设置网格数据源
示例应用程序启动并运行后,是时候用数据填充其视图了。
重要提示:您可以自由使用任何想要的样本数据,在此应用程序中,使用了 Expenses.sqlite3 示例数据库。该文件包含在DevExpress Demo Center的数据中,您可以从本地存储中复制它(默认位置是 C:\Users\Public\Documents\DevExpress Demos 21.2\Components\Data\Expenses.sqlite3)。将此文件包含到您的项目中,并确保应用程序配置使用所需的 SQLiteConnection 来访问它。
所有详细视图都包含以相同方式绑定的 GridControl,由 Scaffolding Wizard 生成的“EntitiesViewModel”文件包含一个可用作数据源的实体集合。以下代码说明了如何使用 SetBinding 方法将“Accounts”视图的 GridControl 绑定到其数据源。
C#
var fluent = mvvmContext1.OfType<AccountCollectionViewModel>(); fluent.SetBinding(gridView1, gView => gView.LoadingPanelVisible, x => x.IsLoading); fluent.SetBinding(gridControl1, gControl => gControl.DataSource, x => x.Entities);
VB.NET
Dim fluent = mvvmContext1.OfType(Of AccountCollectionViewModel)() fluent.SetBinding(gridView1, Function(gView) gView.LoadingPanelVisible, Function(x) x.IsLoading) fluent.SetBinding(gridControl1, Function(gControl) gControl.DataSource, Function(x) x.Entities)
对于其余的详细视图,此代码保持不变。 您只需要修改 ViewModel 的名称,传递给 OfType() 方法。
当您的视图绑定到所需数据时,可能希望将网格与 SelectedEntity 对象同步,该对象保留当前选定的实体。 为此,请使用将创建此绑定并在每次发生 ColumnView.FocusedRowObjectChanged 事件时刷新它的事件到命令操作,下面的代码说明了帐户视图的示例。
C#
fluent.WithEvent<DevExpress.XtraGrid.Views.Base.ColumnView, DevExpress.XtraGrid.Views.Base.FocusedRowObjectChangedEventArgs>(gridView1, "FocusedRowObjectChanged") .SetBinding(x => x.SelectedEntity, args => args.Row as DataModels.Account, (gView, entity) => gView.FocusedRowHandle = gView.FindRow(entity));
VB.NET
fluent.WithEvent(Of DevExpress.XtraGrid.Views.Base.ColumnView, DevExpress.XtraGrid.Views.Base.FocusedRowObjectChangedEventArgs)(gridView1, "FocusedRowObjectChanged").SetBinding(Function(x) x.SelectedEntity, Function(args) TryCast(args.Row, DataModels.Account), Sub(gView, entity) gView.FocusedRowHandle = gView.FindRow(entity))
绑定编辑表单视图
编辑表单视图包含数据布局控件替代网格控件,绑定本身的概念保持不变。 不同之处在于详细视图显示整个实体集合,因此使用自动生成的“EntitiesViewModel”类的属性和方法。编辑表单视图旨在显示单个实体,因此在这里您应该使用“SingleObjectViewModel”类的方法和属性。
C#
//Account Edit Form View var fluent = mvvmContext1.OfType<AccountViewModel>(); fluent.SetObjectDataSourceBinding( accountBindingSource, x => x.Entity, x => x.Update()); //Category Edit Form View var fluent = mvvmContext.OfType<CategoryViewModel>(); fluent.SetObjectDataSourceBinding( bindingSource, x => x.Entity, x => x.Update()); //Transaction Edit Form View var fluent = mvvmContext.OfType<TransactionViewModel>(); fluent.SetObjectDataSourceBinding( bindingSource, x => x.Entity, x => x.Update());
VB.NET
'Account Edit Form View Dim fluent = mvvmContext1.OfType(Of AccountViewModel)() fluent.SetObjectDataSourceBinding(accountBindingSource, Function(x) x.Entity, Function(x) x.Update()) 'Category Edit Form View Dim fluent = mvvmContext.OfType(Of CategoryViewModel)() fluent.SetObjectDataSourceBinding(bindingSource, Function(x) x.Entity, Function(x) x.Update()) 'Transaction Edit Form View Dim fluent = mvvmContext.OfType(Of TransactionViewModel)() fluent.SetObjectDataSourceBinding(bindingSource, Function(x) x.Entity, Function(x) x.Update())
在上面的代码中,使用了 SetObjectDataSourceBinding 方法。 此方法专门用于 Binding Source 组件,并一次实现两个有用的操作。
- 实体被视为实体对象,通常不会在其任何字段更改时发送更改通知。 例如,如果您更改现有帐户的名称,相关的‘Account’ 实体仍将被视为未更改,使用 SetObjectDataSourceBinding 方法进行绑定将导致监视这些更改并更新绑定。
- 当目标 UI 元素更改其绑定属性值时(例如,编辑器的 BaseEdit.EditValue属性在运行时被修改),绑定将被更新。Binding Source 组件将知道这些更改,这会导致调用通常在修改实体时调用的方法。 通常,此方法称为“更新”,如上面的代码示例中所示。
作为这些机制的副作用,用于设计编辑表单的 DevExpress 编辑器将开始验证最终用户输入的值(见下图)。 此验证基于数据注释属性,在第一个教程中在您的数据模型中声明。
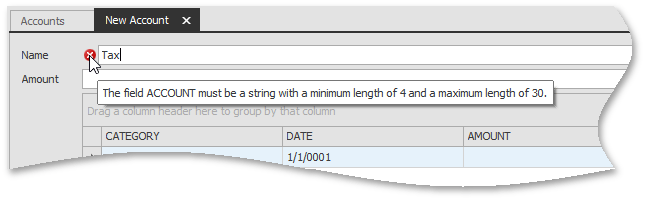
Transactions 集合的编辑表单视图具有更复杂的布局,带有下拉编辑器,允许最终用户从其他两个集合(帐户和类别)中选择实体。 因此,您将需要下拉编辑器来显示这些集合中的项目。 为此,请将您的 accountBindingSource 和 categoryBindingSource 对象绑定到所需的集合。
C#
fluent.SetBinding(accountBindingSource, abs => abs.DataSource, x => x.LookUpAccounts.Entities); fluent.SetBinding(categoryBindingSource, cbs => cbs.DataSource, x => x.LookUpCategories.Entities);
VB.NET
fluent.SetBinding(accountBindingSource, Function(abs) abs.DataSource, Function(x) x.LookUpAccounts.Entities) fluent.SetBinding(categoryBindingSource, Function(cbs) cbs.DataSource, Function(x) x.LookUpCategories.Entities)
DevExpress WinForm拥有180+组件和UI库,能为Windows Forms平台创建具有影响力的业务解决方案。DevExpress WinForms能完美构建流畅、美观且易于使用的应用程序,无论是Office风格的界面,还是分析处理大批量的业务数据,它都能轻松胜任!
DevExpress技术交流群6:600715373 欢迎一起进群讨论