了解自定义指令的钩子函数
bind(){}:每当指令绑定到元素上的时候,就会立刻执行bind这个函数。只调用一次。
和css相关的操作,可以放在这个钩子函数中。
inserted(){}:元素插入到DOM中的时候,会执行inserted函数。只调用一次。
update(){}当数据跟新的时候,就会执行updated,可能会触发多次
可以通过 bing.value(新值) !== bing.oldValue(旧值) 来判断跟新的时候做的处理
componentUpdated(){}指令所在组件的 VNode 及其子 VNode 全部更新后调用
unbind(){}:只调用一次,指令与元素解绑时调用
所有的钩子函数的参数都有以下:
el:指令所绑定的元素,可以用来直接操作 DOM
binding:一个对象
注册成为全局指令
//main.js文件中
Vue.directive("color", {
钩子函数
})
需求描述
做一个加载动画
在我们请求接口的时候,显示加载动画。
当我们请求成功后,移除加载动画。
我们将会通过自定义指令 v-loading="isLoadFlag"来控制加载动画的开启和移除。
我们将会在页面中使用 ListCom.vue 列表组件。
加载动画组件 LoadingCom.vue。
自定义钩子 loading.js
列表组件 ListCom.vue
<template>
<div class="combox">
<div v-for="(item,index) in listArr" :key="index">
人物{{ item.name }}---- 描述 {{ item.dec}}
</div>
</div>
</template>
<script>
export default {
props: {
listArr: {
type: Array,
default: () => []
},
},
}
</script>
加载动画组件 LoadingCom.vue
<template>
<div class="loadingcssbox">
<img src="../../assets/loading.gif"/>
</div>
</template>
钩子函数 loading.js
import Vue from 'vue'
//引入加载动画组件
import LoadingCom from './LoadingCom.vue'
const loadingDirectiive = {
inserted(el, bing) {
// el ==>表示被绑定了指令的那个元素,这个el是一个原生的js对象。
// bing ==> 指令相关的信息
// 得到一个组件的构造函数
const loadingCtor = Vue.extend(LoadingCom)
// 得到实例loadingComp
const loadingComp = new loadingCtor()
// 获取实例的html
const htmlLoading = loadingComp.$mount().$el
// 将html放在el的实例上面去
el.myHtml = htmlLoading
if (bing.value) {
appendHtml(el)
}
},
update(el, bing) {
// bing.value 是v-loading绑定的那个值; true 要显示加载动画
//新值 bing.value与旧值bing.oldValue是否相等
if (bing.value !== bing.oldValue ) {
bing.value ? appendHtml(el) : removeHtml(el)
}
}
}
function appendHtml(el) {
el.appendChild(el.myHtml)
}
function removeHtml(el) {
el.removeChild(el.myHtml)
}
export default loadingDirectiive
分析上面的代码
// 得到一个组件的构造函数
const loadingCtor = Vue.extend(LoadingCom)
// 得到实例loadingComp
const loadingComp = new loadingCtor()
// 获取实例的html。将html放在el的实例上面去。
// 放在el实例上的优势是方便访问。
// $el是可以访问加载动画的html。
// 这样就可以将它添加到某一个节点上。同时也方便移除
const htmlLoading = loadingComp.$mount().$el
el.myHtml = htmlLoading
main.js 中 注册成为全局指令
import loadingDirectiive from './components/loading/loading'
Vue.directive('loading', loadingDirectiive)
<!-- 全局指令的注册方式 -->
Vue.directive("color", {
钩子函数
})
页面中使用加载动画指令 v-loading
<template>
<div class="box">
