题目连接
http://acm.hdu.edu.cn/showproblem.php?pid=3152
Obstacle Course
Description
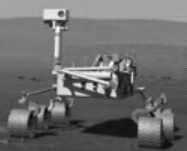
You are working on the team assisting with programming for the Mars rover. To conserve energy, the rover needs to find optimal paths across the rugged terrain to get from its starting location to its final location. The following is the first approximation for the problem.
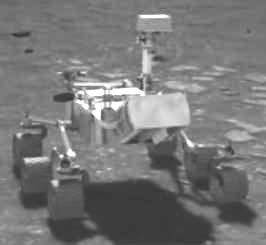
$N * N$ square matrices contain the expenses for traversing each individual cell. For each of them, your task is to find the minimum-cost traversal from the top left cell $[0][0]$ to the bottom right cell $[N-1][N-1]$. Legal moves are up, down, left, and right; that is, either the row index changes by one or the column index changes by one, but not both.
Input
Each problem is specified by a single integer between 2 and 125 giving the number of rows and columns in the $N * N$ square matrix. The file is terminated by the case $N = 0$.
Following the specification of $N$ you will find $N$ lines, each containing $N$ numbers. These numbers will be given as single digits, zero through nine, separated by single blanks.
Output
Each problem set will be numbered (beginning at one) and will generate a single line giving the problem set and the expense of the minimum-cost path from the top left to the bottom right corner, exactly as shown in the sample output (with only a single space after "Problem" and after the colon).
Sample Input
3
5 5 4
3 9 1
3 2 7
5
3 7 2 0 1
2 8 0 9 1
1 2 1 8 1
9 8 9 2 0
3 6 5 1 5
7
9 0 5 1 1 5 3
4 1 2 1 6 5 3
0 7 6 1 6 8 5
1 1 7 8 3 2 3
9 4 0 7 6 4 1
5 8 3 2 4 8 3
7 4 8 4 8 3 4
0
Sample Output
Problem 1: 20
Problem 2: 19
Problem 3: 36
bfs搜索,注意走过的点可以重复走。。

1 #include<algorithm> 2 #include<iostream> 3 #include<cstdlib> 4 #include<cstring> 5 #include<cstdio> 6 #include<vector> 7 #include<queue> 8 #include<map> 9 using std::cin; 10 using std::cout; 11 using std::endl; 12 using std::find; 13 using std::sort; 14 using std::pair; 15 using std::vector; 16 using std::multimap; 17 using std::priority_queue; 18 #define pb(e) push_back(e) 19 #define sz(c) (int)(c).size() 20 #define mp(a, b) make_pair(a, b) 21 #define all(c) (c).begin(), (c).end() 22 #define iter(c) decltype((c).begin()) 23 #define cls(arr,val) memset(arr,val,sizeof(arr)) 24 #define cpresent(c, e) (find(all(c), (e)) != (c).end()) 25 #define rep(i, n) for (int i = 0; i < (int)(n); i++) 26 #define tr(c, i) for (iter(c) i = (c).begin(); i != (c).end(); ++i) 27 const int N = 210; 28 typedef unsigned long long ull; 29 int H, G[N][N], vis[N][N]; 30 const int dx[] = { 0, 0, -1, 1 }, dy[] = { -1, 1, 0, 0 }; 31 struct Node { 32 int x, y, s; 33 Node(int i = 0, int j = 0, int k = 0) :x(i), y(j), s(k) {} 34 inline bool operator<(const Node &a) const { 35 return s > a.s; 36 } 37 }; 38 inline void read() { 39 rep(i, H) { 40 rep(j, H) { 41 scanf("%d", &G[i][j]); 42 vis[i][j] = -1; 43 } 44 } 45 } 46 int bfs() { 47 priority_queue<Node> q; 48 q.push(Node(0, 0, G[0][0])); 49 while (!q.empty()) { 50 Node t = q.top(); q.pop(); 51 if (t.x == H - 1 && t.y == H - 1) break; 52 rep(i, 4) { 53 int x = t.x + dx[i], y = t.y + dy[i]; 54 if (x < 0 || x >= H || y < 0 || y >= H) continue; 55 if (t.s + G[x][y] < vis[x][y] || vis[x][y] == -1) { 56 q.push(Node(x, y, t.s + G[x][y])); 57 vis[x][y] = t.s + G[x][y]; 58 } 59 } 60 } 61 return vis[H - 1][H - 1]; 62 } 63 int main() { 64 #ifdef LOCAL 65 freopen("in.txt", "r", stdin); 66 freopen("out.txt", "w+", stdout); 67 #endif 68 int k = 1; 69 while (~scanf("%d", &H), H) { 70 read(); 71 printf("Problem %d: %d ", k++, bfs()); 72 } 73 return 0; 74 }