基于VSCode的c/c++编程语言的构建调试环境搭建指南
实验环境:macOS catalina 10.15.3 + vscode 1.36.1 + clang++ 11.0.0
c/c++开发环境的搭建
插件安装
为VS Code安装C ++扩展。您可以通过在“扩展”视图(⇧⌘X)中搜索“ c ++”来安装C / C ++扩展。
配置基于vscode的c/c++开发环境,一共要配置3的json文件。
tasks.json
(编译器构建设置)Launch.json
(调试器设置)c_cpp_properties.json
(编译器路径和IntelliSense设置)
创建Hello World
在macOS Terminal中,创建一个名为的空文件夹projects
,您可以在其中存储所有VS Code项目,然后创建一个名为的子文件夹helloworld.cc
,导航至该子文件夹,然后通过输入以下命令在该文件夹中打开VS Code:
mkdir projects
cd projects
mkdir helloworld
cd helloworld
code .
在当前打开的工作区中配置并添加上面的3个文件。
helloworld.cc
的代码如下
#include <iostream>
#include <vector>
#include <string>
using namespace std;
int main()
{
vector<string> msg {"Hello", "C++", "World", "from", "VS Code", "and the C++ extension!"};
for (const string& word : msg)
{
cout << word << " ";
}
cout << endl;
}
配置tasks.json
配置该文件用来告诉vscode如果通过Clang c++编译器,通过源代码编译成可执行程序。
通过command+shift+p 搜索tasks,选择第一个

//我的配置环境
{
"version": "2.0.0",
"tasks": [
{
"label": "Build with Clang",
"type": "shell",
"command": "g++",//在我的系统中g++和clang++相同
"args": [ //编译时传递给clang++的参数
"-std=c++17",
"-stdlib=libc++",
"${file}", //当前打开的文件,也就是当前选中的c++源文件,这里也可以添加多个
"-o",
"${fileBasenameNoExtension}.out", //当前打开的文件的基本名称,没有文件扩展名
"--debug"
],
"group": {
"kind": "build",
"isDefault": true
}
}
]
}
}
这里有很多配置信息,如果需要可以查看这里;
配置Launch.json
注意 macOS Catalina会出现配置了该文件,但是无法打断点的问题#3829。
配置Launch.json使我们对生成的可执行文件可以进行调试,对于上面提到的问题可以安装CodeLLDB插件之后在配置该文件解决,
先下载好CodeLLDB插件
然后command+shift+p 搜索Debug如下
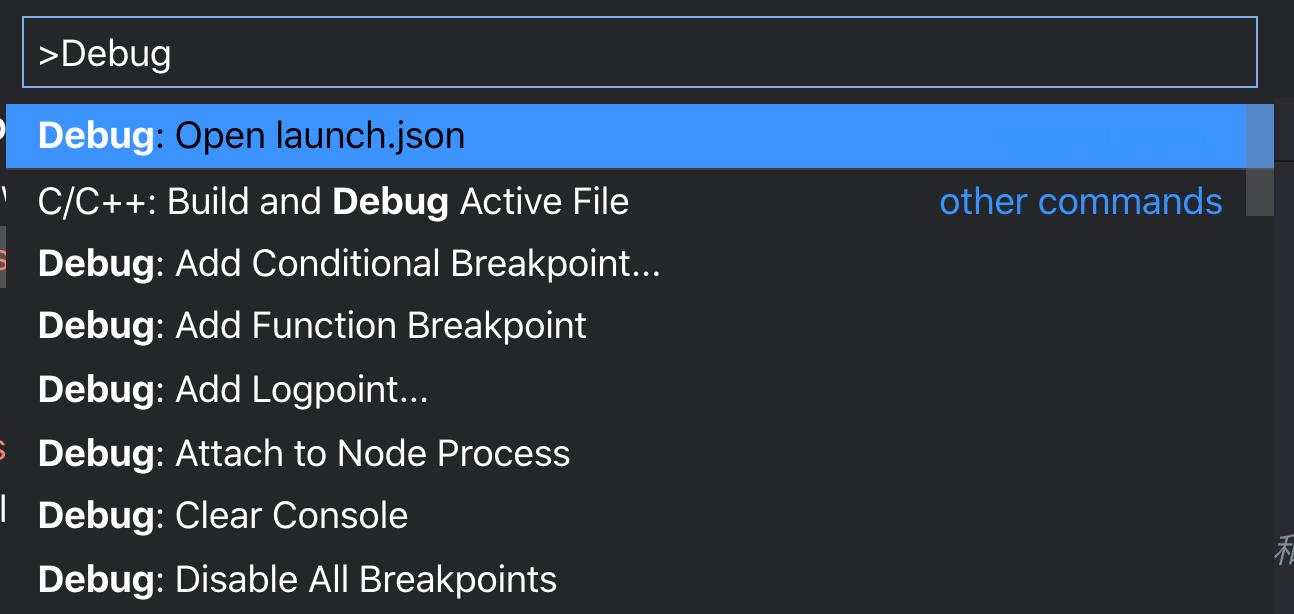
选择Debug:Open launch.json,接着选择LLDB
