edge(j,top_p-1); 84 printf("%.2lf ",SPFA()); 85 } 86 return 0; 87 } 88 89 IL void Add_edge(int u,int v) 90 { 91 edge[++top_edge].to=v; 92 edge[top_edge].next=path[u]; 93 edge[top_edge].cost=Dist(p[u],p[v]); 94 path[u]=top_edge; 95 } 96 IL double Dist(Point a,Point b){return sqrt((a.x-b.x)*(a.x-b.x)+(a.y-b.y)*(a.y-b.y));} 97 IL double SPFA() 98 { 99 queue<int>Q; 100 double dist[N+5]; 101 bool vis[N+5]={0}; 102 while (!Q.empty()) Q.pop(); 103 memset(dist,127,sizeof(dist)); 104 dist[0]=0;vis[0]=1;Q.push(0); 105 while (!Q.empty()) 106 { 107 int u=Q.front();Q.pop(); 108 for (RE int i=path[u];i;i=edge[i].next) 109 { 110 if (dist[edge[i].to]>dist[u]+edge[i].cost) 111 { 112 dist[edge[i].to]=dist[u]+edge[i].cost; 113 if (!vis[edge[i].to]) 114 { 115 Q.push(edge[i].to); 116 vis[edge[i].to]=1; 117 } 118 } 119 } 120 vis[u]=0; 121 } 122 return dist[top_p-1]; 123 } 124 IL bool judge(Point a,Point b) 125 { 126 for (RE int i=0;i<top_segment;i++) if (segment[i].a.x>a.x) 127 if ((Point(segment[i].a.x-a.x,segment[i].a.y-a.y)*Point(b.x-a.x,b.y-a.y)) 128 *(Point(segment[i].b.x-a.x,segment[i].b.y-a.y)*Point(b.x-a.x,b.y-a.y))<0) return 0; 129 return 1; 130 }