Time Limit: 3000MS | Memory Limit: Unknown | 64bit IO Format: %lld & %llu |
Description
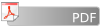
Queues and Priority Queues are data structures which are known to most computer scientists. TheTeam Queue, however, is not so well known, though it occurs often in everyday life. At lunch time the queue in front of the Mensa is a team queue, for example.
In a team queue each element belongs to a team. If an element enters the queue, it first searches the queue from head to tail to check if some of itsteammates (elements of the same team) are already in the queue. If yes, it enters the queue right behind
them. If not, it enters the queue at the tail and becomes the new last element (bad luck). Dequeuing is done like in normal queues: elements are processed from head to tail in the order they appear in the team queue.
Your task is to write a program that simulates such a team queue.
Input
The input file will contain one or more test cases. Each test case begins with the number of teamst (Finally, a list of commands follows. There are three different kinds of commands:
- ENQUEUE x - enter element x into the team queue
- DEQUEUE - process the first element and remove it from the queue
- STOP - end of test case
The input will be terminated by a value of 0 for t.
Warning: A test case may contain up to 200000 (two hundred thousand) commands, so the implementation of the team queue should be efficient: both enqueing and dequeuing of an element should only take constant time.
Output
For each test case, first print a line saying `` Scenario #k", wherek is the number of the test case. Then, for each DEQUEUE command, print the element which is dequeued on a single line. Print a blank line after each test case, even after the last one.Sample Input
2 3 101 102 103 3 201 202 203 ENQUEUE 101 ENQUEUE 201 ENQUEUE 102 ENQUEUE 202 ENQUEUE 103 ENQUEUE 203 DEQUEUE DEQUEUE DEQUEUE DEQUEUE DEQUEUE DEQUEUE STOP 2 5 259001 259002 259003 259004 259005 6 260001 260002 260003 260004 260005 260006 ENQUEUE 259001 ENQUEUE 260001 ENQUEUE 259002 ENQUEUE 259003 ENQUEUE 259004 ENQUEUE 259005 DEQUEUE DEQUEUE ENQUEUE 260002 ENQUEUE 260003 DEQUEUE DEQUEUE DEQUEUE DEQUEUE STOP 0
Sample Output
Scenario #1 101 102 103 201 202 203 Scenario #2 259001 259002 259003 259004 259005 260001
题意:先给出队伍个数以及每队成员的id,然后进行入队、出队操作,出队时输出出队的人,但是入队规则不同,若已有同队的排在前面,则直接排到最后一个同队的人的后面,即有认识的人就可以插队。因此用queue q记录团队的顺序,用queue que数组记录一支队伍的对内顺序,队伍号则用map记录。另外之前纠结修改半天纠结先判断队伍内人数是否为空还是先pop,后来发现不需要,因为你一支队伍的顺序由队员决定,只有存在队员才会有这支队伍,因此队员数>=1,因此先pop,再判断。此题还拓展了queue的数组,队列居然还有数组......醉了
代码:
#include<iostream> #include<algorithm> #include<string> #include<cmath> #include<cstdio> #include<set> #include<map> #include<queue> using namespace std; int main(void) { int t,n,i,j,cnt,T=0,idd,num; string ops; while (cin>>t&&t) { map<int,int> tlist;//队伍名记录 queue<int> q,que[1010];//队伍之间的顺序和队内人数顺序记录 T++;//计数器 idd=0; while (t--)//记录队伍以及队员所属 { cin>>n; while (n--) { cin>>num; tlist[num]=idd; } idd++; } cout<<"Scenario #"<<T<<endl;//输出当前case数 cnt=0; while (cin>>ops&&ops!="STOP") { if(ops[0]=='E')//入队 { cin>>num; i=tlist[num];//这个人属于哪支队伍? if(que[i].empty())//你前面没人即第一个到,那你来卡位 { q.push(i);//签到 } que[i].push(num);//进入对应的队伍 } else if(ops[0]=='D')//出队 { int f=q.front();队伍内第一个人 cout<<que[f].front()<<endl; que[f].pop();//出队 if(que[f].empty())//出完没人了 q.pop();//换下一队 } } cout<<endl; } return 0; }