<ListCom :listArr="listArr" v-loading="isLoadFlag"></ListCom>
</div>
</template>
<script>
import ListCom from '../components/ListCom.vue'
export default {
components: {
ListCom
},
data() {
return {
listArr: [],
//通过isLoadFlag来控制动画是否开启
isLoadFlag:false,
}
},
created() {
this.sendAPi()
},
methods: {
sendAPi() {
this.isLoadFlag = true;//开启加载动画
setTimeout(() => {
this.listArr = [
{ name: '齐玄帧', dec: '五百年前的吕祖', },
{ name: '王仙芝', dec: '白帝转世' },
{ name: '李淳罡', dec: '李淳罡当初的实力是绝对的天下第一的' },
{ name: '邓太阿', dec: '徐凤年的舅舅' },
{ name: '曹长卿', dec: '这位号称独占天象八斗风流,实力排进天下前三' }
]
//移除加载动画
this.isLoadFlag = false
},3000)
}
}
}
</script>
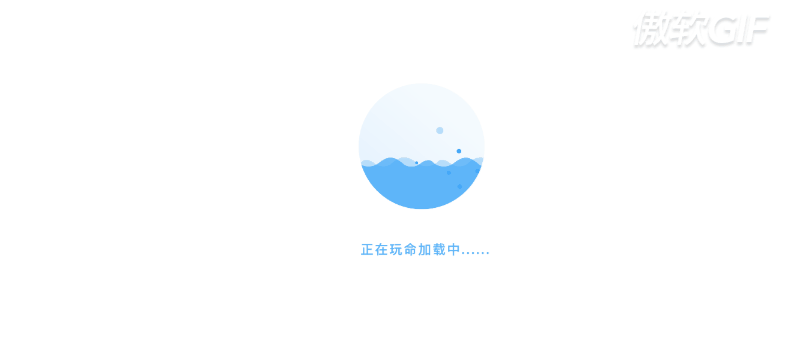
占用图指令 v-showimg
当没有数据的时候,显示一个占位图。
我们将会通过自定义指令 v-showimg="showImgFlag"来控制是否显示占位图
占位图组件 ShowImgCom.vue。
自定义钩子 showimg.js
废话不多说,直接上代码。
占位图组件 ShowImgCom.vue
<template>
<div class="showimgbox">
<img src="../../assets/nodata.png"/>
<p>暂无数据</p>
</div>
</template>
指令的书写 showimg.js
import Vue from 'vue'
import ShowImgCom from './ShowImgCom.vue'
const showimgDirectiive = {
inserted(el, bing) {
const showimgCtor = Vue.extend(ShowImgCom)
const showImgComp = new showimgCtor()
const htmlSHowPic = showImgComp.$mount().$el
el.myHtml = htmlSHowPic
if (bing.value) {
appendHtml(el)
}
},
update(el, bing) {
if (bing.value !== bing.oldValue) {
bing.value ? appendHtml(el) : removeHtml(el)
}
}
}
function appendHtml(el) {
el.appendChild(el.myHtml)
}
function removeHtml(el) {
el.removeChild(el.myHtml)
}
export default showimgDirectiive
main.js注册
import showimgDirectiive from './components/ShowImg/showimg'
Vue.directive('showimg', showimgDirectiive)
指令的使用v-showimg指令
<template>
<div class="box" v-showimg="showImgFlag">
<ListCom :listArr="listArr" v-loading="isLoadFlag"></ListCom>
</div>
</template>
<script>
import ListCom from '../components/ListCom.vue'
export default {
components: {
ListCom
},
data() {
return {
listArr: [],
isLoadFlag: false,
showImgFlag:false
}
},
created() {
this.sendAPi()
},
methods: {
sendAPi() {
this.isLoadFlag = true;//加载中
setTimeout(() => {
this.listArr = []
this.isLoadFlag = false
//是否显示占位图
if (this.listArr && this.listArr.length === 0) {
this.showImgFlag = true
} else {
this.showImgFlag =false
}
},3000)
}
}
}
</script>
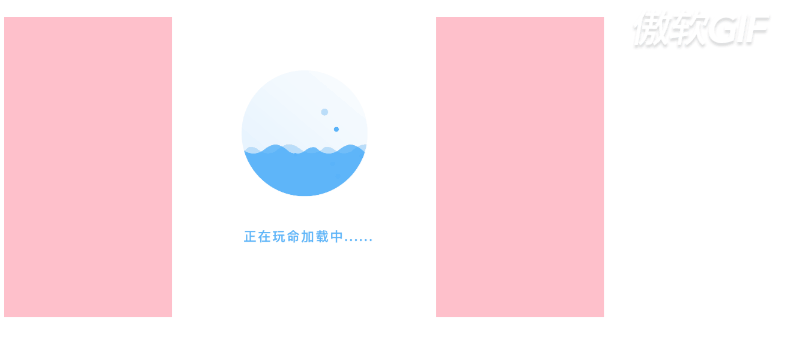