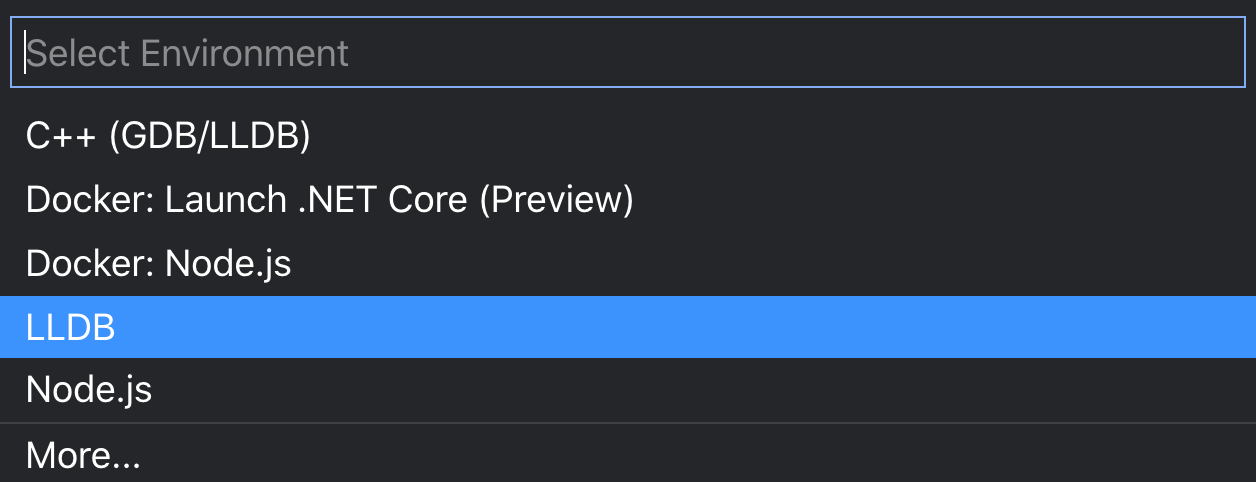
就会在.vscode
文件中看到launch.json
下面是我的配置
{
"version": "0.2.0",
"configurations": [
{
"type": "lldb", //已经安装好的Codelldb插件
"request": "launch",
"name": "Debug",
"program": "${workspaceFolder}/${fileBasenameNoExtension}.out",
"args": [],
"cwd": "${workspaceFolder}"
}
]
}
配置c_cpp_properties.json
该文件是为了更好的控制c/c++扩展,例如该文件可让您更改设置,例如编译器的路径,包含要编译的C ++标准(例如C ++ 17)的路径等。
首先command+shift+p 搜索C++如下
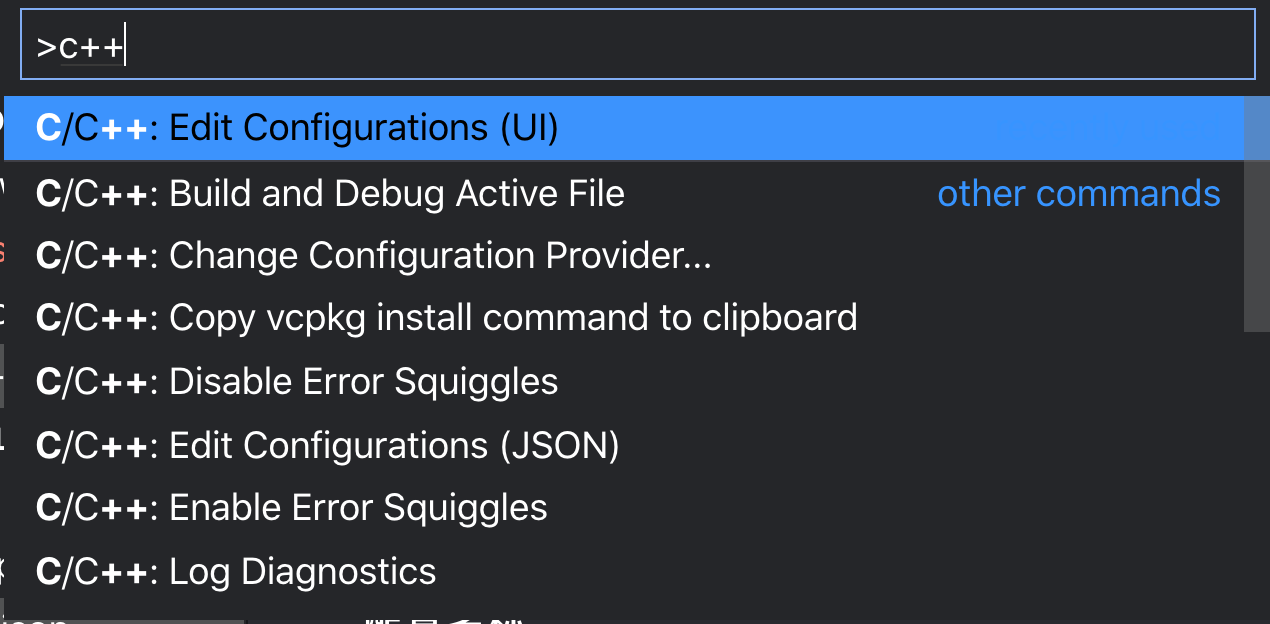
选择c/c++:Edit Configurations(UI)
就会在.vscode
文件中看到c_cpp_properties.json
下面是我的配置
{
"configurations": [
{
"name": "Mac",
"includePath": [
"${workspaceFolder}/**"
],
"defines": [],
"macFrameworkPath": [
"/Library/Developer/CommandLineTools/SDKs/MacOSX.sdk/System/Library/Frameworks"
],
"compilerPath": "/usr/bin/clang",
"cStandard": "c11",
"cppStandard": "c++17",
"intelliSenseMode": "clang-x64"
}
],
"version": 4
}
编译调试程序
通过command+shift+b 对程序helloworld.cc
进行编译,生成helloworld.out
文件
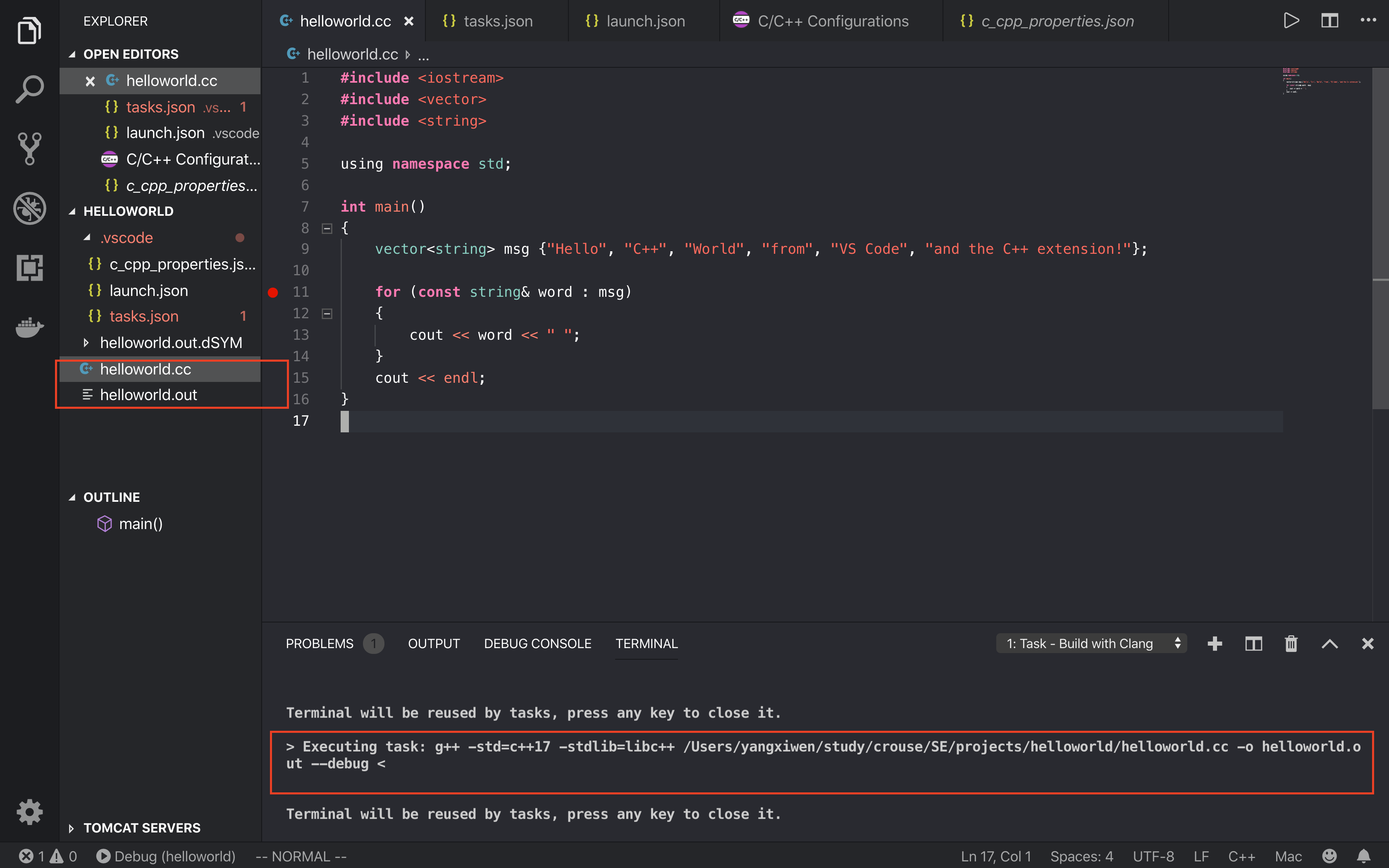
通过command+shift+d 对helloworld.out
进行调试,
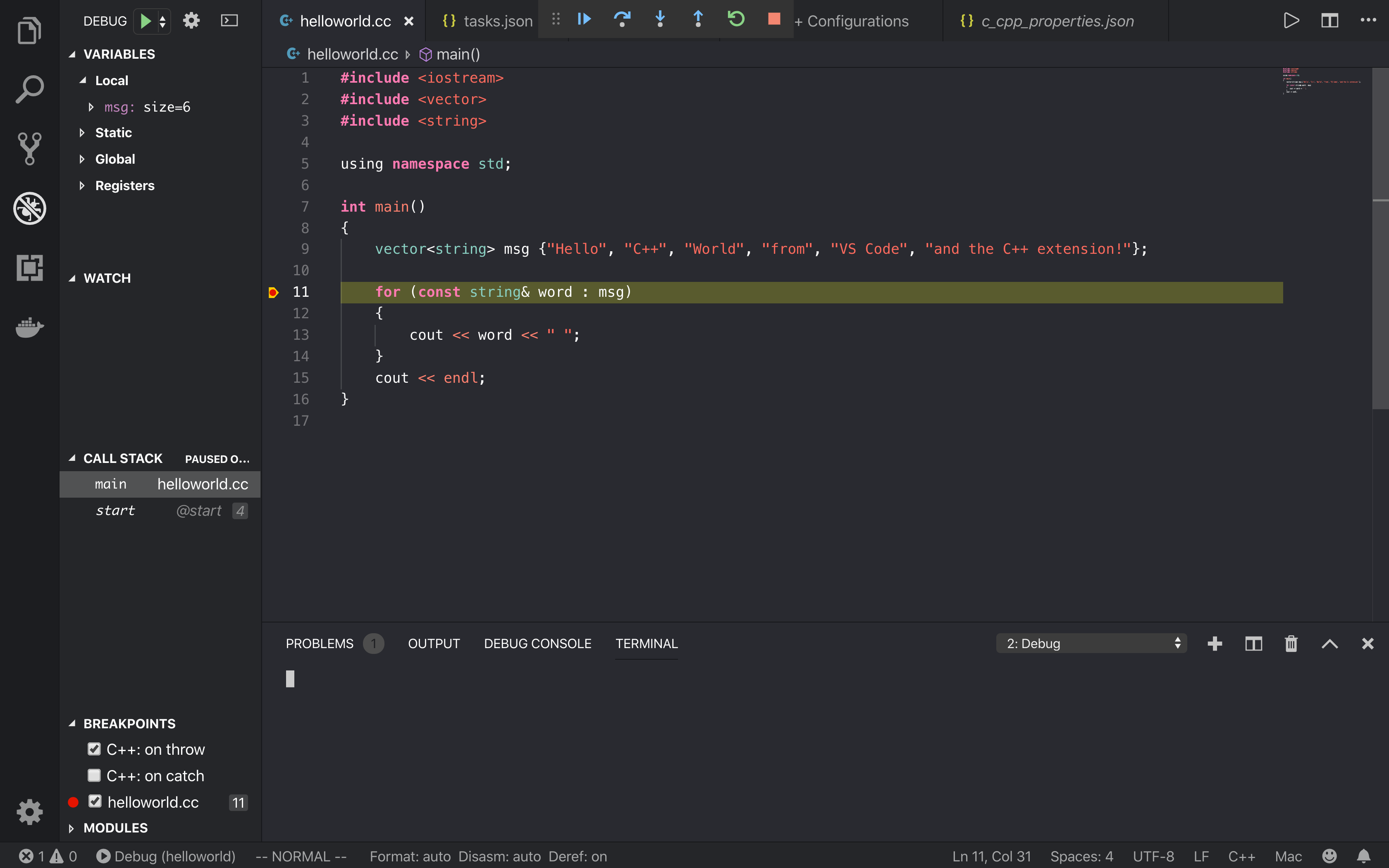
cmake重新配置tasks.json
Launch.json
通过tasks.json的args数组中设置参数可以处理过个文件编译的问题,但是不够便利,cmake是更好的工具,但是由于我也是初次使用,也算是挖个坑给自己,看了很多别人的经验但还是没有解决cmake:dubug的问题(望大佬指教)。既然现也可以通过vscode的配置来debug,就先放一放吧。
下面给出我的taske.json和launch.json的配置
//taske.json
{
"version": "2.0.0",
"options": {
"cwd": "${workspaceRoot}/build"
},
"tasks": [
{
"label": "cmake",
"type": "shell",
"command": "cmake",
"args": [".."],
"group": "build",
},
{
"label": "build",
"command": "cmake",
"args": ["--build", "."],
"group": {
"kind": "build",
"isDefault": true
},
}
]
}
//launch.json
{
// Use IntelliSense to learn about possible attributes.
// Hover to view descriptions of existing attributes.
// For more information, visit: https://go.microsoft.com/fwlink/?linkid=830387
"version": "0.2.0",
"configurations": [
{
"type": "lldb",
"request": "launch",
"name": "Debug",
// "program": "${workspaceFolder}/build/hello-vsc",
"program": "${command:cmake.launchTargetPath}",
//"program": "${workspaceFolder}/menu",
"args": [],
"cwd": "${workspaceFolder}",
}
]
}
参考文章:
https://code.visualstudio.com/docs/cpp/config-clang-mac
https://medium.com/audelabs/c-development-using-visual-studio-code-cmake-and-lldb-d0f13d38c563